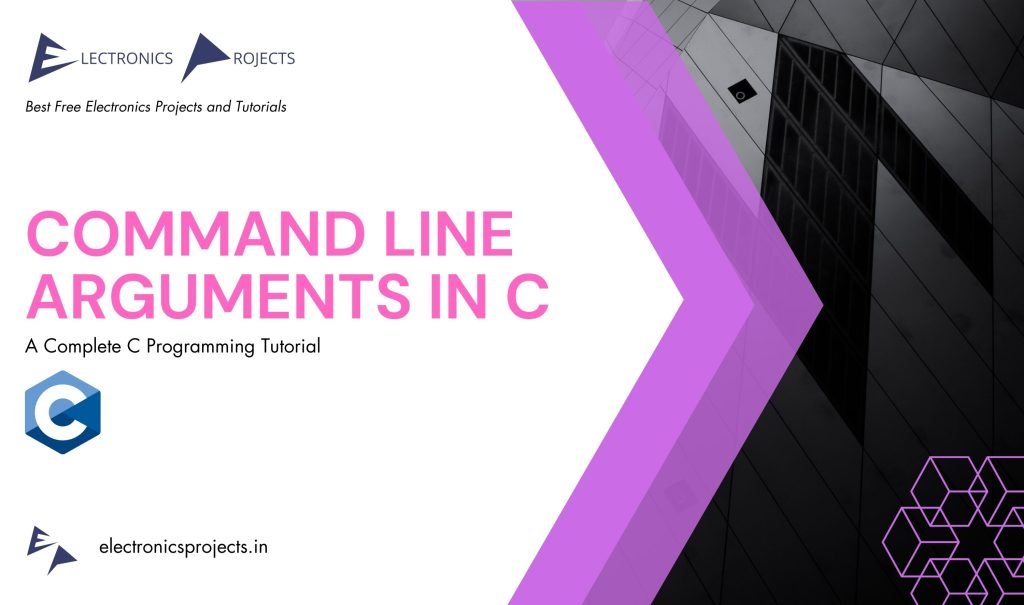
What is Command Line Arguments in C ?
Command line arguments in C are parameters passed to a C program when it is executed from the command line or terminal. These arguments provide a way to input data or configuration settings to the program at runtime. They are stored in the main
function’s parameter list and can be accessed to control the behavior of the program.
The main
function in C can be defined as follows:
int main(int argc, char *argv[])
Here, argc
(argument count) represents the number of command line arguments, and argv
(argument vector) is an array of strings, where each element is a command line argument. argv[0]
typically contains the name of the program itself.
Common uses of command line arguments in C include providing input data files, specifying configuration settings, and controlling program behavior.
There are mainly two types of command line arguments in C:
- Named (Flag) Arguments: These are arguments that are preceded by a specific flag or keyword to indicate their purpose. They are usually optional, and their presence or absence alters the program’s behavior.
- Positional Arguments: These are arguments that don’t have a flag or keyword and are based on their position in the command line. They are typically used for required inputs.
1. Positional Arguments
These are based on their position in the command line.
#include <stdio.h>
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s <arg1> <arg2>\n", argv[0]);
return 1;
}
int arg1 = atoi(argv[1]);
int arg2 = atoi(argv[2]);
printf("Sum of %d and %d is %d\n", arg1, arg2, arg1 + arg2);
return 0;
}
Output:
$ ./myprogram 5 7
Sum of 5 and 7 is 12
Explanation:
In above example, the program takes two positional arguments from the command line, arg1
and arg2
. These arguments are expected to be integers. Here’s the breakdown:
int arg1 = atoi(argv[1]);
extracts the first positional argument (argv[1]) and converts it to an integer.int arg2 = atoi(argv[2]);
extracts the second positional argument (argv[2]) and converts it to an integer.printf("Sum of %d and %d is %d\n", arg1, arg2, arg1 + arg2);
calculates and prints the sum of the two integers.
When you run the program with ./myprogram 5 7
, it takes the values 5 and 7 as positional arguments, calculates their sum (12), and displays the result as “Sum of 5 and 7 is 12.”
2. Named (Flag) Arguments
#include <stdio.h>
#include <string.h>
int main(int argc, char *argv[]) {
int verbose = 0; // Default is not verbose
for (int i = 1; i < argc; i++) {
if (strcmp(argv[i], "-v") == 0 || strcmp(argv[i], "--verbose") == 0) {
verbose = 1;
}
}
if (verbose) {
printf("Verbose mode enabled.\n");
} else {
printf("Verbose mode is disabled.\n");
}
return 0;
}
Output:
$ ./myprogram -v
Verbose mode enabled.
$ ./myprogram
Verbose mode is disabled.
Explanation:
In this example, the program checks for the presence of a named argument -v
or --verbose
in the command line. If either of these arguments is provided, it sets the verbose
flag to 1, indicating that verbose mode should be enabled. Here’s the breakdown:
- The
for
loop iterates through each command line argument starting fromargv[1]
(sinceargv[0]
contains the program name). - It checks each argument using
strcmp
to see if it matches either-v
or--verbose
. - If a match is found, the
verbose
flag is set to 1.
When you run the program with ./myprogram -v
, it detects the -v
flag and sets verbose
to 1, resulting in the output “Verbose mode enabled.” If you run it without the flag, as in ./myprogram
, verbose
remains 0, and the output is “Verbose mode is disabled.”