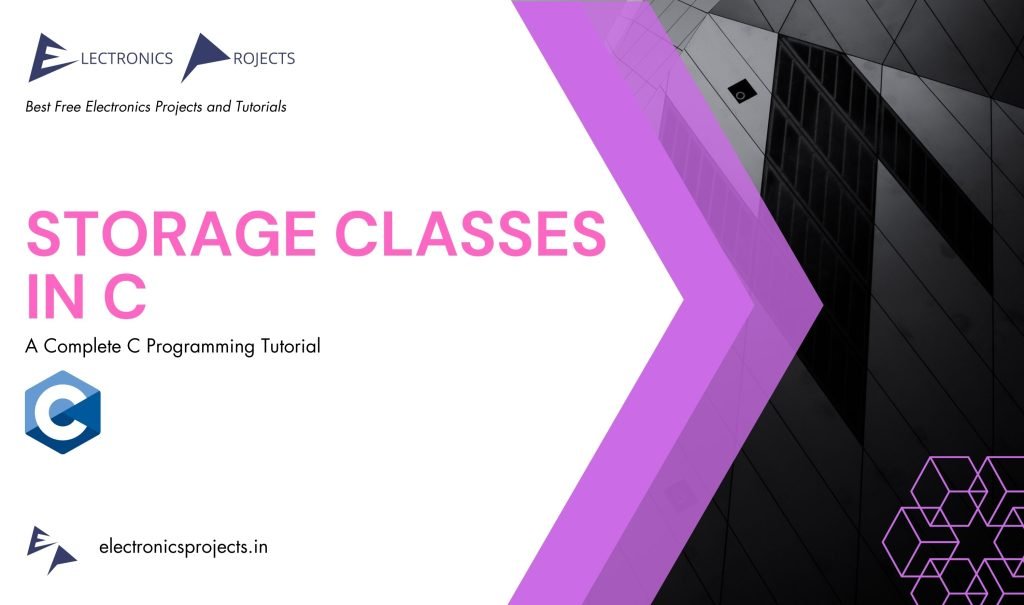
What are Storage Classes in C?
Storage classes in C define the scope, lifetime, and storage location of variables and functions. They also specify the initial value of a variable if it is not explicitly initialized.
Types of Storage Classes in C:
- Automatic: This is the default storage class for all local variables declared inside a function or block. The variable is created when the function or block is entered and destroyed when the function or block is exited. The storage location for an automatic variable is the stack. The initial value of an automatic variable is undefined.
- Register: This storage class is used to declare local variables that should be stored in a register instead of memory. This is only a suggestion to the compiler, and the compiler may choose to ignore it. Register variables are also automatically initialized to an undefined value.
- Static: Variables declared with the static storage class have a lifetime throughout the entire program execution. The storage location for static variables is in the data segment of memory. The initial value of a static variable is zero by default.
- Extern: This storage class is used to declare variables or functions that are defined in another file. The storage location for an extern variable is in the memory location of the file that defines it. The initial value of an extern variable is also zero by default.
Sr. No. | Storage Class | Storage Location | Value | Scope | Lifetime | Information |
---|---|---|---|---|---|---|
1 | Automatic | Stack | Undefined | Block | Function Call | Default Storage Class for Local Variables. |
2 | Register | Register | Undefined | Block | Function Call | Suggests the compiler to store the variable in a register for faster access. |
3 | Static | Data Segment | 0 | File | Program Execution | Retain it’s value between Function/Block calls. |
4 | Extern | Memory location of the file that defines it | 0 | File | Program Execution | Used to declare variables or functions that are defined in another file. Links to the memory location where it is defined. |
Automatic
This is the default storage class for all local variables declared inside a function or block. The variable is created when the function or block is entered and destroyed when the function or block is exited. The storage location for an automatic variable is the stack. The initial value of an automatic variable is undefined.
Example:
#include <stdio.h>
void function() {
int x = 10; // Automatic variable
printf("x inside function: %d\n", x);
}
int main() {
int x = 5; // Automatic variable
printf("x inside main: %d\n", x);
function();
return 0;
}
Output:
x inside main: 5
x inside function: 10
Explanation:
In above example, the variable x
is declared as an automatic variable in both main()
and function()
. Each function has its own separate instance of x
, and the value of x
inside each function is independent of the other.
Register
This storage class is used to declare local variables that should be stored in a register instead of memory. This is only a suggestion to the compiler, and the compiler may choose to ignore it. Register variables are also automatically initialized to an undefined value.
Example:
#include <stdio.h>
int main() {
register int x = 5; // Register variable
printf("x: %d\n", x);
return 0;
}
Output:
x: 5
Explanation:
In above example, the variable x
is declared as a register variable. The register
keyword suggests to the compiler that x
should be stored in a CPU register for faster access. However, the compiler may choose to ignore this suggestion.
Static
Variables declared with the static storage class have a lifetime throughout the entire program execution. The storage location for static variables is in the data segment of memory. The initial value of a static variable is zero by default.
Example:
#include <stdio.h>
void function() {
static int x = 0; // Static variable
printf("x: %d\n", x);
x++;
}
int main() {
function(); // Output: x: 0
function(); // Output: x: 1
function(); // Output: x: 2
return 0;
}
Output:
x: 0
x: 1
x: 2
Explanation:
In above example, the variable x
is declared as a static variable inside the function()
function. The static variable retains its value between function calls, so each time function()
is called, the value of x
is incremented by 1.
Extern
This storage class is used to declare variables or functions that are defined in another file. The storage location for an extern variable is in the memory location of the file that defines it. The initial value of an extern variable is also zero by default.
Example:
File1.c
#include <stdio.h>
int count; // Extern variable
void increment() {
count++;
}
void print() {
printf("count: %d\n", count);
}
File2.c
#include <stdio.h>
extern int count; // Extern variable
int main() {
count = 10;
increment();
print();
return 0;
}
Output:
count: 11
Explanation:
In this example, the variable count
is declared as an extern variable in File1.c
. It is then used in File2.c
, where it is assigned a value of 10. The increment()
function increments the value of count
by 1, and the print()
function prints the value of count
, which is 11.
Remember to include the necessary header files and compile and link the code appropriately when working with multiple files.
Rules to follow while using Storage Classes in C:
- Each variable must have only one storage class.
- Automatic variables are created and destroyed when their scope is entered and exited, respectively.
- Register variables may or may not be stored in a register, depending on the compiler’s discretion.
- Static variables retain their value between function calls and have a lifetime throughout the program’s execution.
- Extern variables must be defined in a separate file, and they link to the memory location where they are defined.