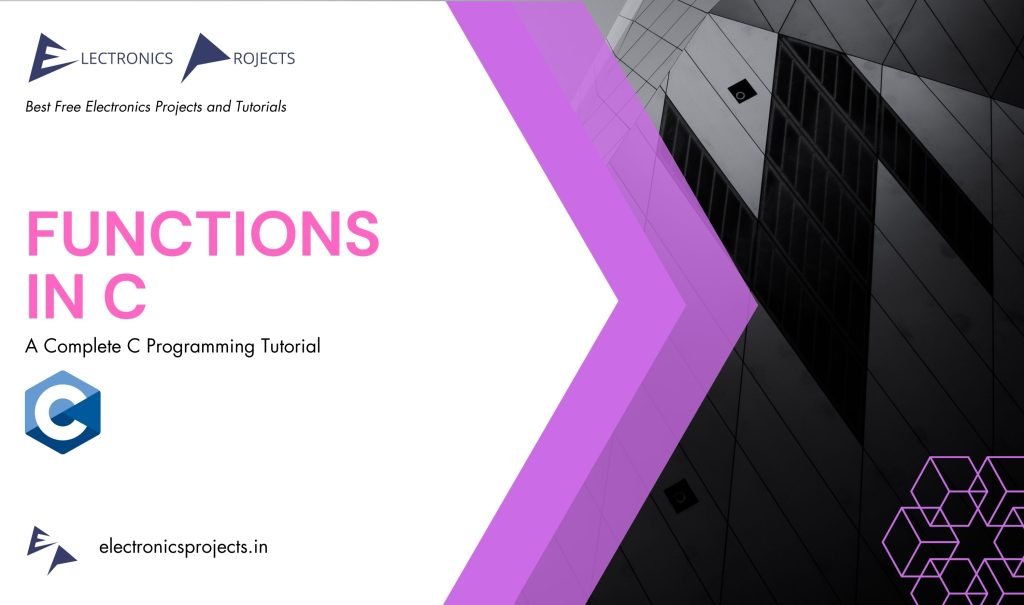
What is Function in C?
A function in C is a block of code that performs a specific task. It can be called multiple times from different parts of the program. Functions are used to modularize code, improve code readability, and reduce redundancy.
1. Declaring a Function:
Functions are declared in C using the following syntax:
return_type function_name(parameter list) {
// code to be executed
return value;
}
Here, the return_type is the data type of the value returned by the function, function_name is the name of the function, and the parameter list contains the variables that the function accepts as input. The function body contains the code to be executed, and the return statement is used to return a value to the calling function.
Example:
int addNumbers(int a, int b) {
int result = a + b;
return result;
}
Explanation:
In above example, the return type of the function is int
(integer), and the function name is addNumbers
. The function takes two parameters of type int
(a
and b
). The code inside the function adds the two input integers and stores the result in a variable called result
. Finally, the function returns the value of result
using the return
statement.
2. Calling a Function:
Functions are called in C using the following syntax:
function_name(arguments);
Here, function_name is the name of the function and arguments are the values passed to the function as input.
Example:
int sum = addNumbers(x, y);
Now lets see a complete example with both function declaration and calling:
#include <stdio.h>
// function prototype
int addNumbers(int a, int b);
int main() {
int x = 5;
int y = 7;
int sum = addNumbers(x, y);
printf("The sum of %d and %d is %d\n", x, y, sum);
return 0;
}
// function definition
int addNumbers(int a, int b) {
int result = a + b;
return result;
}
Output:
The sum of 5 and 7 is 12
Explanation:
In above example, we have included the function prototype for addNumbers
before the main
function. This allows the compiler to know the function’s signature before it is used in the program.
Inside the main
function, we declare two integer variables x
and y
with values 5 and 7, respectively. We then call the addNumbers
function with these two values as input arguments and store the result in a new integer variable called sum
. Finally, we print the result using the printf
function.
Types of Functions in C:
There are two types of functions in C:
- Library Functions
- User-Defined Functions.
1. Library Functions:
A library function in C is a predefined function that is provided in a library and can be used by the programmer. These functions are already implemented and tested, so they can be used directly without having to write any code. The C standard library contains a large number of useful functions for various purposes, such as input/output, string handling, math operations, and memory management.
