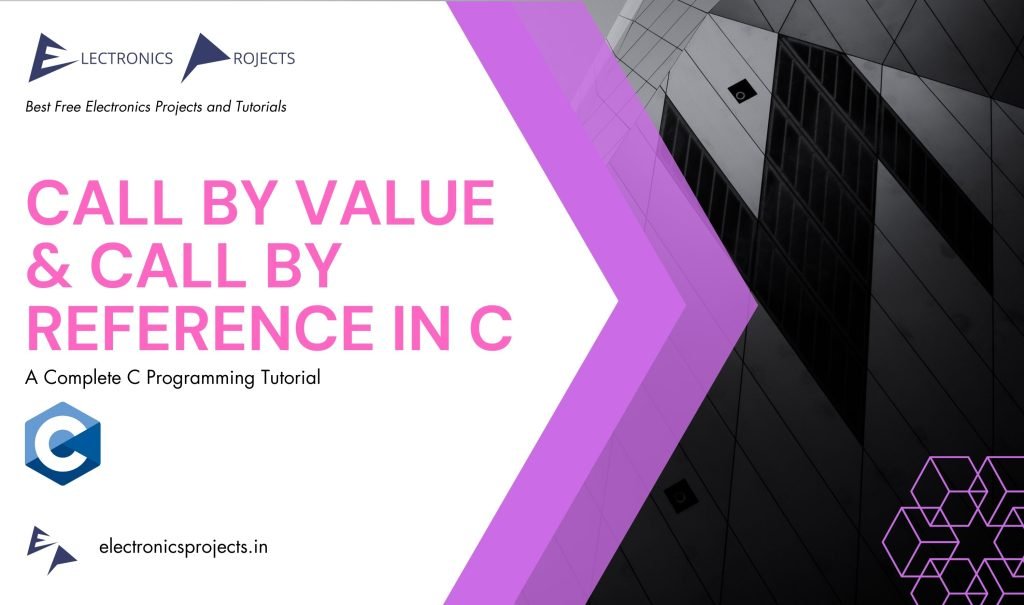
What is Call by Value in C?
In C programming, there are two ways to pass arguments to a function: call by value and call by reference.
Call by value means that the function receives a copy of the value of the variable passed as an argument. In other words, the function works with a local copy of the variable’s value and cannot modify the original value of the variable in the calling function.
Example:
#include <stdio.h>
void swap(int a, int b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int x = 10, y = 20;
swap(x, y);
printf("x = %d, y = %d", x, y); // x = 10, y = 20
return 0;
}
Output:
x = 10, y = 20
Explanation:
In the above example, the function swap
receives the values of x
and y
as arguments. However, it only works with local copies of these values and cannot modify the original values of x
and y
in the main
function.
Some rules to note:
- In the called function, the parameters are local variables that are initialized with the values of the corresponding arguments passed in the calling function.
- Any changes made to the values of the parameters within the called function do not affect the original values of the arguments in the calling function.
- Call by value is preferred when the values of the arguments passed to the function are not intended to be changed by the function.
- Complex data structures such as arrays, structures or pointers can be passed by value, but they can be inefficient for large data structures.
What is Call by Reference in C?
#include <stdio.h>
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 10, y = 20;
swap(&x, &y);
printf("x = %d, y = %d", x, y); // x = 20, y = 10
return 0;
}
Output:
x = 20, y = 10
Explanation:
In the above example, the function swap
receives pointers to x
and y
as arguments. It then dereferences these pointers to modify the original values of x
and y
in the main
function.
Some rules to note:
- In the called function, the parameters are pointers that are initialized with the memory addresses of the corresponding arguments passed in the calling function.
- Any changes made to the values of the parameters within the called function affect the original values of the arguments in the calling function.
- Call by reference is preferred when the values of the arguments passed to the function need to be changed by the function.
- The caller function needs to ensure that the address of the variable is passed to the function using the “&” operator before the variable’s name.
- The called function needs to ensure that the pointers are dereferenced using the “*” operator to access the value of the pointed-to variable.
- Care must be taken to avoid unintended side effects such as modifying the values of the parameters in the called function that can affect the operation of the calling function.
- Complex data structures such as arrays, structures, or pointers are usually passed by reference to avoid the overhead of copying large amounts of data.