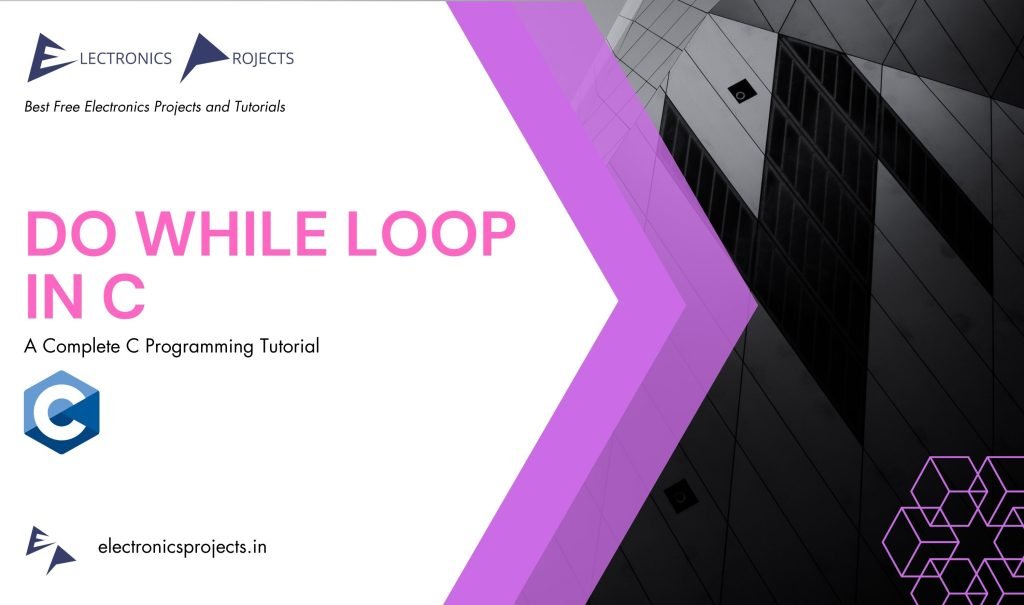
What is do while loop in C?
A “do-while” loop is a type of loop in the C programming language that executes a block of code at least once before checking the loop condition. If the condition is true, the loop body is executed again, and the process continues until the condition becomes false.
We use a do-while loop when we need to execute the loop body at least once, regardless of the condition. For example, if we want to prompt a user to enter a value until they enter a valid value, we can use a do-while loop.
Syntax:
do {
// code block to be executed
} while (condition);
Examples of do while loop in C:
1. Printing Numbers
#include <stdio.h>
int main() {
int i = 1;
do {
printf("%d ", i);
i++;
} while (i <= 5);
return 0;
}
Output:
1 2 3 4 5
Explanation:
In above example, the loop body prints the value of the variable i and increments it by 1 in each iteration. The loop continues until the value of i becomes greater than 5.
2. User Input between 1 and 10
#include <stdio.h>
int main() {
int num;
do {
printf("Enter a number between 1 and 10: ");
scanf("%d", &num);
} while (num < 1 || num > 10);
printf("You entered %d.", num);
return 0;
}
Output:
Enter a number between 1 and 10: 0
Enter a number between 1 and 10: 11
Enter a number between 1 and 10: 5
You entered 5.
Explanation:
In above example, the loop prompts the user to enter a number between 1 and 10 and reads the input using scanf(). The loop continues until the user enters a valid number within the range.
3. Printing Multiplication based on User Input
#include <stdio.h>
int main() {
int num, i = 1;
printf("Enter a number: ");
scanf("%d", &num);
do {
printf("%d x %d = %d\n", num, i, num * i);
i++;
} while (i <= 10);
return 0;
}
Output:
Enter a number: 5
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
Explanation:
In above example, the program uses a do-while loop to print the multiplication table of a number entered by the user. The loop iterates from 1 to 10, multiplies the number with the loop variable, and prints the result.
4. Printing Fibonacci Series
#include <stdio.h>
int main() {
int limit, a = 0, b = 1, c;
printf("Enter a limit: ");
scanf("%d", &limit);
printf("%d %d ", a, b);
do {
c = a + b;
printf("%d ", c);
a = b;
b = c;
} while (c <= limit);
return 0;
}
Output:
Enter a limit: 50
0 1 1 2 3 5 8 13 21 34 55
Explanation:
In above example, the program uses a do-while loop to print the Fibonacci series up to a certain limit entered by the user. The loop calculates the next term in the series by adding the previous two terms and prints the result.
5. Password Validation
#include <stdio.h>
#include <ctype.h>
int main() {
char password[50];
int has_upper = 0, has_lower = 0, has_digit = 0, i = 0;
do {
printf("Enter a password which has\n1. Lower case letter\n2. Upper case letter\n3. Number\n4. 8 or above characters\n");
printf("->");
scanf("%s", password);
for (i = 0; password[i] != '\0'; i++) {
if (isupper(password[i]))
has_upper = 1;
else if (islower(password[i]))
has_lower = 1;
else if (isdigit(password[i]))
has_digit = 1;
}
} while (i < 8 || !has_upper || !has_lower || !has_digit);
printf("Password is valid.\n");
return 0;
}
Output:
Enter a password which has
1. Lower case letter
2. Upper case letter
3. Number
4. 8 or above characters
->bruh69
Enter a password which has
1. Lower case letter
2. Upper case letter
3. Number
4. 8 or above characters
->Bruh69420
Password is valid.
Explanation:
In above example the program uses a do-while loop to validate a password entered by the user. The loop prompts the user to enter a password and checks if it meets the following criteria: it should have at least 8 characters, contain at least one uppercase letter, one lowercase letter, and one digit. If the password is invalid, the loop continues until the user enters a valid password.
Rules to follow when using do while loop in C:
- The loop body must be enclosed in curly braces {}.
- The condition must be placed after the loop body and separated by a semicolon.
- The loop body must contain code that can modify the condition, or the loop will run indefinitely.
- The loop will execute at least once, regardless of the condition.