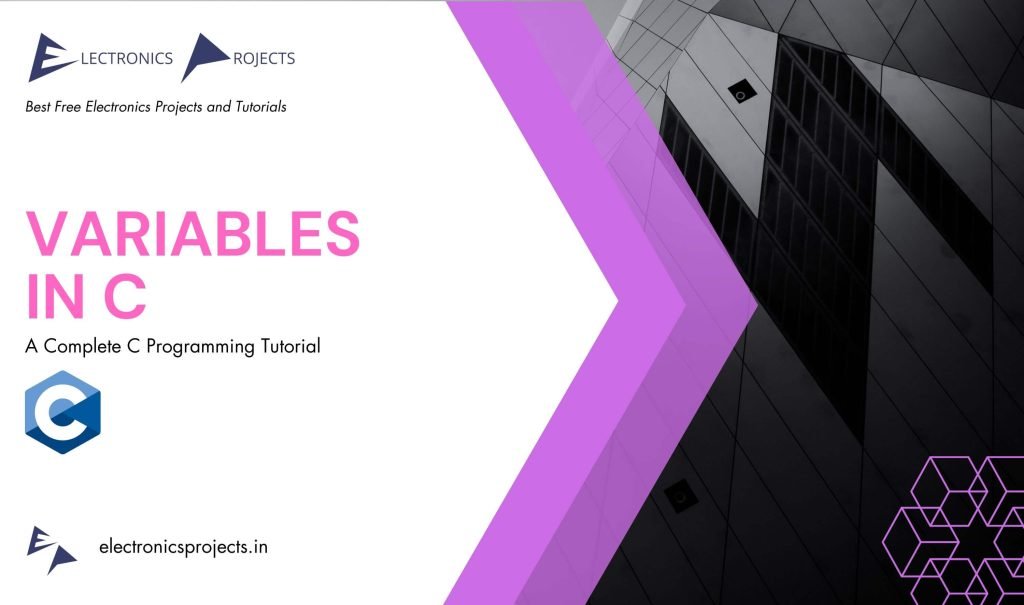
What are variables in C?
Variables are fundamental entities in C programming language that represent a reserved memory location to store a value or data. Variables are a primary tool for data manipulation and storage in computer programming.
Variables are used to store data values that are subject to change or modification throughout the program’s execution. By assigning a name or identifier to a memory location, the program can easily access and manipulate the value stored in that location using the identifier.
Data Types | Meaning | Data Types with Variables |
---|---|---|
int | Integer | int age; |
char | Character | char grade; |
float | Floating Point | float pi; |
double | Double-precision floating-point | double price; |
long | Long Integer | long population; |
short | Short Integer | short temp; |
unsigned int | Unsigned Integer | unsigned int score; |
signed int | Signed Integer | signed int balance; |
void | void | void result(); |
In above table, in Examples column, the age, grade, pi, price, population, temp, score and balance are all variables. You can also assign values to variables just like given below:
Variables with values |
---|
int age = 21; |
char grade = ‘A’; |
float pi = 3.14; |
double price = 420 |
long population = 1000; |
short temp = 3; |
unsigned int score = 69; |
signed int balance = 100; |
Rules while declaring a Variable in C:
- Variable names can contain letters, digits, and underscores.
- Variable names cannot start with a digit.
- Variable names are case sensitive.
- Variable names should be descriptive and meaningful.
- Variables should be declared before they are used.
- Variables should be initialized with a value.
Syntax:
data_type variable_name = value;
Types of Variables in C:
- 1. Local Variable.
- 2. Global Variable.
- 3. Static Variable.
- 4. Automatic Variable.
- 5. External Variable.
1. Local Variable
A local variable is a variable that is declared inside a function or block of code. It can only be accessed within that function or block of code.
Syntax:
data_type variable_name = value;
Example of Local Variable in C:
#include <stdio.h>
void foo()
{
int x = 10;
printf("x = %d\n", x);
}
int main()
{
foo();
return 0;
}
Output:
x = 10
Explanation:
In this example, we declare a function called “foo” that contains a local variable “x”. We initialize “x” with the value 10 and print it to the console. When we call the “foo” function from the “main” function, the value of “x” is printed to the console.
