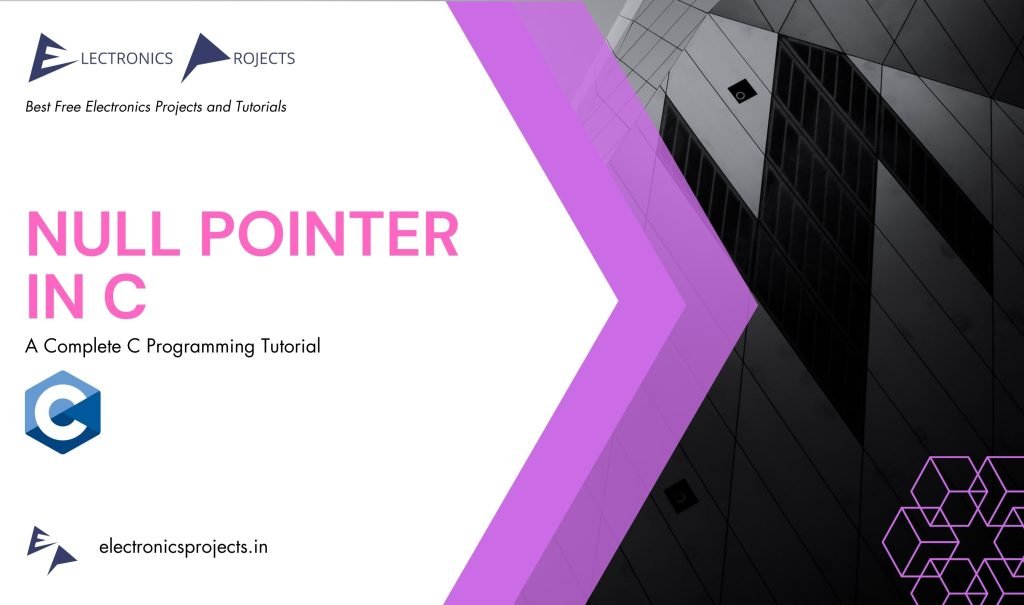
What is Null Pointer in C ?
In C, a null pointer is a pointer that does not point to any valid memory location. It is represented by the constant value “NULL” (or “0” in older versions of C). It is used to indicate that a pointer variable is not currently pointing to any object or memory location.
The syntax of a null pointer in C is simply the “NULL” keyword or the value “0”. Here’s an example of how it can be declared:
int *ptr = NULL;
In this example, ptr
is a null pointer of type int*
. It is initialized to NULL, indicating that it does not currently point to any valid memory location.
There are several reasons why null pointers are used in C:
- Initialization: Null pointers are often used to initialize pointers before they are assigned valid addresses.
- Indicating absence of data: Null pointers are used to represent the absence of an object or the absence of a valid memory location.
Examples of Null Pointer in C
Example 1: Checking if a pointer is null
#include <stdio.h>
int main() {
int *ptr = NULL;
if (ptr == NULL) {
printf("Pointer is null\n");
} else {
printf("Pointer is not null\n");
}
return 0;
}
Output:
Pointer is null
Explanation:
In above example, we declare a pointer ptr
and initialize it to NULL. Then, we check if the pointer is null using the ==
comparison operator. Since ptr
is indeed null, the condition is true, and it prints “Pointer is null.”
Example 2: Using a null pointer to terminate a linked list
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
int main() {
Node* head = NULL;
Node* current;
// Creating a linked list
for (int i = 0; i < 5; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = i;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
current = head;
} else {
current->next = newNode;
current = current->next;
}
}
// Printing the linked list
current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
return 0;
}
Output:
0 1 2 3 4
Explanation:
In above example, we create a linked list by dynamically allocating nodes using malloc()
. We use a null pointer (NULL
) to indicate the end of the list. Initially, both head
and current
pointers are set to NULL. As new nodes are created and appended to the list, the next
pointer of the last node is set to NULL. Finally, we traverse the linked list by starting from the head
and print the data of each node until we reach the end (null pointer).
Different ways to use null pointer in C
- Initializing pointers: Set pointers to NULL before assigning them valid memory addresses.
- Checking for nullness: Use null pointers in conditional statements to check if a pointer is null before accessing its contents.
- Terminating data structures: Use null pointers to indicate the end of linked lists, trees, or other data structures.
- Error handling: Functions returning pointers can use null pointers to indicate failure or absence of a valid result.
Rules to be followed while using null pointers in C
- Avoid dereferencing null pointers: Attempting to access the contents of a null pointer can lead to undefined behavior and crashes. Always check if a pointer is null before using it.
- Assign valid addresses before accessing: Make sure to assign a valid memory address to a null pointer before accessing or modifying its contents.
- Be cautious with pointer arithmetic: Performing pointer arithmetic on null pointers can lead to undefined behavior. It’s best to avoid such operations.
- Free dynamically allocated memory: If you allocate memory dynamically using
malloc()
,calloc()
, orrealloc()
, remember to free the memory usingfree()
when it is no longer needed.