Example:
Here’s an example of using the printf
function, which is a library function that is used to print output to the console:
#include <stdio.h>
int main() {
printf("Hello, world!\n");
return 0;
}
Output:
Hello, world!
Explanation:
In above example, we have included the stdio.h
library, which contains the definition of the printf
function. Inside the main
function, we call the printf
function with a string argument "Hello, world!\n"
. The \n
at the end of the string is a special character that represents a newline character, so the output will be printed on a new line. Finally, we return 0 to indicate that the program executed successfully.
2. User-Defined Functions:
A user-defined function in C is a function that is created by the programmer to perform a specific task. These functions can be defined and used in the same way as library functions, but they are tailored to the programmer’s specific needs. User-defined functions can help to modularize code, improve code readability, and reduce redundancy.
Example:
Here’s an example of a user-defined function that calculates the factorial of a positive integer:
#include <stdio.h>
// function prototype
int factorial(int n);
int main() {
int num = 5;
int fact = factorial(num);
printf("Factorial of %d is %d\n", num, fact);
return 0;
}
// function definition
int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
Output:
Factorial of 5 is 120
Explanation:
In this example, we have defined a user-defined function factorial
that takes an integer input n
and returns its factorial. Inside the function, we use recursion to calculate the factorial, which is the product of all positive integers up to and including n
.
In the main
function, we call the factorial
function with an input argument of 5 and store the result in a new integer variable called fact
. Finally, we print the result using the printf
function.
Different ways to declare a Function in C:
1. Declaration of function prototype
A function prototype is a declaration of a function that tells the compiler about the function’s name, return type, and parameter types. This allows the compiler to check that the function is being used correctly before the actual function definition is encountered. Function prototypes are usually placed at the top of a source file or in a header file, so that they can be easily accessed by other functions in the program.
Example:
Here’s an example of a function prototype for a function that calculates the sum of two integers:
#include <stdio.h>
// function prototype
int addNumbers(int a, int b);
int main() {
int x = 5;
int y = 7;
int sum = addNumbers(x, y);
printf("The sum of %d and %d is %d\n", x, y, sum);
return 0;
}
// function definition
int addNumbers(int a, int b) {
int result = a + b;
return result;
}
Output:
The sum of 5 and 7 is 12
Explanation:
In above example, we have included the function prototype for addNumbers
before the main
function. The prototype tells the compiler that addNumbers
is a function that takes two integer parameters and returns an integer value. This allows the compiler to check that the function is being called correctly inside the main
function.
2. Declaration of Function Definition
A function definition is a block of code that specifies the actual implementation of a function. It includes the function’s name, return type, parameter types, and the code to be executed when the function is called. Function definitions are usually placed below the main
function or in a separate source file.
Example:
Here’s an example of a function definition for the same function addNumbers
as in the previous example:
#include <stdio.h>
// function prototype (not needed if the function definition is placed before main)
int addNumbers(int a, int b);
int main() {
int x = 5;
int y = 7;
int sum = addNumbers(x, y);
printf("The sum of %d and %d is %d\n", x, y, sum);
return 0;
}
// function definition
int addNumbers(int a, int b) {
int result = a + b;
return result;
}
Output:
The sum of 5 and 7 is 12
Explanation:
In above example, we have defined the function addNumbers
below the main
function. The function definition includes the same information as the function prototype, but also includes the code to be executed when the function is called. Inside the function, we declare a new integer variable called result
and assign it the value of a + b
. We then return result
as the function’s output.
When the addNumbers
function is called inside the main
function, the code inside the function definition is executed and the resulting value is returned to the main
function.
3, Inline Functions
An inline function is a function that is expanded in-line at the point where it is called, rather than being called through the usual function call mechanism. Inline functions are used to eliminate the overhead of function calls, which can improve performance in some cases.
Example:
Here’s an example of an inline function that calculates the square of a number:
#include <stdio.h>
// inline function
inline int square(int num) {
return num * num;
}
int main() {
int x = 5;
int square_x = square(x);
printf("The square of %d is %d\n", x, square_x);
return 0;
}
Output:
The square of 5 is 25
Explanation:
In above example, we have declared the square
function as an inline function using the inline
keyword. When the function is called inside the main
function, the code inside the function is expanded in-line, eliminating the overhead of a function call.
