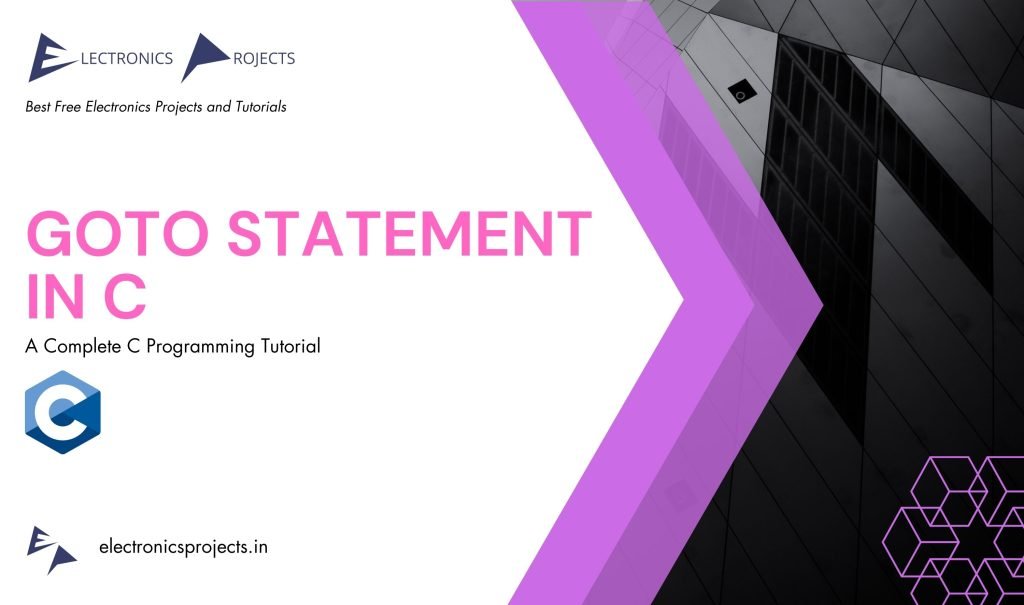
What is goto statement in C?
The goto
statement in C is a control flow statement that transfers program control to a labeled statement elsewhere in the code. It allows the programmer to jump from one part of the code to another part without executing the code in between. Also, the goto
statement can be used in any loop or block of code
The goto
statement is not recommended in modern programming as it can make the code difficult to understand and maintain. It can lead to spaghetti code and can make debugging and testing more difficult. However, it can still be useful in certain situations such as breaking out of deeply nested loops or handling errors in a complex way.
Examples of goto statement in C:
1. Handling Input Errors using goto Statement
#include <stdio.h>
int main() {
int num;
printf("Enter a positive integer: ");
scanf("%d", &num);
if (num <= 0) {
goto error;
}
printf("The square of %d is %d\n", num, num*num);
return 0;
error:
printf("Error: Invalid input\n");
return 1;
}
Output;
Enter a positive integer: -5
Error: Invalid input
Explanation:
In above example, the program prompts the user to enter a positive integer. If the user enters a non-positive integer, the program jumps to the error
label using goto
statement, which prints an error message and returns with a non-zero exit code. If the input is valid, the program calculates and prints the square of the input.
2. Breaking Out of Nested Loops using goto Statement
#include <stdio.h>
int main() {
int i, j;
for (i = 1; i <= 3; i++) {
for (j = 1; j <= 3; j++) {
if (i == 2 && j == 2) {
goto end;
}
printf("%d%d ", i, j);
}
printf("\n");
}
end:
printf("\nEnd of program\n");
return 0;
}
Output;
11 12 13
21
31 32 33
End of program
Explanation:
In above example, the program uses nested loops to print a matrix of numbers from 11 to 33, except for the element at row 2, column 2. When the program reaches that element, it jumps to the end
label using goto
statement, which prints a message and terminates the program.
3. Implementing a Simple Loop using goto Statement
#include <stdio.h>
int main() {
int num, sum = 0, i = 1;
loop:
if (i > 5) {
goto end;
}
printf("Enter integer #%d: ", i);
scanf("%d", &num);
sum += num;
i++;
goto loop;
end:
printf("The sum is %d\n", sum);
return 0;
}
Output;
Enter integer #1: 3
Enter integer #2: 5
Enter integer #3: 1
Enter integer #4: 2
Enter integer #5: 4
The sum is 15
Explanation:
In above example, the program uses a goto
statement to implement a loop that reads 5 integers from the user and computes their sum. The loop
label marks the beginning of the loop, and the end
label marks the end of the loop. Inside the loop, the program reads an integer, adds it to the sum, and increments the loop counter. When the loop counter reaches 6, the program jumps to the end
label, which prints the final sum and terminates the program.
Rules to be followed when using goto statement in C:
- Limit the use of
goto
statement: Use it only when it is absolutely necessary, such as breaking out of nested loops or handling error conditions. - Always label the target statement: A
goto
statement must always jump to a labeled statement. The label can appear anywhere in the same function, but it must not be inside a control structure like a loop or a switch. - Use descriptive and meaningful labels: The label should reflect the purpose of the statement that it marks. Avoid using generic or ambiguous labels like “exit” or “error”.
- Avoid jumping into a block of code: A
goto
statement should only jump forward to a labeled statement. Avoid using it to jump backward into a block of code, as it can make the code harder to understand and debug. - Keep the code structure simple: Avoid using
goto
statement to implement complex control structures or to bypass the normal flow of execution. Instead, use structured constructs like loops, conditionals, and functions. - Test and verify the code: When you use
goto
statement, it can be difficult to ensure that the code behaves as intended. Therefore, it is important to test and verify the code thoroughly, and to document the behavior of the code carefully.