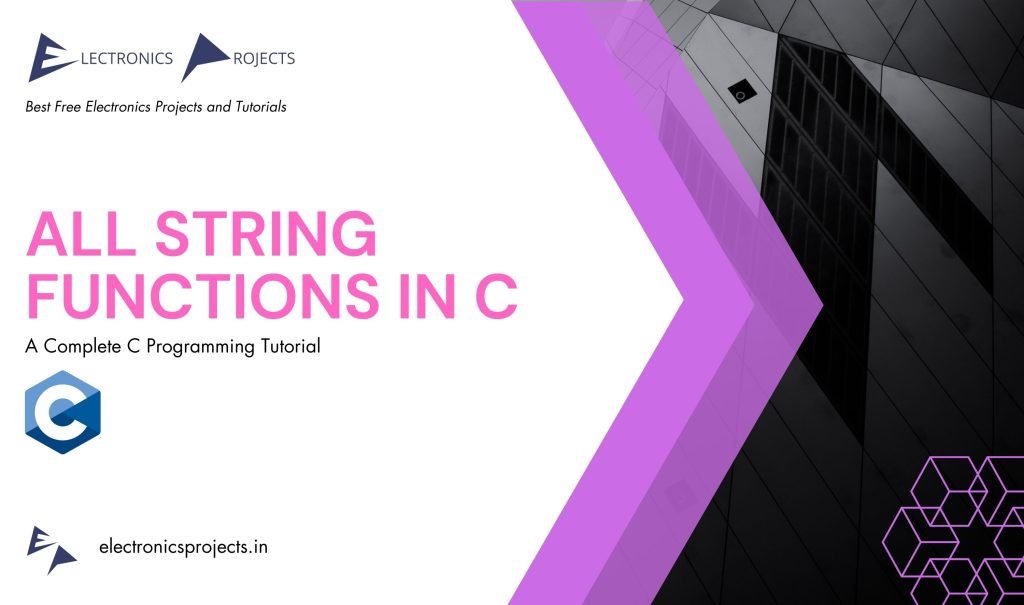
What is string function in C ?
In C programming, string functions are a set of built-in functions that operate on character arrays (strings) and provide various operations for manipulating and working with strings. These functions are included in the standard C library header file <string.h>
. String functions are used to perform common string operations such as copying, concatenating, comparing, searching, and manipulating strings.
Here is a table explaining some commonly used string functions in C:
Sr. No. | Function Name | Decription |
---|---|---|
1 | strlen(string) | Returns the length of a string (number of characters) excluding the null character. |
2 | strcpy(destination, source) | Copies a string from source to destination. |
3 | strncpy(destination, source, n) | Copies a certain number of characters from source to destination. |
4 | strcat(destination, source) | Concatenates (appends) one string to the end of another string. |
5 | strncat(destination, source, n) | Concatenates a certain number of characters from one string to the end of another string. |
6 | strcmp(string1, string2) | Compares two strings and returns an integer indicating the relation between them. |
7 | strncmp(string1, string2, n) | Compares a certain number of characters of two strings and returns an integer indicating the relation between them. |
8 | strchr(string, c) | Searches for the first occurrence of a character in a string and returns a pointer to that character. |
9 | strrchr(string, c) | Searches for the last occurrence of a character in a string and returns a pointer to that character. |
10 | strstr(string1, string2) | Searches for the first occurrence of a substring in a string and returns a pointer to that substring. |
11 | strtok(string, delimiter) | Breaks a string into a series of tokens based on a delimiter. |
12 | sprintf(string, format, ...) | Writes formatted data to a string (similar to printf ). |
13 | sscanf(string, format, ...) | Reads formatted data from a string (similar to scanf ). |
1. strlen(string)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
int length = strlen(str);
printf("Length of the string: %d\n", length);
return 0;
}
Output:
Length of the string: 13
Explanation:
This example demonstrates the usage of strlen
function to find the length of a string. The function strlen
returns an integer representing the length of the provided string excluding the null character.
2. strcpy(destination, source)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[20];
strcpy(destination, source);
printf("Copied string: %s\n", destination);
return 0;
}
Output:
Copied string: Hello, World!
Explanation:
This example shows how to use strcpy
to copy a string from the source to the destination. The strcpy
function copies the contents of the source string to the destination string.
