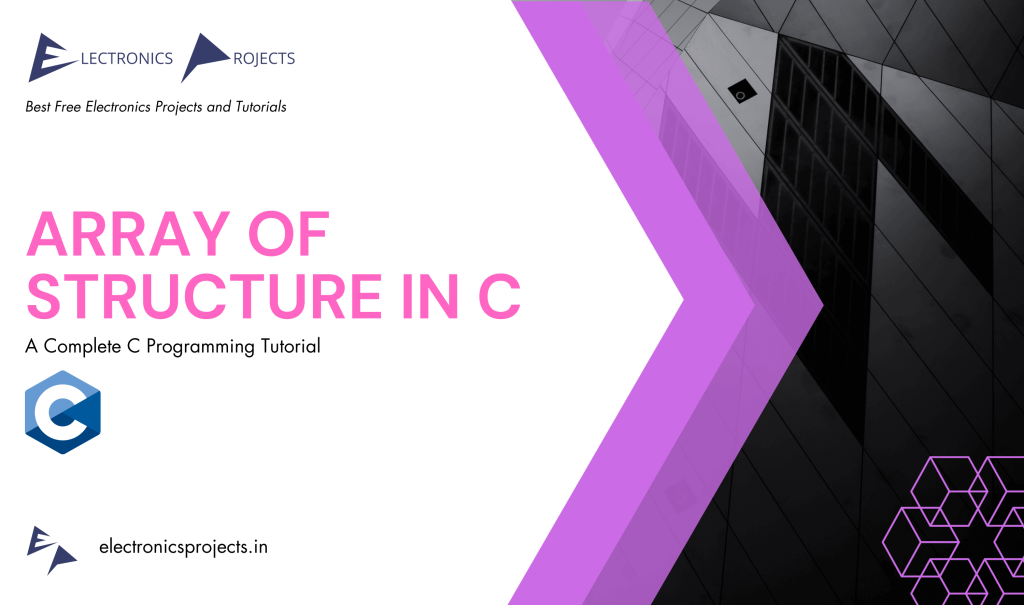
What is Array of Structure in C ?
In C, an array of structure is a data structure that allows you to store multiple instances of a structure in a contiguous block of memory. Each element in the array is a structure, and you can access individual elements using array indexing.
Array of structure is used in various scenarios to organize related data together. It provides a convenient way to represent and manipulate multiple objects of the same type, where each object consists of different fields or attributes.
Syntax:
struct StructureName {
// Define structure fields or members
dataType fieldName1;
dataType fieldName2;
// ...
};
// Declare an array of structures
struct StructureName arrayName[size];
// Access and modify individual elements of the array
arrayName[index].fieldName = value;
Explanation:
- First, define the structure using the keyword
struct
followed by the structure name (StructureName
in the example). Inside the structure, define the fields or members that make up the structure, each with its own data type and field name. - After defining the structure, declare an array of structures using the structure name and the desired array name (
arrayName
in the example). Specify the desired size of the array in square brackets ([size]
). - To access individual elements of the array of structures, use the array name followed by the index within square brackets (
arrayName[index]
). Then, access or modify the specific field of the structure using the dot operator (.
) followed by the field name (fieldName
in the example).
Examples of Array of Structure in C
Example 1: Storing Points.
#include <stdio.h>
struct Point {
int x;
int y;
};
int main() {
struct Point points[3];
points[0].x = 1;
points[0].y = 2;
points[1].x = 3;
points[1].y = 4;
points[2].x = 5;
points[2].y = 6;
for (int i = 0; i < 3; i++) {
printf("Point %d: (%d, %d)\n", i + 1, points[i].x, points[i].y);
}
return 0;
}
Output:
Point 1: (1, 2)
Point 2: (3, 4)
Point 3: (5, 6)
Explanation:
In above example, we define a structure called “Point” that has two integer fields, x
and y
. We then declare an array of three Point
structures. Each element of the array represents a point with its respective x and y coordinates. We initialize the values of the points and print them using a loop.
Example 2: Library Books Management.
