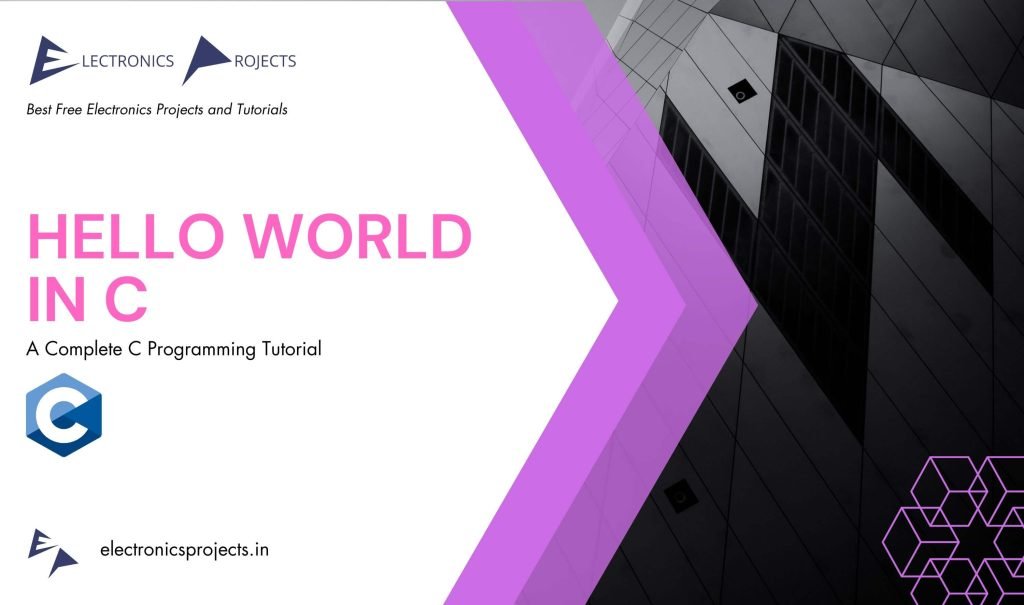
Hello World in C Programming Language:
“Hello, World!” is a common phrase used in computer programming tutorials and examples to demonstrate the basic syntax of a programming language. The “Hello, World!” program is a very simple program that just prints this phrase to the console or terminal window, without doing any real computation or processing.
In C programming, the “Hello, World!” program is typically written using the printf()
function to print the text to the console. The program is very short and easy to understand, which makes it a good starting point for learning the language.
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Output of above code:
Hello, World!
This text would be printed to the console or terminal window when you run the program. The program doesn’t have any other output, so this would be the only thing you see.
Explanation of above code:
Let’s go through each line of the program and explain what it does:
#include <stdio.h>
: This line is a preprocessor directive that tells the compiler to include the standard input/output library, which contains theprintf()
function we use later in the program.int main() {
: This line starts themain()
function, which is the entry point of the program. Theint
beforemain()
specifies the return type of the function, which in this case is an integer. The empty parentheses indicate thatmain()
takes no arguments.printf("Hello, World!\n");
: This line uses theprintf()
function to print the string “Hello, World!” to the console. The\n
at the end of the string represents a newline character, which adds a line break after the text is printed.return 0;
: This line ends themain()
function and returns the integer value0
. In C, returning0
frommain()
indicates that the program executed successfully.
That’s it! When you compile and run this program, you should see the text “Hello, World!” printed to the console.