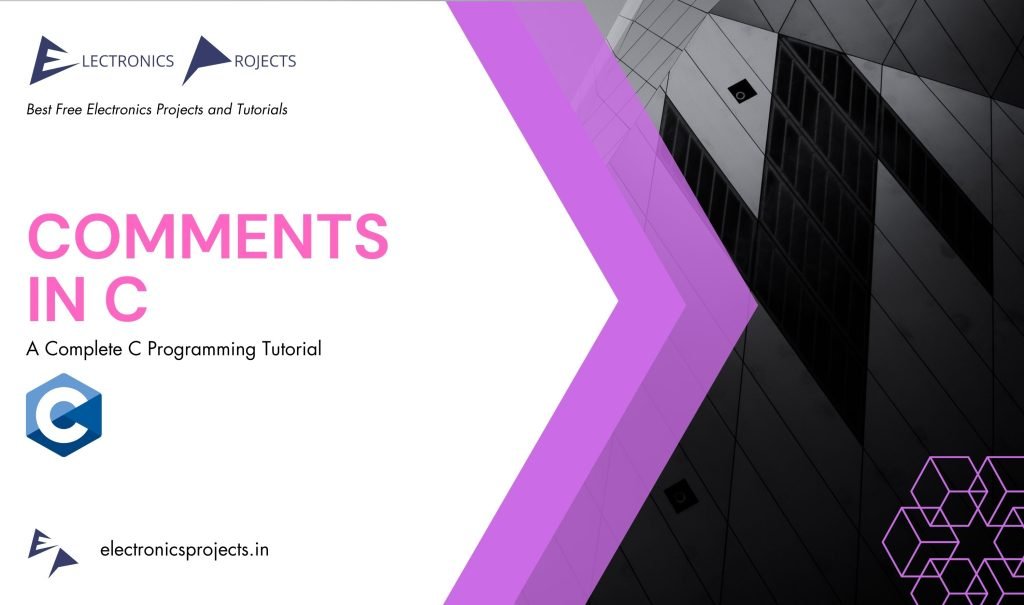
What is Comments in C?
In C programming, comments are lines of text that are added to the source code but are ignored by the compiler. They are used to document the code, provide information about the code, and make it more readable for other developers.
There are two main reasons to use comments in C:
- To explain what the code is doing: Comments can be used to explain the purpose of the code, its functionality, and its expected behavior. This is especially important when working on large projects with many collaborators.
- To make the code more readable: Comments can be used to break up long blocks of code into smaller, more manageable sections. They can also be used to highlight important parts of the code or to indicate areas that need further attention or revision.
Example:
// This is a comment
Explanation:
In this example, the comment starts with two forward slashes, which indicate that it is a single-line comment. The text following the slashes is the comment itself, which explains the purpose of the program.
1. Single Line Comments:
A single-line comment starts with two forward slashes (//) and continues until the end of the line. Anything written after the // is ignored by the compiler.
Example:
// This is a single-line comment in C programming language
Explanation:
In this example, the comment starts with // and ends at the end of the line. The text in the comment is ignored by the compiler.
2. Multiline Comment:
A multi-line comment starts with a forward slash and an asterisk (/) and ends with an asterisk and a forward slash (/). Anything written between the /* and */ is ignored by the compiler.
Example:
/* This is a multi-line comment in C programming language
It can span multiple lines and is useful for commenting out blocks of code
that you want to temporarily disable. */
Explanation:
In this example, the comment starts with /* and ends with /. The text between the / and */ is ignored by the compiler, even if it spans multiple lines.
Rules while using Comments in C:
- Comments should be clear, concise, and easy to understand.
- They should explain the purpose of the code, its functionality, and its expected behavior.
- They should be written in plain English and avoid technical jargon.
- Single-line comments should be used for short comments that fit on a single line.
- Multi-line comments should be used for longer comments that span multiple lines.
- Comments should be used sparingly and only when necessary to explain the code. Over-commenting can make the code harder to read.