#include <stdio.h>
#include <string.h>
// Define a structure for a book
struct Book {
char title[100];
char author[50];
int year;
};
int main() {
int numBooks;
// Prompt the user for the number of books in the library
printf("Enter the number of books in the library: ");
scanf("%d", &numBooks);
// Declare an array of Book structures
struct Book library[numBooks];
// Input book information
for (int i = 0; i < numBooks; i++) {
printf("\nEnter details for book %d:\n", i + 1);
// Clear the input buffer to prevent issues with fgets
while ((getchar()) != '\n');
printf("Title: ");
fgets(library[i].title, sizeof(library[i].title), stdin);
library[i].title[strcspn(library[i].title, "\n")] = '\0';
printf("Author: ");
fgets(library[i].author, sizeof(library[i].author), stdin);
library[i].author[strcspn(library[i].author, "\n")] = '\0';
printf("Publication Year: ");
scanf("%d", &library[i].year);
// Clear the input buffer after each input
while ((getchar()) != '\n');
}
// Display the library contents
printf("\nLibrary Contents:\n");
for (int i = 0; i < numBooks; i++) {
printf("Book %d:\n", i + 1);
printf("Title: %s\n", library[i].title);
printf("Author: %s\n", library[i].author);
printf("Publication Year: %d\n", library[i].year);
printf("\n");
}
return 0;
}
Output:
Enter the number of books in the library: 2
Enter details for book 1:
Title: The Great Gatsby
Author: F. Scott Fitzgerald
Publication Year: 1925
Enter details for book 2:
Title: To Kill a Mockingbird
Author: Harper Lee
Publication Year: 1960
Library Contents:
Book 1:
Title: The Great Gatsby
Author: F. Scott Fitzgerald
Publication Year: 1925
Book 2:
Title: To Kill a Mockingbird
Author: Harper Lee
Publication Year: 1960
Explanation:
In above example, we define a structure Book
to represent book information, including the title, author, and publication year. Users are prompted to input the number of books in the library, and then they can provide details for each book. The program uses fgets
to safely input strings and clears the input buffer as needed.
Finally, the program displays the contents of the library, including the details of each book.
Example 3: College Management System.
#include <stdio.h>
#define MAX_STUDENTS 100
struct Student {
char name[50];
int age;
float gpa;
};
struct College {
char collegeName[100];
struct Student students[MAX_STUDENTS];
int studentCount;
};
void addStudent(struct College* college, const char* name, int age, float gpa) {
if (college->studentCount < MAX_STUDENTS) {
struct Student newStudent;
snprintf(newStudent.name, sizeof(newStudent.name), "%s", name);
newStudent.age = age;
newStudent.gpa = gpa;
college->students[college->studentCount++] = newStudent;
} else {
printf("Maximum student capacity reached for college %s\n", college->collegeName);
}
}
void printCollegeDetails(const struct College* college) {
printf("College Name: %s\n", college->collegeName);
printf("Number of Students: %d\n", college->studentCount);
printf("Student Details:\n");
for (int i = 0; i < college->studentCount; i++) {
printf("Student %d: Name - %s, Age - %d, GPA - %.2f\n",
i + 1, college->students[i].name, college->students[i].age, college->students[i].gpa);
}
}
int main() {
struct College myCollege;
snprintf(myCollege.collegeName, sizeof(myCollege.collegeName), "ABC University");
myCollege.studentCount = 0;
addStudent(&myCollege, "John Doe", 20, 3.8);
addStudent(&myCollege, "Jane Smith", 21, 3.9);
addStudent(&myCollege, "Alice Johnson", 19, 3.7);
printCollegeDetails(&myCollege);
return 0;
}
Output:
College Name: ABC University
Number of Students: 3
Student Details:
Student 1: Name - John Doe, Age - 20, GPA - 3.80
Student 2: Name - Jane Smith, Age - 21, GPA - 3.90
Student 3: Name - Alice Johnson, Age - 19, GPA - 3.70
Explanation:
In above example, we define two structures: Student
and College
. The Student
structure represents individual student details, including name, age, and GPA. The College
structure contains the college name, an array of Student
structures, and a student count to keep track of the number of students.
We provide functions addStudent
and printCollegeDetails
to add students to a college and display the college’s information, respectively. The addStudent
function takes a pointer to the College
structure, as well as the student’s name, age, and GPA. It adds the new student to the array of students in the college structure, as long as the maximum capacity hasn’t been reached.
In the main
function, we create a College
structure and add three students using the addStudent
function. Finally, we print the college details using the printCollegeDetails
function.