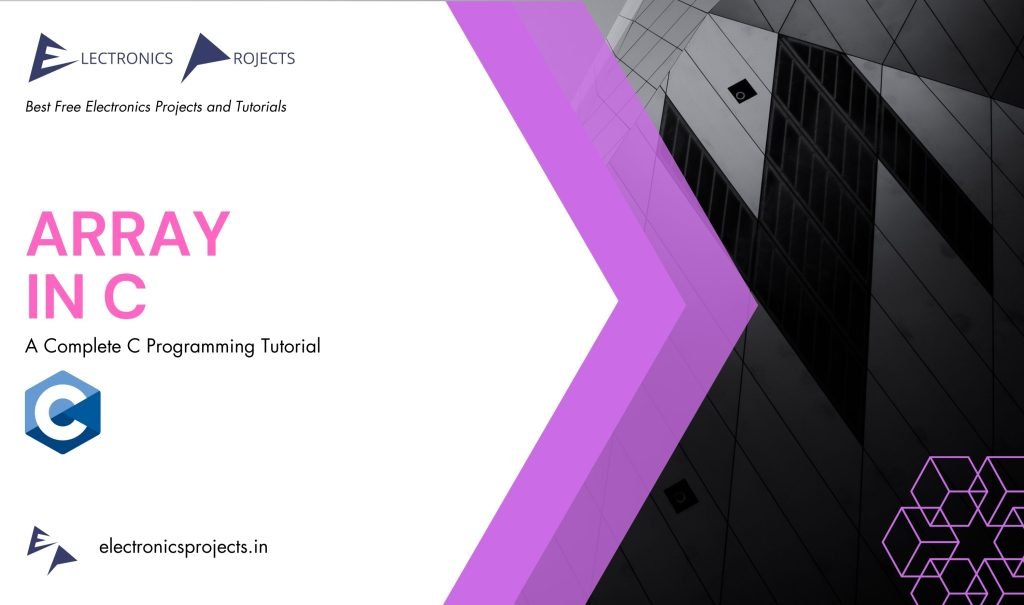
What is an Array?
An array is a collection of similar data items stored in contiguous memory locations, which are accessed using an index or a subscript. In C, arrays are used to store a fixed number of elements of the same data type.
Arrays are used to group related data items and make it easy to access and manipulate them. They are also used to implement algorithms such as searching, sorting, and matrix operations.
Syntax of an Array in C:
data_type array_name[array_size];
where data_type
specifies the type of data to be stored in the array, array_name
is the name of the array, and array_size
is the number of elements in the array.
Array Initialization and Declaration Separately:
#include <stdio.h>
int main() {
int numbers[5]; // array declaration
numbers[0] = 10; // assigning values to array elements
numbers[1] = 20;
numbers[2] = 30;
numbers[3] = 40;
numbers[4] = 50;
printf("%d\n", numbers[2]); // accessing array element using index
return 0;
}
Output:
30
In above example, we have included the standard input/output header file stdio.h
. We have also defined the main function and declared its return type as int
. Inside the main function, we have declared an integer array named numbers
with 5 elements. We have assigned values to the array elements using their indices. Finally, we have accessed and printed one of the array elements using the printf()
function.
Array Declaration and Initialization Together:
#include <stdio.h>
int main() {
int numbers[5] = {10, 20, 30, 40, 50}; // array declaration and initialization
printf("%d\n", numbers[3]); // accessing array element using index
return 0;
}
Output:
40
In above example, we have also included the standard input/output header file stdio.h
. We have defined the main function and declared its return type as int
. Inside the main function, we have declared an integer array named numbers
with 5 elements and initialized it with values using the curly braces notation. Finally, we have accessed and printed one of the array elements using the printf()
function.
Examples of Array:
Easy Examples:
1. Printing Numbers for no reason
#include <stdio.h>
int main() {
int numbers[5] = {3, 1, 4, 1, 5};
printf("The numbers are: ");
int i;
for(i = 0; i < 5; i++) {
printf("%d ", numbers[i]);
}
return 0;
}
Output:
The numbers are: 3 1 4 1 5
In above example, we have declared an integer array named numbers
with a size of 5 and initialized it with the values 3, 1, 4, 1, and 5. We have then used a for loop to print the values in the array.
2. Printing Grades
#include <stdio.h>
int main() {
float grades[3];
grades[0] = 3.7;
grades[1] = 4.0;
grades[2] = 3.9;
printf("The grades are: %.1f, %.1f, and %.1f\n", grades[0], grades[1], grades[2]);
return 0;
}
Output:
The grades are: 3.7, 4.0, and 3.9
In above example, we have declared a float array named grades
with a size of 3. We have then assigned the values 3.7, 4.0, and 3.9 to the elements of the array using the array indexing notation grades[i]
. Finally, we have printed the values in the array using the printf
function and the %f
format specifier, with a precision of one decimal place specified by the .1
modifier.
