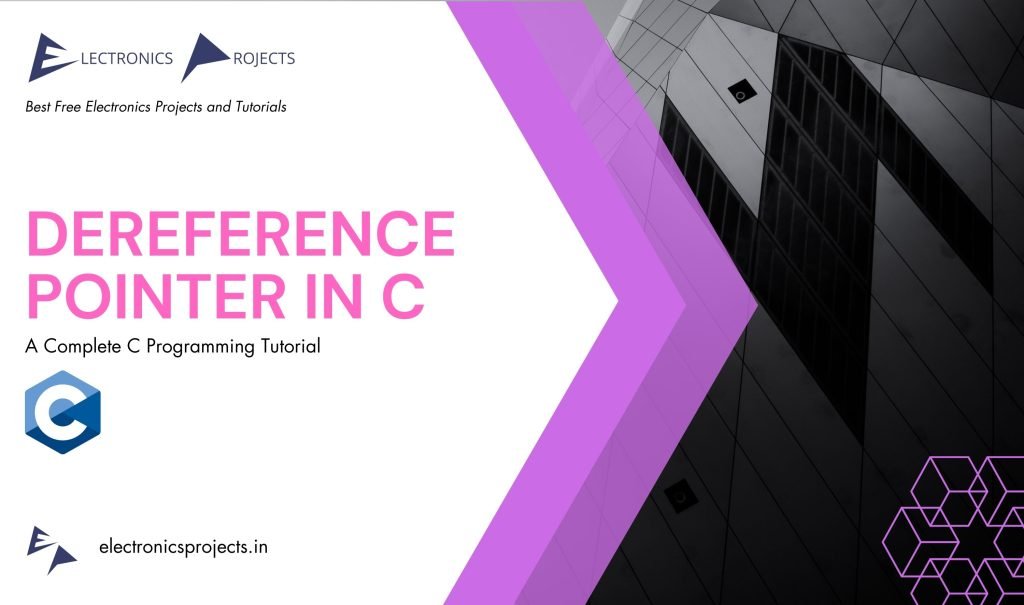
What is Dereference Pointer in C ?
In C, a pointer is a variable that holds the memory address of another variable. Dereferencing a pointer means accessing the value stored at the memory location pointed to by the pointer. The syntax for dereferencing a pointer in C is to use the asterisk (*) operator in front of the pointer variable.
To dereference a pointer in C, you need to follow these steps:
- Declare a pointer variable of the appropriate data type.
- Assign the memory address of a variable to the pointer.
- Use the dereference operator (*) to access the value stored at the memory location pointed to by the pointer.
Examples of Dereference Pointer in C
Example 1
#include <stdio.h>
int main() {
int num = 42;
int *ptr = # // Pointer initialization with the address of 'num'
printf("Value of num: %d\n", *ptr); // Dereferencing 'ptr' to access the value
return 0;
}
Output:
Value of num: 42
Explanation:
In above example, we declare an integer variable num
and assign it the value of 42. Then, we declare an integer pointer ptr
and initialize it with the address of num
using the &
operator. Finally, we use the dereference operator *
to access the value stored at the memory location pointed to by ptr
, which is the value of num
. The program prints the value of num
, which is 42.
Example 2
#include <stdio.h>
void changeValue(int *ptr) {
*ptr = 99; // Dereferencing 'ptr' and assigning a new value
}
int main() {
int num = 42;
int *ptr = # // Pointer initialization with the address of 'num'
printf("Before: %d\n", num);
changeValue(ptr); // Passing the pointer to a function
printf("After: %d\n", num);
return 0;
}
Output:
Before: 42
After: 99
Explanation:
In above example, we have a function called changeValue
that takes a pointer to an integer as a parameter. Inside the function, we dereference the pointer using *ptr
and assign a new value of 99 to the variable stored at the memory location pointed to by the pointer. In the main
function, we declare an integer variable num
and assign it the value of 42. Then, we declare an integer pointer ptr
and initialize it with the address of num
using the &
operator. We pass the pointer ptr
to the changeValue
function, which modifies the value of num
through dereferencing. Finally, we print the value of num
before and after the function call, demonstrating how the dereferencing operation can modify the value of a variable indirectly.
Rules to be followed when using dereference pointer in C
- Ensure that the pointer is pointing to a valid memory location before dereferencing it.
- Avoid dereferencing a null pointer, as it can lead to undefined behavior.
- Make sure to match the data type of the pointer and the type of the variable being accessed through dereferencing.
- Be cautious when modifying the value of a variable through dereferencing, as it can introduce bugs if not handled properly.
- Avoid accessing or modifying memory outside the bounds of the allocated memory, as it can result in memory corruption or segmentation faults.