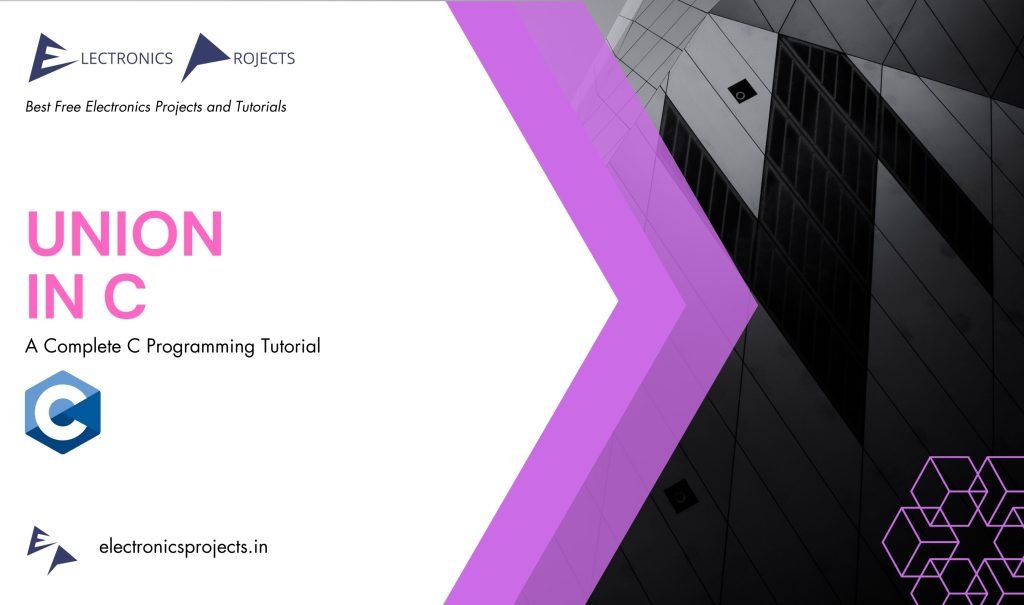
What is Union in C ?
In C programming, a union is a user-defined data type that allows different types of data to be stored in the same memory location. Unlike structures, which allocate separate memory for each member, unions allocate memory that is large enough to hold the largest member.
The main purpose of using a union in C is to save memory when multiple types of data need to be stored in the same memory location. Unions are particularly useful when the different types of data are mutually exclusive and only one of them needs to be accessed at a time.
Syntax:
union union_name {
member1;
member2;
...
};
Here, union_name
is the name of the union, and member1
, member2
, etc., are the members of the union. Each member can have a different data type.
Examples of Union in C
Example 1: Accessing Members.
#include <stdio.h>
union Person {
int age;
float height;
};
int main() {
union Person person;
person.age = 25;
printf("Age: %d\n", person.age);
person.height = 1.75;
printf("Height: %.2f\n", person.height);
return 0;
}
Output:
Age: 25
Height: 1.75
Explanation:
In above example, we define a union named Person
with members age
and height
. We assign the value 25
to the age
member and print it. Then, we assign 1.75
to the height
member and print it. Since age
and height
share the same memory location, modifying one member will affect the other.
Example 2: Assigning Values.
#include <stdio.h>
union Status {
int statusCode;
char message[20];
};
int main() {
union Status status;
status.statusCode = 200;
printf("Status Code: %d\n", status.statusCode);
strcpy(status.message, "Success");
printf("Message: %s\n", status.message);
return 0;
}
Output:
Status Code: 200
Message: Success
Explanation:
In above example, we define a union named Status
with members statusCode
and message
. We assign the value 200
to the statusCode
member and print it. Then, we use strcpy
to assign the string "Success"
to the message
member and print it.
Example 3: Bit Manipulation.
#include <stdio.h>
union BitManipulation {
int number;
struct {
unsigned int bit0 : 1;
unsigned int bit1 : 1;
unsigned int bit2 : 1;
unsigned int bit3 : 1;
unsigned int bit4 : 1;
unsigned int bit5 : 1;
unsigned int bit6 : 1;
unsigned int bit7 : 1;
} bits;
};
int main() {
union BitManipulation data;
data.number = 0;
data.bits.bit0 = 1;
data.bits.bit4 = 1;
printf("Number: %d\n", data.number);
printf("Bit 0: %u\n", data.bits.bit0);
printf("Bit 1: %u\n", data.bits.bit1);
printf("Bit 2: %u\n", data.bits.bit2);
printf("Bit 3: %u\n", data.bits.bit3);
printf("Bit 4: %u\n", data.bits.bit4);
printf("Bit 5: %u\n", data.bits.bit5);
printf("Bit 6: %u\n", data.bits.bit6);
printf("Bit 7: %u\n", data.bits.bit7);
return 0;
}
Output:
Number: 17
Bit 0: 1
Bit 1: 0
Bit 2: 0
Bit 3: 0
Bit 4: 1
Bit 5: 0
Bit 6: 0
Bit 7: 0
Explanation:
In above example, we define a union named BitManipulation
that contains an int
member number
and a structure with individual bit fields representing the bits of the number
. We start with number
initialized to 0
. Then, we set bit0
and bit4
to 1
. By modifying the individual bits, we can manipulate the value of number
.
Rules to be followed when using unions in C:
- Only one member of a union should be accessed at a time.
- Unions can only store the largest member’s size of memory.
- Changing the value of one member will affect the other members.
- Unions cannot contain members that require constructors or destructors.
- It is not safe to assume the value of a union member unless it has been explicitly assigned.
- Unions can be used for type punning, but it can lead to undefined behavior if misused.
- Unions cannot contain members that have a non-trivial assignment operator or copy constructor.