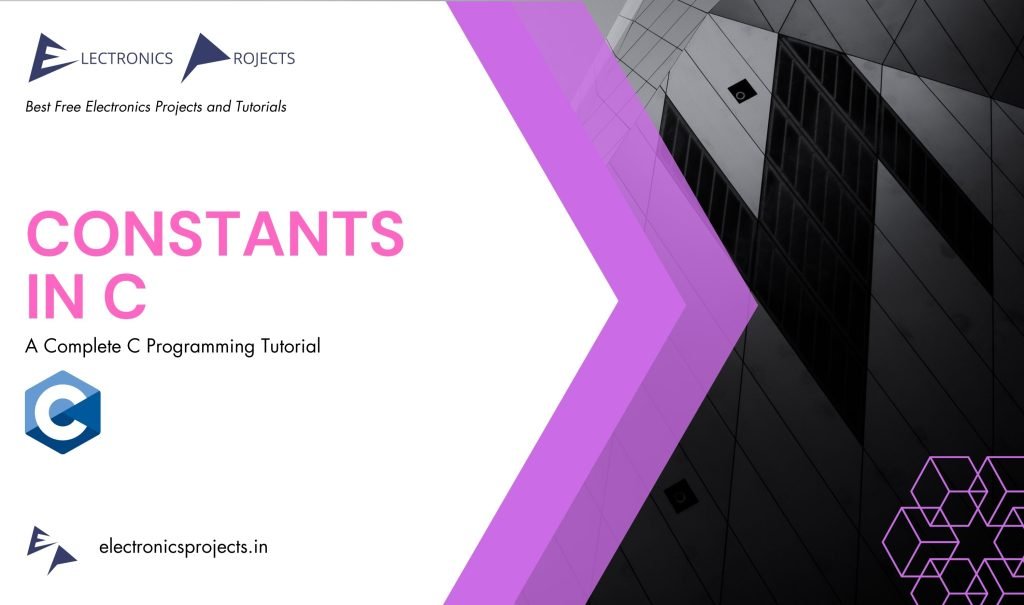
What are Constants in C?
In C, constants are values that are fixed and cannot be changed during the execution of the program. Constants are used to represent values that are known at compile time, such as the value of pi, the number of days in a week, or the maximum value of a data type.
Here’s a list of some of the most commonly used constants in C:
SR. NO. | Constants | Description | Example |
---|---|---|---|
1 | NULL | A pointer to a null object | int* ptr = NULL; |
2 | EOF | End of file marker | while ((ch = getchar()) != EOF) |
3 | TRUE | Boolean true value | if (flag == TRUE) |
4 | FALSE | Boolean false value | if (flag == FALSE) |
5 | INT_MAX | The maximum value of an ‘int’ | int i = INT_MAX; |
6 | INT_MIN | The minimum value of an ‘int’ | int i = INT_MIN; |
7 | LONG_MAX | The maximum value of an ‘long’ | long l = LONG_MAX; |
8 | LONG_MIN | The minimum value of an ‘long’ | long l = LONG_MIN; |
9 | FLT_MAX | The maximum value of an ‘float’ | float f = FLT_MAX; |
10 | FLT_MIN | The minimum value of an ‘float’ | float f = FLT_MIN; |
11 | DBL_MAX | The maximum value of an ‘double’ | double d = DBL_MAX; |
12 | DBL_MIN | The minimum value of an ‘double’ | double d = DBL_MIN; |
Different ways to define Constants in C:
1. Using #define preprocessor directive:
#define PI 3.14159265358979323846
2. Using const keyword:
const int MAX_VALUE = 100;
3. Using enum keyword:
enum boolean { FALSE, TRUE };
Example:
Here’s an example program that demonstrates the use of constants in C:
#include <stdio.h>
#define PI 3.14159265358979323846
const int MAX_VALUE = 100;
enum boolean { FALSE, TRUE };
int main() {
printf("The value of PI is: %lf\n", PI);
printf("The maximum value is: %d\n", MAX_VALUE);
printf("The value of TRUE is: %d\n", TRUE);
return 0;
}
Output:
The value of PI is: 3.141593
The maximum value is: 100
The value of TRUE is: 1
Rules to follow while using Constants in C:
- Constants cannot be modified once they are defined.
- Constants must be initialized at the time of declaration.
- Constants have a scope that is limited to the block in which they are defined.
- Constants should be named using uppercase letters to differentiate them from variables.