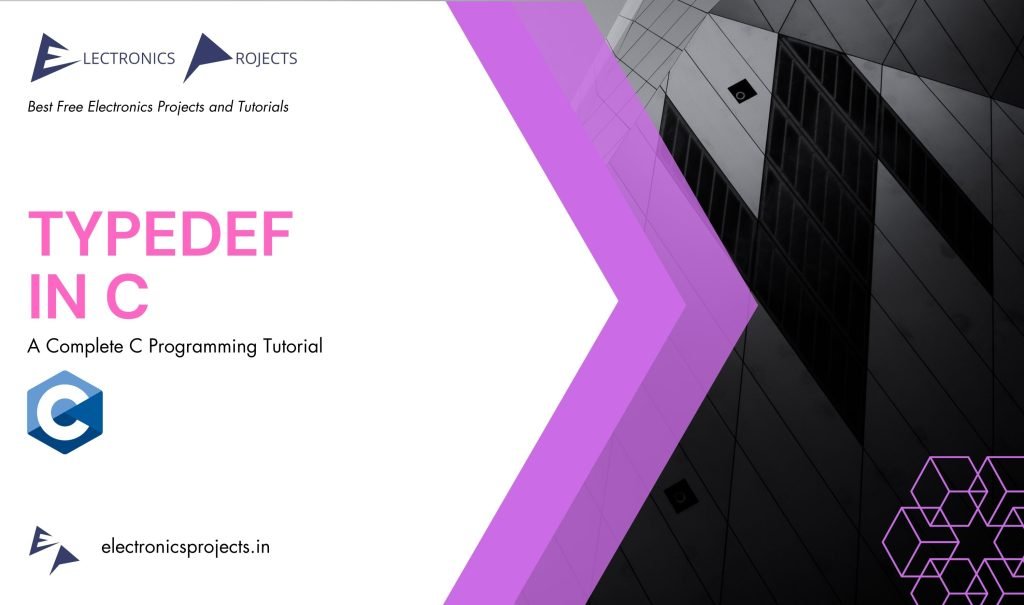
What is Typedef in C ?
In easy words, typedef
in C is like giving a nickname to a data type. It makes it easier to use and understand different data types in your program.
People use typedef
in C to make code more readable and manageable. Instead of writing long and confusing data type names, you can create shorter and more meaningful names for them.
typedef is used for:
- Creating simpler names for basic data types.
- Defining custom data types to make code more understandable.
- Creating aliases for complex structures or pointers
Syntax of typedef in C:
typedef existing_data_type new_name;
In above syntax:
existing_data_type
is the data type you want to give a nickname to.new_name
is the nickname you want to use for that data type.
Examples of typedef in C
1. Creating Simpler Names for Basic Data Types:
#include <stdio.h>
// Define a nickname for int
typedef int Number;
int main() {
Number age = 25;
printf("Age: %d\n", age);
return 0;
}
Output:
Age: 25
Explanation:
In above example, we include the necessary header file, define a nickname “Number” for the int
data type using typedef
, and then use it to declare an integer variable “age” with a value of 25. The program prints the age, demonstrating how typedef
simplifies code readability.
2. Defining Custom Data Types:
#include <stdio.h>
// Define a custom data type "Point" for 2D coordinates
typedef struct {
int x;
int y;
} Point;
int main() {
Point p;
p.x = 5;
p.y = 10;
printf("Point coordinates: (%d, %d)\n", p.x, p.y);
return 0;
}
Output:
Point coordinates: (5, 10)
Explanation:
In above example, we create a custom data type “Point” using typedef
for a structure that holds two integers representing 2D coordinates. We then declare a “Point” variable “p,” assign values to its “x” and “y” members, and print the coordinates.
3. Using Typedef for Function Pointers:
#include <stdio.h>
// Define a typedef for a function pointer
typedef void (*FunctionPtr)(int);
// Function to print a number
void printNumber(int num) {
printf("Number: %d\n", num);
}
int main() {
FunctionPtr pointer = printNumber;
pointer(42);
return 0;
}
Output:
Number: 42
Explanation:
In above complex example, we use typedef
to create a custom data type “FunctionPtr” for a pointer to a function that takes an integer argument and returns void. We then declare a “pointer” variable of this type and assign it the address of the “printNumber” function. Finally, we call the function through the pointer with the value 42, resulting in the number being printed. This demonstrates the use of typedef
for function pointers in C.