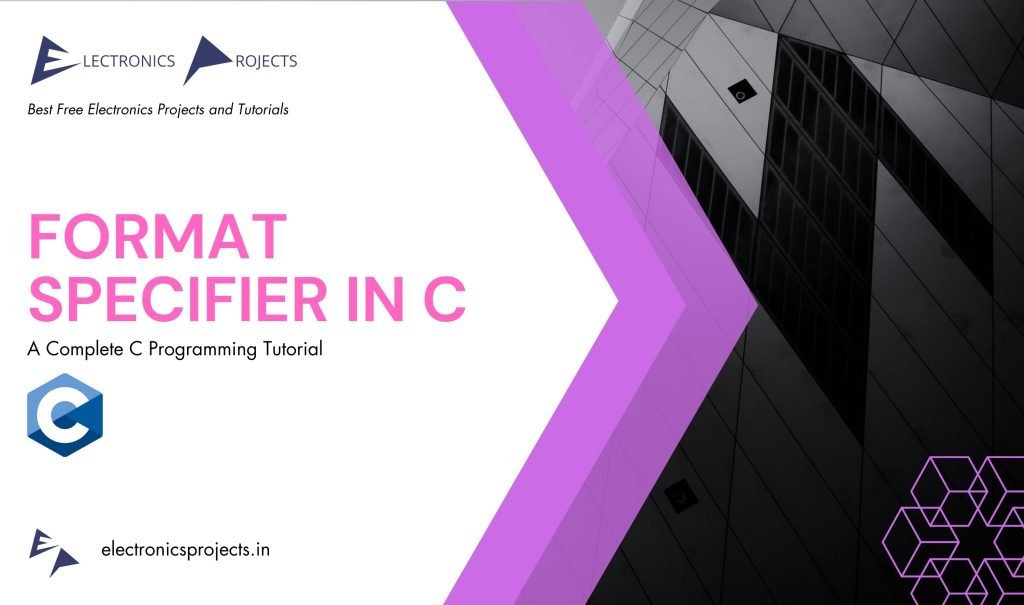
What is Format Specifier in C?
Format specifiers in C are special characters that are used to indicate the type of data that is being input or output through standard input/output functions like scanf() and printf(). The format specifier provides a way to tell the compiler how to interpret and format the input or output data.
Format specifiers are used to ensure that the data is correctly interpreted and displayed. Without format specifiers, the data could be interpreted or displayed incorrectly, leading to errors in the program or unexpected results.
Types of Format Specifiers in C:
Sr. No. | Format Specifier | Type of Data |
---|---|---|
1 | %d | Decimal Integer |
2 | %i | Integer |
3 | %0 | Octal Integer |
4 | %u | Unsigned Integer |
5 | %x | Hexadecimal Integer |
6 | %f | Floating-Point Number |
7 | %e | Exponential Notation |
8 | %g | Use the Shorted Representation (Either %e or %f) |
9 | %c | Character |
10 | %s | String |
11 | %p | Point Address |
12 | %% | Prints a % Symbol |
Example:
Here’s an example program that demonstrates the use of all the format specifiers:
#include <stdio.h>
int main() {
int i = 42;
char c = 'A';
float f = 3.14;
double d = 3.14159265;
char s[] = "Hello, World!";
void* p = &i;
printf("Integer: %d, %i, %o, %u, %x\n", i, i, i, i, i);
printf("Float: %f, %e, %g\n", f, f, f);
printf("Double: %f, %e, %g\n", d, d, d);
printf("Character: %c\n", c);
printf("String: %s\n", s);
printf("Pointer Address: %p\n", p);
printf("Percent Symbol: %%\n");
return 0;
}
Output:
Integer: 42, 42, 52, 42, 2a
Float: 3.140000, 3.140000e+00, 3.14
Double: 3.141593, 3.141593e+00, 3.14159
Character: A
String: Hello, World!
Pointer Address: 0x7ffee049f9c4
Percent Symbol: %
Explanation:
In this program, we have defined variables of different data types and used the corresponding format specifiers to print their values. Note that the output may vary depending on the system and compiler being used.
Rules to follow when using Format Specifiers:
- The format specifier must match the data type of the argument being passed.
- The order of the arguments must match the order of the format specifiers in the format string.
- The format string must be a constant string literal.
- The format string should be null-terminated.
- The number of arguments must match the number of format specifiers in the format string.
Modification that can be made while using Format Specifiers:
- Field width: You can specify the minimum width of the field for the output using a number between the percent sign and the conversion character. For example, %5d would output an integer with a minimum width of 5 characters, padded with spaces on the left if necessary.
- Precision: For floating-point numbers, you can specify the number of digits to display after the decimal point using a period (.) and a number after the field width. For example, %.2f would output a floating-point number with 2 digits after the decimal point.
- Length modifiers: You can modify the length of the input argument using length modifiers. For example, %ld would output a long integer, and %Lf would output a long double.
- Flags: You can use flags to modify the format of the output. For example, the minus sign (-) flag left-justifies the output, and the plus sign (+) flag always shows the sign of the number.
Here’s an example of modifications to format specifiers:
int num = 42;
printf("%10d\n", num); // output: " 42" (minimum width of 10 characters)
printf("%.2f\n", 3.1415); // output: "3.14" (2 digits after the decimal point)
printf("%ld\n", 123456789012345); // output: "123456789012345" (long integer)
printf("%+d\n", -10); // output: "-10" (always show the sign)