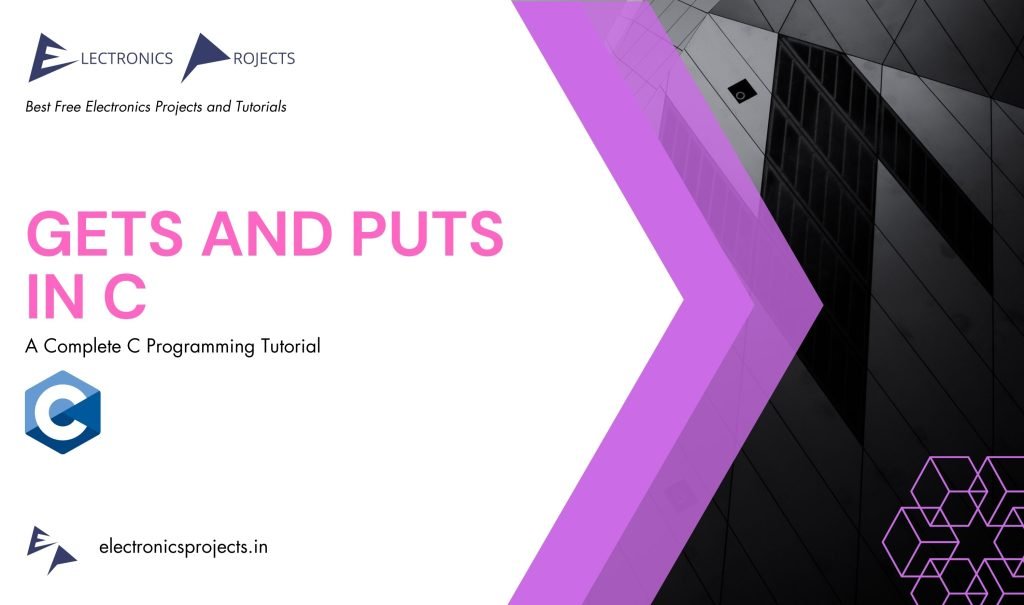
What is gets and puts in C ?
The gets()
and puts()
functions are I/O functions in the C programming language.
1. gets()
The gets()
function is used to read a string from the standard input (usually the keyboard) and store it in an array of characters.
char* gets(char* str);
The gets()
function takes a single argument, str
, which is a pointer to an array of characters where the input string will be stored. It reads characters from the standard input until a newline character ('\n'
) is encountered. It then adds a null character ('\0'
) to mark the end of the string. However, it is important to note that gets()
is considered unsafe to use due to its inability to handle buffer overflow, so it is generally recommended to use safer alternatives like fgets()
.
2. puts()
The puts()
function is used to write a string to the standard output (usually the console).
int puts(const char* str);
The puts()
function takes a single argument, str
, which is a pointer to a null-terminated string. It writes the string followed by a newline character ('\n'
) to the standard output. It returns a non-negative value on success and a negative value on failure.
Examples
Examples 1:
#include <stdio.h>
int main() {
char name[50];
printf("Enter your name: ");
gets(name);
printf("Hello, ");
puts(name);
return 0;
}
Output:
Enter your name: JUDY
Hello, JUDY
Explanation:
In above example, the user is prompted to enter their name. The gets()
function reads the input string from the user and stores it in the name
array. Then, the puts()
function prints the name with a greeting.
Examples 2:
#include <stdio.h>
int main() {
char sentence[100];
printf("Enter a sentence: ");
gets(sentence);
printf("You entered: ");
puts(sentence);
return 0;
}
Output:
Enter a sentence: Hello there chooms!!!
You entered: Hello there chooms!!!
Explanation:
In above example, the user is asked to enter a sentence. The gets()
function reads the sentence and stores it in the sentence
array. The puts()
function then prints the entered sentence.
Examples 3:
Now lets see some complex example of gets()
and puts()
that reverses a sentence:
#include <stdio.h>
#include <string.h>
void reverseSentence(char* sentence) {
char* token;
char* words[100];
int wordCount = 0;
// Tokenize the sentence into individual words
token = strtok(sentence, " ");
while (token != NULL) {
words[wordCount++] = token;
token = strtok(NULL, " ");
}
// Reverse the order of the words
for (int i = wordCount - 1; i >= 0; i--) {
puts(words[i]);
}
}
int main() {
char sentence[100];
printf("Enter a sentence: ");
gets(sentence);
printf("Reversed sentence:\n");
reverseSentence(sentence);
return 0;
}
Output:
Enter a sentence: Pikachu I choose you!!!
Reversed sentence:
you!!!
choose
I
Pikachu
Explanation:
In above example, the user is prompted to enter a sentence. The gets()
function is used to read the sentence and store it in the sentence
array.
The reverseSentence()
function takes the entered sentence as input. It uses the strtok()
function from the string.h
library to tokenize the sentence into individual words based on the space delimiter. The words are then stored in an array called words[]
in reverse order.
Finally, in the main()
function, the reversed sentence is printed using the puts()
function. Each word is printed on a separate line.