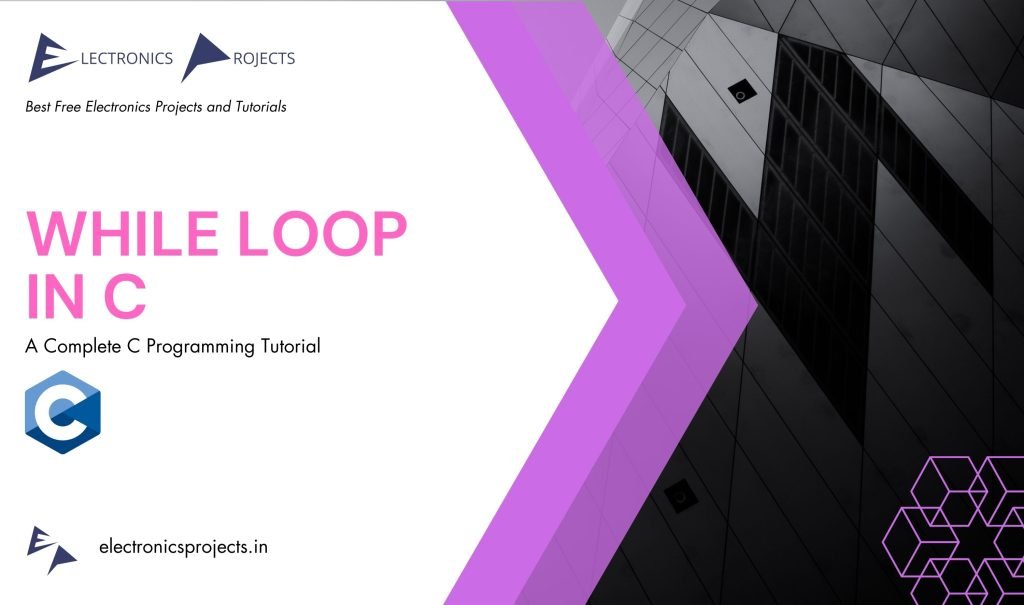
What is while loop in C?
A while loop is a control structure used in C programming language that allows the execution of a set of statements repeatedly until a specific condition is met. It is a type of loop that evaluates a condition before executing the block of code inside it.
A while loop is used when the number of iterations is not known beforehand and depends on a certain condition. It provides a flexible way to repeat a set of statements until a specific condition is satisfied.
Syntax:
while (condition)
{
// statement(s) to be executed repeatedly
}
The condition in the while loop is a boolean expression that is evaluated before executing the block of code inside the loop. If the condition is true, the statements inside the loop are executed. The loop continues to execute until the condition becomes false.
Examples of while loop:
1. Print numbers from 1 to 5
#include <stdio.h>
int main() {
int i = 1;
while (i <= 5) {
printf("%d ", i);
i++;
}
return 0;
}
Output:
1 2 3 4 5
Explanation:
In above example, a while loop is used to print the numbers from 1 to 5. The variable i
is initialized to 1, and the loop continues to execute until i
is less than or equal to 5. Inside the loop, the value of i
is printed and incremented by 1. This process continues until i
is equal to 6, and the loop terminates.
2. Sum of Natural Numbers
#include <stdio.h>
int main() {
int n, i = 1, sum = 0;
printf("Enter a positive integer: ");
scanf("%d", &n);
while (i <= n) {
sum += i;
i++;
}
printf("Sum of first %d natural numbers = %d", n, sum);
return 0;
}
Output:
Enter a positive integer: 5
Sum of first 5 natural numbers = 15
Explanation:
In above example, a while loop is used to calculate the sum of the first n
natural numbers, where n
is a positive integer entered by the user. The loop continues to execute until i
is less than or equal to n
. Inside the loop, the value of i
is added to the variable sum
, and i
is incremented by 1. This process continues until i
is equal to n+1
, and the loop terminates. Finally, the sum is printed to the console.
3. Reversing numbers
#include <stdio.h>
int main() {
int num, reversed_num = 0, remainder;
printf("Enter an integer: ");
scanf("%d", &num);
while (num != 0) {
remainder = num % 10;
reversed_num = reversed_num * 10 + remainder;
num /= 10;
}
printf("Reversed Number = %d", reversed_num);
return 0;
}
Output:
Enter an integer: 12345
Reversed Number = 54321
Explanation:
In above example, a while loop is used to reverse a given integer. Inside the loop, the remainder of the integer divided by 10 is computed, and it is added to the reversed number after being multiplied by 10. The integer is then divided by 10, and the process continues until the original number becomes 0, at which point the loop terminates. Finally, the reversed number is printed to the console.
4. Check if number is Prime or not
#include <stdio.h>
int main() {
int num, i = 2;
printf("Enter a positive integer: ");
scanf("%d", &num);
while (i <= num/2) {
if (num % i == 0) {
printf("%d is not a prime number.", num);
return 0;
}
i++;
}
printf("%d is a prime number.", num);
return 0;
}
Output:
Enter a positive integer: 17
17 is a prime number.
Explanation:
In above example, a while loop is used to check if a given number is prime or not. The loop runs from 2 to half of the given number, checking if the number is divisible by any number between 2 and half of the number. If the number is divisible by any number, it is not prime, and the loop terminates. If the loop completes without finding any divisors, the number is prime.
5. Calculate the Factorial of a number
#include <stdio.h>
int main() {
int num, i = 1, fact = 1;
printf("Enter a positive integer: ");
scanf("%d", &num);
while (i <= num) {
fact *= i;
i++;
}
printf("Factorial of %d = %d", num, fact);
return 0;
}
Output:
Enter a positive integer: 5
Factorial of 5 = 120
Explanation:
In this example, a while loop is used to calculate the factorial of a given number. The loop multiplies the variable fact
with i
and increments i
by 1 in each iteration, until i
becomes equal to the given number. The final value of fact
is the factorial of the given number.
6. Find sum of even numbers
#include <stdio.h>
int main() {
int start, end, sum = 0, i;
printf("Enter the starting and ending values of the range: ");
scanf("%d%d", &start, &end);
i = start;
while (i <= end) {
if (i % 2 == 0) {
sum += i;
}
i++;
}
printf("Sum of even numbers in the range %d to %d = %d", start, end, sum);
return 0;
}
Output:
Enter the starting and ending values of the range: 1 10
Sum of even numbers in the range 1 to 10 = 30
Explanation:
In above example, a while loop is used to find the sum of even numbers in a given range. The loop checks if the current number is even using the modulus operator, and if it is even, it adds it to the sum
variable. The loop runs from the starting value to the ending value of the range, and the final value of sum
is the sum of even numbers in the range.
Rules to be followed when using while loops in C:
- The condition in the while loop must be a boolean expression, which evaluates to either true or false.
- The loop body must be enclosed within braces
{}
. - The loop body must contain a statement that modifies the condition, otherwise the loop will run indefinitely.
- It is important to ensure that the loop will eventually terminate, otherwise it will result in an infinite loop.
- The loop body should not be empty, as an empty loop will result in a compilation error.
Modifications that can be made when using while loops in C:
- A loop can be nested within another loop to perform more complex iterations.
- The
break
statement can be used to exit the loop prematurely if a certain condition is met. - The
continue
statement can be used to skip a specific iteration and continue with the next iteration of the loop. - The condition in the while loop can be a complex expression that involves multiple variables and operators.