We use local variables when we need to store temporary data within a function or block of code.
2. Global Variable
A global variable is a variable that is declared outside of any function or block of code. It can be accessed from any part of the program.
Syntax:
data_type variable_name = value;
Example of Global Variable in C:
#include <stdio.h>
int x = 10;
void foo()
{
printf("x = %d\n", x);
}
int main()
{
foo();
return 0;
}
Output:
x = 10
Explanation:
In this example, we declare a global variable “x” outside of any function. We initialize it with the value 10 and print it to the console within the “foo” function. When we call the “foo” function from the “main” function, the value of “x” is printed to the console.
We use global variables when we need to store data that is used across multiple functions or blocks of code.
3. Static Variable
A static variable is a variable that is declared inside a function or block of code with the “static” keyword. It retains its value between function calls.
Syntax:
static data_type variable_name = value;
Example of Static Variable in C:
#include <stdio.h>
void foo()
{
static int x = 0;
x++;
printf("x = %d\n", x);
}
int main()
{
foo();
foo();
foo();
return 0;
}
Output:
x = 1
x = 2
x = 3
Explanation:
In this example, we declare a function called “foo” that contains a static variable “x”. We initialize “x” with the value 0 and increment it each time the function is called. When we call the “foo” function three times from the “main” function, the value of “x” is printed to the console each time, and we can see that it retains its value between function calls.
We use static variables when we need to store data that retains its value between function calls.
4. Automatic Variable
An automatic variable is a variable that is declared inside a function or block of code without the “static” keyword. It is destroyed when the function or block of code ends.
Syntax:
data_type variable_name = value;
Example of Automatic Variable in C:
#include <stdio.h>
void foo()
{
int x = 10;
printf("x = %d\n", x);
}
int main()
{
foo();
return 0;
}
Output:
x = 10
Explanation:
In this example, we declare a function called “foo” that contains an automatic variable “x”. We initialize “x” with the value 10 and print it to the console. When the “foo” function ends, the value of “x” is destroyed.
We use automatic variables when we need to store temporary data within a function or block of code that does not need to be retained between function calls.
5. External Variable
An external variable is a variable that is declared outside of any function or block of code with the “extern” keyword. It can be accessed from any part of the program.
Syntax:
extern data_type variable_name;
Example of External Variable in C:
#include <stdio.h>
extern int x;
void foo()
{
printf("x = %d\n", x);
}
int main()
{
x = 10;
foo();
return 0;
}
Output:
x = 10
In this example, we declare an external variable “x” outside of any function using the “extern” keyword. We then define it and initialize it with the value 10 within the “main” function. We then call the “foo” function, which prints the value of “x” to the console.
We use external variables when we need to store data that is used across multiple functions or blocks of code that are in different source files.
Modifications that can be made while declaring C:
1. Static
Static: When a variable is declared as static, it retains its value between function calls. The scope of a static variable is local to the function in which it is declared, but its lifetime is throughout the program.
Syntax:
static int count = 0;
2. Extern
When a variable is declared as extern, it is declared in another file, but its memory is allocated in the current file. The extern keyword is used to declare a global variable that is defined in another source file.
Syntax:
extern int count;
3. Register
When a variable is declared as register, it suggests the compiler to store the variable in a CPU register instead of memory. Register variables are faster than memory variables, but their size is limited, and they can’t have an address.
Syntax:
register int i;
4. Volatile
When a variable is declared as volatile, it tells the compiler that the variable can be changed by external factors, so the compiler shouldn’t optimize it. The volatile keyword is used to force the compiler to reload the value of the variable from memory every time it is used, to avoid any optimizations.
Syntax:
volatile int status;
5. Const
When a variable is declared as const, its value can’t be changed once it is initialized. The const keyword is used to define a read-only variable.
Syntax:
const int MAX_VALUE = 100;