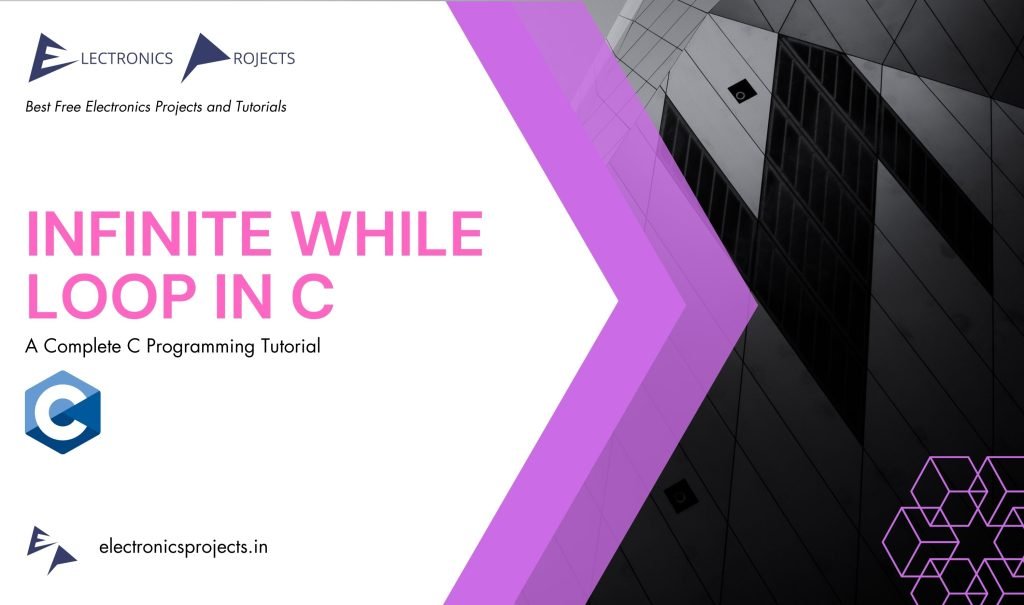
What is Infinite while loop in C?
An infinite while loop is a loop in C programming language that runs continuously without an exit condition, which means that the loop will keep executing until the program is terminated or an interrupt is called. An infinite while loop can be created by omitting the loop termination condition in the while statement.
The primary use of an infinite while loop is to create a continuous loop for programs that require constant monitoring or updating, such as real-time systems or server programs.
Syntax:
while(1)
{
// code to be executed repeatedly
}
Here, the condition “1” is always true, which leads to an infinite loop.
Example of Infinite while loop in C:
1. Infinite Bruh!
#include <stdio.h>
int main()
{
while(1)
{
printf("Bruh!\n");
}
return 0;
}
Output:
Bruh!
Bruh!
Bruh!
...
Explanation:
The above example keeps printing “Bruh!” indefinitely.
2. Printing Infinite Numbers
#include <stdio.h>
int main()
{
int i = 0;
while (i >= 0)
{
printf("%d\n", i);
i++;
}
return 0;
}
Output:
0
1
2
...
2147483647
-2147483648
-2147483647
...
Explanation:
The above example, the program will keep printing integer values in ascending order until it reaches the maximum value of an integer data type (2147483647) and then start printing negative integer values in descending order.
3. Reading infinite number of inputs from the user
#include <stdio.h>
int main()
{
int num;
while(1)
{
printf("Enter a number: ");
scanf("%d", &num);
printf("The number you entered is: %d\n", num);
}
return 0;
}
Output:
Enter a number: 10
The number you entered is: 10
Enter a number: 20
The number you entered is: 20
Enter a number: 30
The number you entered is: 30
...
The above example, the program reads a number from the user in each iteration of the infinite loop and prints it back.
Rules to follow when using infinite while loop in C:
- Always include an interrupt mechanism to break the loop when necessary.
- Ensure that the loop does not consume too much system resources.
- Avoid using an infinite loop for programs that require user input.
- Use an infinite loop only when it is necessary and appropriate.