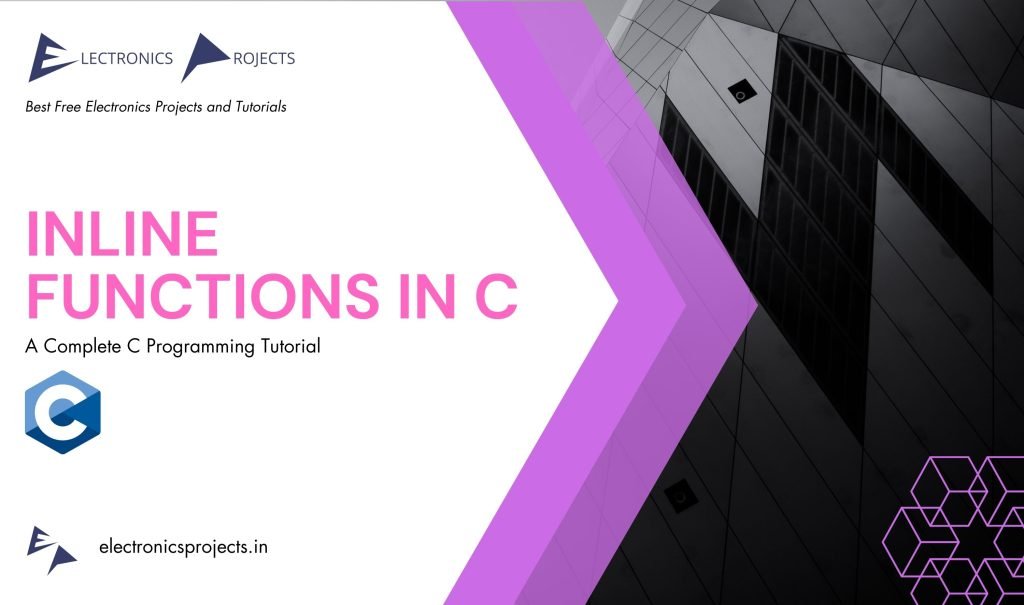
What is inline function in C?
In C, the inline
keyword is used to suggest the compiler to substitute the function call with the function code at the place where the function is called. It is used to improve the performance of a program by reducing the overhead of function calls.
An inline
function is a function that is expanded in-line when it is called, rather than calling the function through the usual function call mechanism. This means that the function’s code is directly inserted into the caller’s code, and there is no function call overhead. However, using inline
also increases the size of the program as the code for the function is duplicated at each call site.
Syntax of inline function in C:
inline return_type function_name(parameter_list)
{
// function body
}
Here, inline
is the keyword used to specify that the function is an inline function. return_type
is the data type that the function returns, function_name
is the name of the function, and parameter_list
is the list of input parameters that the function takes.
Example of inline Function
#include <stdio.h>
inline int square(int x) {
return x * x;
}
int main() {
int a = 5;
printf("Square of %d is %d\n", a, square(a));
return 0;
}
Output:
Square of 5 is 25
In above example, the square()
function is defined as inline
, which suggests the compiler to replace the function call with the function’s code at the place where it is called. In the main()
function, we call square()
with an argument of 5
. The compiler replaces the function call with 5 * 5
, which gives the result 25
. The program then prints the result using printf()
.
Rules to follow when using inline function:
inline
is a suggestion to the compiler, and the compiler may or may not inline the function.- The function should be small, preferably one-liner.
- The function should not contain loops, switches, or recursion.
- The function should not have any side effects.
- The function should be defined in the header file or in the same source file where it is used.