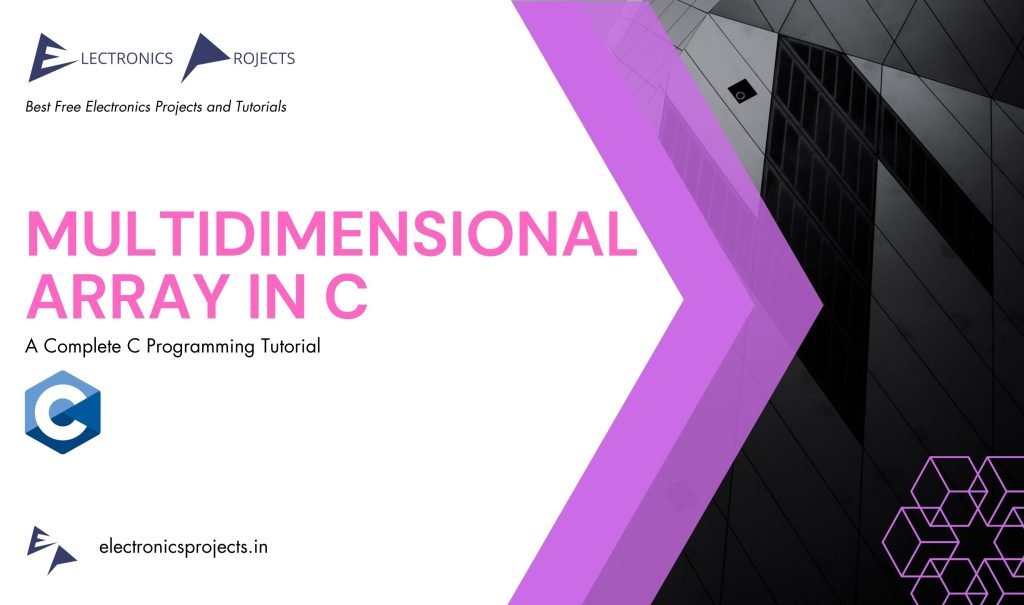
What is Multidimensional Array in C?
A multi-dimensional array in C is an array that contains more than one dimension. It is a way to organize data in a tabular form with rows and columns, or in a higher-dimensional structure. The elements in a multi-dimensional array are accessed using multiple indices.
Multi-dimensional arrays are used to represent data that can be naturally organized in multiple dimensions. They are particularly useful when dealing with matrices, tables, grids, or any data that can be conceptually represented in multiple rows and columns.
Syntax:
data_type array_name[size1][size2]...[sizeN];
Here, data_type
represents the type of elements in the array (e.g., int
, char
, etc.), array_name
is the name of the array, and size1
, size2
, …, sizeN
are the sizes of each dimension.
Examples of Multidimensional Array:
1. Two Dimensional Array representing a Matrix:
#include <stdio.h>
int main() {
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
printf("Element at matrix[1][2]: %d\n", matrix[1][2]);
return 0;
}
Output:
Element at matrix[1][2]: 6
Explanation:
In above example, we declare a two-dimensional integer array called matrix
with dimensions 3×3. We initialize the array with values 1 to 9. The statement matrix[1][2]
accesses the element in the second row and third column, which is 6. The output is “Element at matrix[1][2]: 6”.
2. Three Dimensional Array representing a 3D Space:
#include <stdio.h>
int main() {
int space[2][3][4] = {
{
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
},
{
{13, 14, 15, 16},
{17, 18, 19, 20},
{21, 22, 23, 24}
}
};
printf("Element at space[1][2][3]: %d\n", space[1][2][3]);
return 0;
}
Output:
Element at space[1][2][3]: 24
Explanation:
In above example, we declare a three-dimensional integer array called space
with dimensions 2x3x4. We initialize the array with values 1 to 24. The statement space[1][2][3]
accesses the element in the second “block”, third row, and fourth column, which is 24. The output is “Element at space[1][2][3]: 24”.
1. Two Dimensional Array representing a chessboard:
#include <stdio.h>
int main() {
char chessboard[8][8] = {
{'R', 'N', 'B', 'Q', 'K', 'B', 'N', 'R'},
{'P', 'P', 'P', 'P', 'P', 'P', 'P', 'P'},
{' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '},
{' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '},
{' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '},
{' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '},
{'p', 'p', 'p', 'p', 'p', 'p', 'p', 'p'},
{'r', 'n', 'b', 'q', 'k', 'b', 'n', 'r'}
};
// Displaying the chessboard
printf("Chessboard:\n");
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 8; j++) {
printf("%c ", chessboard[i][j]);
}
printf("\n");
}
// Getting user input for row and column
int row, column;
printf("\nEnter the row (1-8): ");
scanf("%d", &row);
printf("Enter the column (1-8): ");
scanf("%d", &column);
// Validating user input
if (row < 1 || row > 8 || column < 1 || column > 8) {
printf("Invalid input!\n");
return 0;
}
// Accessing the chosen element in the chessboard
char chosenElement = chessboard[row - 1][column - 1];
printf("Element at chessboard[%d][%d]: %c\n", row - 1, column - 1, chosenElement);
return 0;
}
Output:
Chessboard:
R N B Q K B N R
P P P P P P P P
p p p p p p p p
r n b q k b n r
Enter the row (1-8): 2
Enter the column (1-8): 2
Element at chessboard[1][1]: P
Explanation:
In above example, the program first displays the initial configuration of the chessboard to the user. It then prompts the user to enter the row and column numbers (1-8) for the element they want to access. The program validates the user input and displays the chosen element along with its indices.
Rules to follow when using Multidimensional Array:
- The dimensions of the array must be specified during declaration.
- Each dimension of the array must have a size greater than zero.
- The number of elements in the initialization list must match the total number of elements in the array.
- Array indices start from 0 and go up to size-1 in each dimension.
- The elements of a multi-dimensional array are stored in row-major order, meaning the elements of a row are stored contiguously in memory.