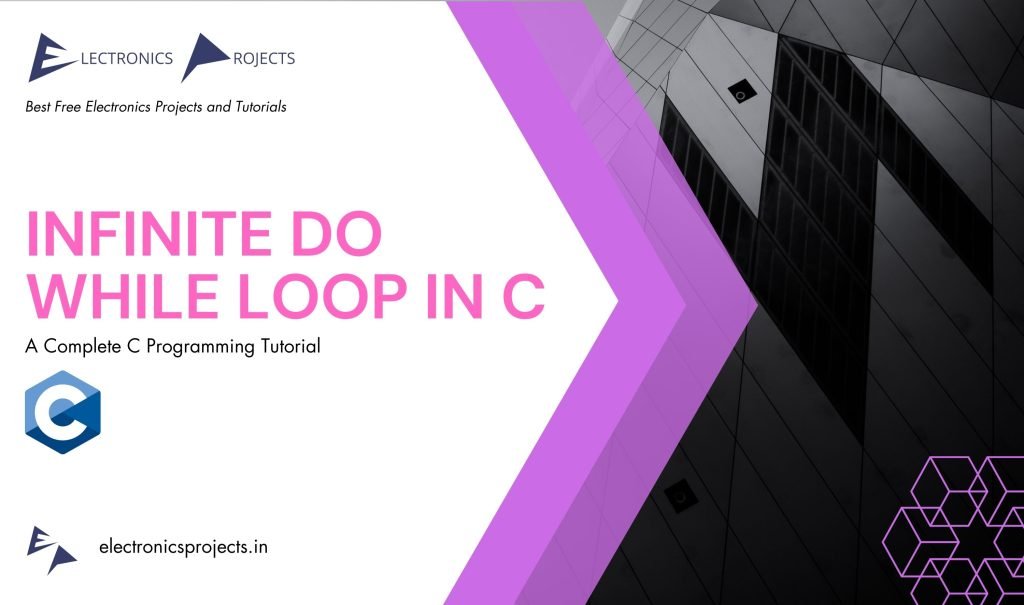
What is Infinite do while loop in C?
An infinite do while loop is a type of loop in the C programming language that runs indefinitely until it is explicitly terminated by a break or exit statement. It is called an infinite loop because it does not have a condition that will cause it to stop running on its own.
The infinite do while loop is used when we want a certain block of code to run continuously, such as when implementing a game loop or a program that listens for input from the user. The loop will continue running until we break out of it, either by using a break statement or by terminating the program.
Syntax:
do {
// block of code to be executed repeatedly
} while(1);
Here, the do
keyword starts the loop, followed by the code block that will be executed repeatedly. The while(1)
statement acts as a loop condition that will always evaluate to true, ensuring that the loop continues running indefinitely.
Examples of Infinite do while loop in C:
1. Printing infinite numbers for no reason
#include <stdio.h>
int main() {
int i = 0;
do {
printf("%d\n", i);
i++;
} while(1);
return 0;
}
Output:
0
1
2
3
4
5
...
Explanation:
In above example, the loop will continue running indefinitely, printing the value of i
and incrementing it by 1 with each iteration. Since there is no termination condition, the loop will continue running until the program is manually terminated.
2. Infinite User Input
#include <stdio.h>
int main() {
char c;
do {
printf("Enter a character: ");
scanf(" %c", &c);
printf("You entered '%c'\n", c);
} while(1);
return 0;
}
Output:
Enter a character: a
You entered 'a'
Enter a character: b
You entered 'b'
Enter a character: c
You entered 'c'
...
Explanation:
In above example, the loop prompts the user to enter a character, reads in the character using scanf()
, and then prints out the character that was entered. Since there is no termination condition, the loop will continue running until the program is manually terminated.
3. Sum of Numbers from User
#include <stdio.h>
int main() {
int num = 0, sum = 0;
do {
sum += num;
printf("Enter a number (enter a negative number to exit): ");
scanf("%d", &num);
} while(num >= 0);
printf("The sum of the numbers entered is %d\n", sum);
return 0;
}
Output:
Enter a number (enter a negative number to exit): 5
Enter a number (enter a negative number to exit): 10
Enter a number (enter a negative number to exit): -2
The sum of the numbers entered is 15
Explanation:
In above example, the loop prompts the user to enter a number, reads in the number using scanf()
, and then adds it to the running total sum
. If the user enters a negative number, the loop will terminate and the final sum will be printed. Otherwise, the loop will continue running indefinitely, prompting the user to enter more numbers.
4. Generating Random Numbers
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
int num;
srand(time(0));
do {
num = rand();
printf("%d\n", num);
} while(1);
return 0;
}
Output:
1727525946
890115539
892875636
838246135
1545910645
1848556186
593456181
1790203879
...
Explanation:
In above example, the program generates random integers using the rand()
function from the <stdlib.h>
library. The srand(time(0))
statement initializes the random number generator with the current time as the seed, so that the sequence of random numbers generated will be different each time the program is run.
The do-while loop runs indefinitely, since the loop condition 1
is always true. The loop generates a new random number in each iteration using the rand()
function, and prints it to the console using printf()
. The program will continue generating random numbers.
Rules to follow when using Infinite do while loop in C:
- Always include a way to break out of the loop: Since an infinite loop will continue running indefinitely, it is important to include a way to terminate the loop manually. This can be done using a break statement, or by terminating the program using an exit function.
- Be careful not to create an infinite loop unintentionally: Infinite loops can cause a program to crash or hang indefinitely, so it is important to be careful when designing loops to ensure that they terminate as expected.
- Ensure that any inputs to the loop are properly validated: Since an infinite loop will continue running indefinitely, it is important to validate any inputs to the loop to ensure that they are in the correct format and do not cause unexpected behavior.