4. Recursive Functions
A recursive function is a function that calls itself either directly or indirectly. Recursive functions are used to solve problems that can be broken down into smaller, similar problems. Each time the function is called, it solves a smaller version of the original problem, until the problem is small enough to be solved directly.
Example:
Here’s an example of a recursive function that calculates the factorial of a number:
#include <stdio.h>
// recursive function
int factorial(int num) {
if (num == 0) {
return 1;
}
else {
return num * factorial(num - 1);
}
}
int main() {
int x = 5;
int fact_x = factorial(x);
printf("The factorial of %d is %d\n", x, fact_x);
return 0;
}
Output:
The factorial of 5 is 120
Explanation:
In above example, we have defined the factorial
function as a recursive function. Inside the function, we check if the input number is equal to 0, in which case we return 1. If the input number is not equal to 0, we return the product of the input number and the factorial of the input number minus 1.
When the factorial
function is called inside the main
function with the input number 5, the function calls itself recursively with input numbers 4, 3, 2, and 1, until it reaches the base case of 0. Then the function returns the factorial of 1, which is multiplied by 2, 3, 4, and 5 to get the factorial of 5.
5. Function Pointers
A function pointer is a variable that stores the address of a function. Function pointers can be used to pass a function as an argument to another function, or to call a function through a pointer.
Example:
Here’s an example of a function pointer that points to a function that takes two integer arguments and returns an integer:
#include <stdio.h>
// function that takes two integers and returns an integer
int add(int a, int b) {
return a + b;
}
int main() {
// declare a function pointer that points to the add function
int (*ptr)(int, int);
ptr = &add;
// call the add function through the function pointer
int result = (*ptr)(2, 3);
printf("The result is %d\n", result);
return 0;
}
Output:
The result is 5
Explanation:
In above example, we have declared a function pointer ptr
that points to the add
function using the &
operator. We then call the add
function through the function pointer by dereferencing the pointer with the *
operator and passing in two arguments. The result of the function call is stored in the result
variable, which is then printed to the console.
Another example of function pointers for no reason:
Here’s an example program that demonstrates how to use a function pointer:
#include <stdio.h>
int add(int x, int y) {
return x + y;
}
int subtract(int x, int y) {
return x - y;
}
int main() {
// declare function pointers
int (*p_add)(int, int);
int (*p_subtract)(int, int);
// assign function addresses to pointers
p_add = &add;
p_subtract = &subtract;
// call functions using pointers
int num1 = 10;
int num2 = 5;
int sum = (*p_add)(num1, num2);
int difference = (*p_subtract)(num1, num2);
printf("The sum of %d and %d is %d\n", num1, num2, sum);
printf("The difference between %d and %d is %d\n", num1, num2, difference);
return 0;
}
Output:
The sum of 10 and 5 is 15
The difference between 10 and 5 is 5
Explanation:
In above example, we define two functions add
and subtract
that take two integers as arguments and return an integer result. We then declare two function pointers p_add
and p_subtract
that can hold the memory addresses of functions that take two integer arguments and return an integer result.
We assign the addresses of the add
and subtract
functions to the function pointers using the &
(address-of) operator. We can then use the function pointers to call the functions using the (*function_pointer_name)(arguments)
syntax.
Rules to be followed when using functions in C:
- Declare the function before calling it: In C, it’s best to declare a function before calling it. This allows the compiler to check that the function is called with the correct number and types of arguments, and that the function returns the correct type of value.
- Use descriptive function names: Choose function names that describe what the function does. This makes it easier to understand the purpose of the function when reading the code.
- Limit the scope of functions: Functions should have a clear and limited purpose, and should not have side effects that affect the program state outside of the function. This makes the code easier to understand and debug.
- Use meaningful variable names: Choose variable names that describe the purpose of the variable. This makes the code easier to read and understand.
- Keep functions short and focused: Functions should be short and focused on a single task. This makes the code easier to understand and modify.
- Document the function: Include comments that describe what the function does, the arguments it takes, and the value it returns. This makes the code easier to read and maintain.