Hard Examples:
1. Matrix Multiplication:
#include <stdio.h>
#define ROWS 3
#define COLS 3
void matrix_multiplication(int a[][COLS], int b[][COLS], int result[][COLS]) {
int i, j, k;
for(i = 0; i < ROWS; i++) {
for(j = 0; j < COLS; j++) {
result[i][j] = 0;
for(k = 0; k < COLS; k++) {
result[i][j] += a[i][k] * b[k][j];
}
}
}
}
void print_matrix(int matrix[][COLS]) {
int i, j;
for(i = 0; i < ROWS; i++) {
for(j = 0; j < COLS; j++) {
printf("%d ", matrix[i][j]);
}
printf("\n");
}
}
int main() {
int matrix1[ROWS][COLS] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int matrix2[ROWS][COLS] = {
{9, 8, 7},
{6, 5, 4},
{3, 2, 1}
};
int result[ROWS][COLS];
matrix_multiplication(matrix1, matrix2, result);
printf("Matrix 1:\n");
print_matrix(matrix1);
printf("\nMatrix 2:\n");
print_matrix(matrix2);
printf("\nResult Matrix:\n");
print_matrix(result);
return 0;
}
Output:
Matrix 1:
1 2 3
4 5 6
7 8 9
Matrix 2:
9 8 7
6 5 4
3 2 1
Result Matrix:
30 24 18
84 69 54
138 114 90
In above example, we have defined a function named matrix_multiplication
that takes two 2D arrays a
and b
of size ROWS x COLS
and performs matrix multiplication on them to generate a third 2D array result
. We have also defined another function named print_matrix
that takes a 2D array matrix
of size ROWS x COLS
and prints it to the console.
In the main
function, we have declared three 2D arrays named matrix1
, matrix2
, and result
, and initialized the first two arrays with values. We have then called the matrix_multiplication
function with matrix1
, matrix2
, and result
as arguments. Finally, we have printed the original two matrices and the resulting matrix using the print_matrix
function.
2. Sorting an Array of String:
#include <stdio.h>
#include <string.h>
#define MAX_SIZE 10
#define MAX_LENGTH 20
void sort_strings(char strings[][MAX_LENGTH], int size) {
int i, j;
char temp[MAX_LENGTH];
for(i = 0; i < size - 1; i++) {
for(j = i + 1; j < size; j++) {
if(strcmp(strings[i], strings[j]) > 0) {
strcpy(temp, strings[i]);
strcpy(strings[i], strings[j]);
strcpy(strings[j], temp);
}
}
}
}
int main() {
char strings[MAX_SIZE][MAX_LENGTH] = {
"apple",
"orange",
"banana",
"grapes",
"pear",
"kiwi",
"mango",
"watermelon",
"pineapple",
"pomegranate"
};
printf("Unsorted Strings:\n");
int i;
for(i = 0; i < MAX_SIZE; i++) {
printf("%s\n", strings[i]);
}
sort_strings(strings, MAX_SIZE);
printf("\nSorted Strings:\n");
for(i = 0; i < MAX_SIZE; i++) {
printf("%s\n", strings[i]);
}
return 0;
}
Output:
Unsorted Strings:
apple
orange
banana
grapes
pear
kiwi
mango
watermelon
pineapple
pomegranate
Sorted Strings:
apple
banana
grapes
kiwi
mango
orange
pear
pineapple
pomegranate
watermelon
In above example, we have defined a function named sort_strings
that takes a 2D array of strings strings
and an integer size
as arguments, and sorts the strings in the array in lexicographic order using the strcmp
function and the strcpy
function.
In the main
function, we have declared a 2D array of strings named strings
with a maximum size of MAX_SIZE
and a maximum length of MAX_LENGTH
, and initialized it with 10 strings. We have then printed the unsorted strings using a for loop and the %s
format specifier in the printf
function. We have then called the sort_strings
function with strings
and MAX_SIZE
as arguments to sort the strings in the array. Finally, we have printed the sorted strings using another for loop and the %s
format specifier in the printf
function.
Rules to note while using Array in C:
- The index of the first element of an array is always 0.
- The index of the last element of an array is always
array_size - 1
. - Arrays have a fixed size, which cannot be changed during runtime.
- Array elements can be accessed using an index or a pointer.
- Arrays can be passed to functions as arguments by reference.