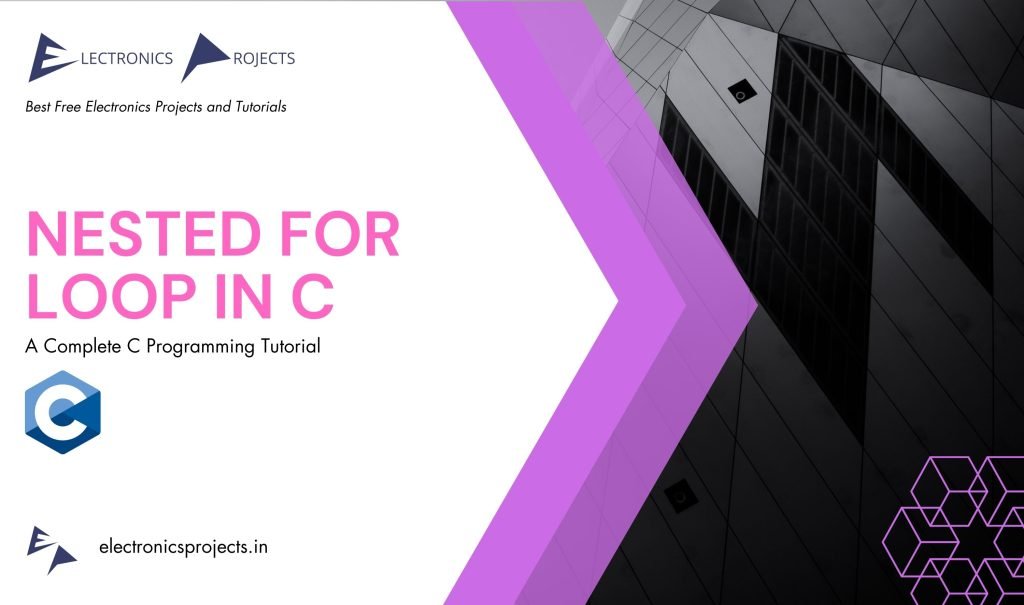
What is Nested for loop in C?
A nested for loop is a loop inside another loop in the C programming language. It is a way to execute a set of statements repeatedly for each element in one or more arrays or collections.
The primary use of nested loops is to access multidimensional arrays, matrices, and other complex data structures. They can also be used to perform computations on multiple levels or to traverse a data structure with nested levels.
Syntax:
for (initialization_expression_1; condition_expression_1; increment_expression_1)
{
for (initialization_expression_2; condition_expression_2; increment_expression_2)
{
/* statements */
}
}
Examples of Nested for loop:
1. Print Multiplication Table using Nested for loop:
#include <stdio.h>
int main()
{
int i, j;
for(i = 1; i <= 10; i++)
{
for(j = 1; j <= 10; j++)
{
printf("%d x %d = %d\n", i, j, i*j);
}
printf("\n");
}
return 0;
}
Output:
1 x 1 = 1
1 x 2 = 2
1 x 3 = 3
1 x 4 = 4
1 x 5 = 5
1 x 6 = 6
1 x 7 = 7
1 x 8 = 8
1 x 9 = 9
1 x 10 = 10
2 x 1 = 2
2 x 2 = 4
2 x 3 = 6
2 x 4 = 8
2 x 5 = 10
2 x 6 = 12
2 x 7 = 14
2 x 8 = 16
2 x 9 = 18
2 x 10 = 20
3 x 1 = 3
3 x 2 = 6
3 x 3 = 9
3 x 4 = 12
3 x 5 = 15
3 x 6 = 18
3 x 7 = 21
3 x 8 = 24
3 x 9 = 27
3 x 10 = 30
4 x 1 = 4
4 x 2 = 8
4 x 3 = 12
4 x 4 = 16
4 x 5 = 20
4 x 6 = 24
4 x 7 = 28
4 x 8 = 32
4 x 9 = 36
4 x 10 = 40
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
6 x 1 = 6
6 x 2 = 12
6 x 3 = 18
6 x 4 = 24
6 x 5 = 30
6 x 6 = 36
6 x 7 = 42
6 x 8 = 48
6 x 9 = 54
6 x 10 = 60
7 x 1 = 7
7 x 2 = 14
7 x 3 = 21
7 x 4 = 28
7 x 5 = 35
7 x 6 = 42
7 x 7 = 49
7 x 8 = 56
7 x 9 = 63
7 x 10 = 70
8 x 1 = 8
8 x 2 = 16
8 x 3 = 24
8 x 4 = 32
8 x 5 = 40
8 x 6 = 48
8 x 7 = 56
8 x 8 = 64
8 x 9 = 72
8 x 10 = 80
9 x 1 = 9
9 x 2 = 18
9 x 3 = 27
9 x 4 = 36
9 x 5 = 45
9 x 6 = 54
9 x 7 = 63
9 x 8 = 72
9 x 9 = 81
9 x 10 = 90
10 x 1 = 10
10 x 2 = 20
10 x 3 = 30
10 x 4 = 40
10 x 5 = 50
10 x 6 = 60
10 x 7 = 70
10 x 8 = 80
10 x 9 = 90
10 x 10 = 100
Explanation:
In above example, we are using nested for loops to print the multiplication table from 1 to 10. The outer loop iterates from 1 to 10 and the inner loop iterates from 1 to 10 for each iteration of the outer loop. The printf statement inside the inner loop prints the multiplication table.
2. Find the sum of all elements in a 2D array using nested for loop:
#include <stdio.h>
int main()
{
int arr[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
int i, j, sum = 0;
for(i = 0; i < 3; i++)
{
for(j = 0; j < 3; j++)
{
sum += arr[i][j];
}
}
printf("Sum of all elements in the array: %d\n", sum);
return 0;
}
Output:
Sum of all elements in the array: 45
Explanation:
In above example, we are using nested for loops to find the sum of all elements in a 2D array. The outer loop iterates over the rows of the array, and the inner loop iterates over the columns of the array. The sum variable is initialized to 0 and is incremented by each element in the array.
3. Print a pattern using nested for loop:
#include <stdio.h>
int main()
{
int i, j, k;
for(i = 1; i <= 5; i++)
{
for(j = 1; j <= 5-i; j++)
{
printf(" ");
}
for(k = 1; k <= 2*i-1; k++)
{
printf("*");
}
printf("\n");
}
return 0;
}
Output:
*
***
*****
*******
*********
Explanation:
In above example, we are using nested for loops to print a pattern of stars in a pyramid shape. The outer loop iterates from 1 to 5 for the number of rows. The first inner loop is used to print spaces before the stars, and the second inner loop is used to print the stars. The number of spaces and stars to be printed is calculated using the value of the outer loop variable.
Rules to follow while using nested for loops:
- The number of loops should be kept to a minimum to avoid excessive computational time.
- The inner loop should be dependent on the outer loop.
- The initialization, condition, and increment/decrement statements of each loop should be separated by a semicolon.
- It is important to properly format the loops to make the code more readable and easier to understand.
Modifications that can be made while using nested for loops:
- The number of loops can be increased or decreased based on the complexity of the program.
- The loops can be nested within if statements, while loops, or do-while loops to add more functionality to the program.
- The increment/decrement statements of the inner loop can be used to modify the condition of the outer loop, allowing for more complex conditions.
- The loops can be used with break and continue statements to control the flow of the program.