3. strncpy(destination, source, n)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[10];
strncpy(destination, source, 5);
destination[5] = '\0';
printf("Copied string: %s\n", destination);
return 0;
}
Output:
Copied string: Hello
Explanation:
This example demonstrates the usage of strncpy
function to copy a certain number of characters (5 characters in this case) from the source to the destination. The strncpy
function copies the specified number of characters or until it reaches the null character, whichever comes first. It is important to manually append the null character to ensure the resulting string is properly terminated.
4. strcat(destination, source)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char destination[20] = "Hello";
char source[] = ", World!";
strcat(destination, source);
printf("Concatenated string: %s\n", destination);
return 0;
}
Output:
Concatenated string: Hello, World!
Explanation:
This example shows how to use strcat
to concatenate (append) one string to the end of another. The strcat
function appends the source string to the destination string.
5. strncat(destination, source, n)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char destination[20] = "Hello";
char source[] = ", World!";
strncat(destination, source, 5);
printf("Concatenated string: %s\n", destination);
return 0;
}
Output:
Concatenated string: Hello, Wor
Explanation:
This example demonstrates the usage of strncat
function to concatenate a certain number of characters (5 characters in this case) from the source to the end of the destination. The strncat
function appends the specified number of characters from the source string to the destination string or until it reaches the null character, whichever comes first.
6. strcmp(string1, string2)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Hello";
char str2[] = "World";
int result = strcmp(str1, str2);
if (result == 0) {
printf("Strings are equal\n");
} else if (result < 0) {
printf("String 1 is less than String 2\n");
} else {
printf("String 1 is greater than String 2\n");
}
return 0;
}
Output:
String 1 is less than String 2
Explanation:
This example showcases the usage of strcmp
function to compare two strings. The strcmp
function returns an integer value indicating the relation between the strings: 0 if both strings are equal, a negative value if the first string is less than the second, or a positive value if the first string is greater than the second.
7. strncmp(string1, string2, n)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Hello";
char str2[] = "Help";
int result = strncmp(str1, str2, 3);
if (result == 0) {
printf("Strings are equal\n");
} else if (result < 0) {
printf("String 1 is less than String 2\n");
} else {
printf("String 1 is greater than String 2\n");
}
return 0;
}
Output:
Strings are equal
Explanation:
This example demonstrates the usage of strncmp
function to compare a certain number of characters (3 characters in this case) of two strings. The strncmp
function returns an integer value indicating the relation between the strings, similar to strcmp
.
8. strchr(string, c)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
char *ptr = strchr(str, 'W');
printf("Character found: %c\n", *ptr);
return 0;
}
Output:
Character found: W
Explanation:
This example showcases the usage of strchr
function to search for the first occurrence of a character in a string. The strchr
function returns a pointer to the first occurrence of the specified character in the string or NULL if the character is not found.
9. strrchr(string, c)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
char *ptr = strrchr(str, 'o');
printf("Character found: %c\n", *ptr);
return 0;
}
Output:
Character found: o
Explanation:
This example demonstrates the usage of strrchr
function to search for the last occurrence of a character in a string. The strrchr
function returns a pointer to the last occurrence of the specified character in the string or NULL if the character is not found.
10 strstr(string1, string2)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Hello, World!";
char str2[] = "World";
char *ptr = strstr(str1, str2);
printf("Substring found: %s\n", ptr);
return 0;
}
Output:
Substring found: World!
Explanation:
This example showcases the usage of strstr
function to search for the first occurrence of a substring in a string. The strstr
function returns a pointer to the first occurrence of the specified substring in the string or NULL if the substring is not found.
11. strtok(string, delimiter)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello,World,How,Are,You";
char *token = strtok(str, ",");
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, ",");
}
return 0;
}
Output:
Hello
World
How
Are
You
Explanation:
This example demonstrates the usage of strtok
function to break a string into a series of tokens based on a delimiter. The strtok
function returns a pointer to the next token found in the string or NULL if there are no more tokens.
12. sprintf(string, format, …)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str[20];
int number = 42;
sprintf(str, "The answer is %d", number);
printf("%s\n", str);
return 0;
}
Output:
The answer is 42
Explanation:
This example shows how to use sprintf
function to write formatted data to a string. The sprintf
function formats the data according to the specified format string and stores it in the provided string.
13. sscanf(string, format, …)
Examples:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "John Doe 25";
char name[20];
int age;
sscanf(str, "%s %s %d", name, name, &age);
printf("Name: %s\n", name);
printf("Age: %d\n", age);
return 0;
}
Output:
Name: Doe
Age: 25
Explanation:
This example demonstrates the usage of sscanf
function to read formatted data from a string. The sscanf
function scans the input string according to the specified format string and stores the extracted values in the provided variables.