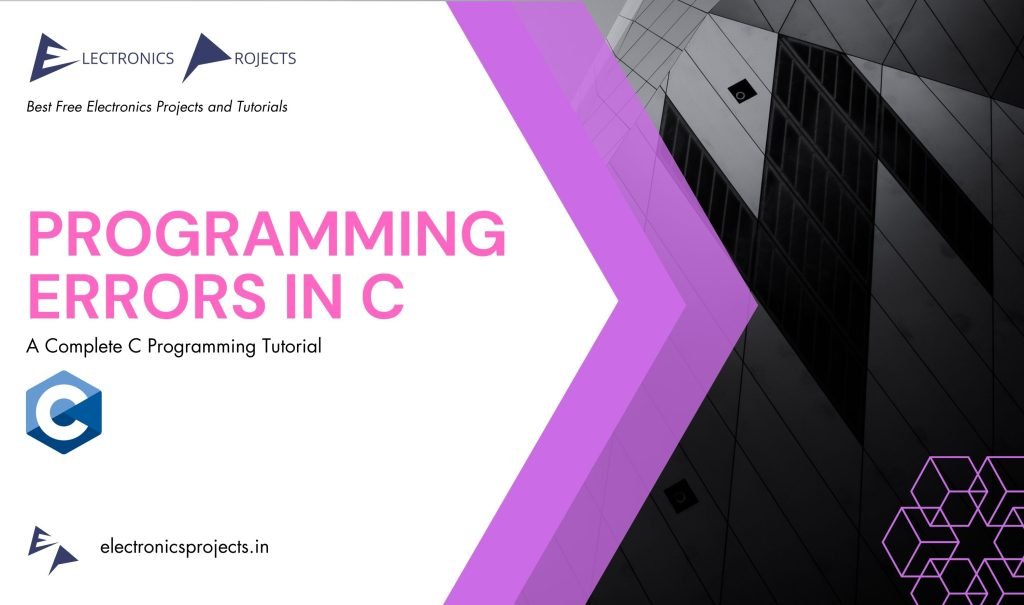
What is Programming Errors in C?
Programming errors in C are mistakes made by programmers while writing code, which prevent the program from running correctly or as intended. These errors can cause a variety of issues, ranging from minor bugs to serious security vulnerabilities.
Types of Programming Errors in C:
- Syntax Errors
- Logical Errors
- Runtime Errors
- Linker Errors
- Semantic Errors
1. Syntax Errors in C:
These errors occur when the program contains a mistake in the syntax. The compiler will usually catch these errors and prevent the program from running. Examples of syntax errors include missing semicolons, mismatched brackets, and misspelled function names.
Example:
#include <stdio.h>
int main()
{
printf("Hello, World!")
return 0;
}
Output:
prog.c: In function 'main':
prog.c:5:16: error: expected ';' before 'return'
printf("Hello, World!")
Explanation:
In the above code, a semicolon is missing after the printf
statement. The compiler detects this error and provides an error message indicating that a semicolon is expected before the return
statement.
2. Logical Errors in C:
These errors occur when the program’s logic is incorrect, resulting in unexpected or incorrect output. Examples of logical errors include incorrect conditional statements or loops that do not execute as intended.
Example:
#include <stdio.h>
int main()
{
int num1 = 5, num2 = 10, sum;
sum = num1 - num2;
printf("The sum of %d and %d is %d\n", num1, num2, sum);
return 0;
}
Output:
The sum of 5 and 10 is -5
Explanation:
In the above code, the programmer intended to calculate the sum of num1
and num2
. However, the programmer mistakenly subtracted num2
from num1
, resulting in an incorrect output.
3. Runtime Errors in C:
These errors occur during program execution and can cause crashes or unexpected behavior. Examples of runtime errors include memory leaks, division by zero, and accessing memory that is out of bounds.
Example:
#include <stdio.h>
int main()
{
int num1 = 5, num2 = 0, result;
result = num1 / num2;
printf("The result is %d\n", result);
return 0;
}
Output:
Floating point exception (core dumped)
Explanation:
In the above code, the programmer attempts to divide num1
by num2
, where num2
is 0. This results in a runtime error due to division by zero, and the program crashes.
4. Linker Errors in C:
Linker errors occur when the linker is unable to find or resolve external symbols in a program. These symbols may be defined in other source files or libraries that the program depends on. Examples of linker errors include missing function definitions, undefined references, or multiple definitions of the same symbol.
Example:
#include <stdio.h>
int main()
{
sayHello();
return 0;
}
Output:
undeined reference to `sayHello'
Explanation:
In the above code, the sayHello()
function is called, but the linker is unable to find the definition of the sayHello()
function, resulting in an undefined reference error.
5. Semantic Errors in C:
Semantic errors occur when the program logic is incorrect, even though the code is syntactically correct. These errors can be difficult to identify as they do not result in compile-time or runtime errors. Semantic errors can result in incorrect program output or unexpected behavior.
Example:
#include <stdio.h>
int main()
{
int num1 = 5, num2 = 10, sum;
sum = num1 + num2;
printf("The sum of %d and %d is %f\n", num1, num2, sum);
return 0;
}
Output:
The sum of 5 and 10 is -1073741776.000000
Explanation:
In the above code, the printf()
statement is incorrect as it expects a float value, but the sum
variable is an integer. This results in incorrect program output.
Rules and Advice to Reduce Programming Errors in C:
- Always use a consistent coding style to make the code easier to read and maintain.
- Use tools such as static analyzers and compilers with error/warning flags to identify and fix syntax, linker, and semantic errors.
- Modularize the program by breaking it down into smaller functions to reduce the complexity of the code.
- Use version control systems to keep track of code changes and revert to previous versions if necessary.
- Follow industry-standard coding practices and conventions, such as the C99 and C11 standards, to ensure code portability and compatibility.