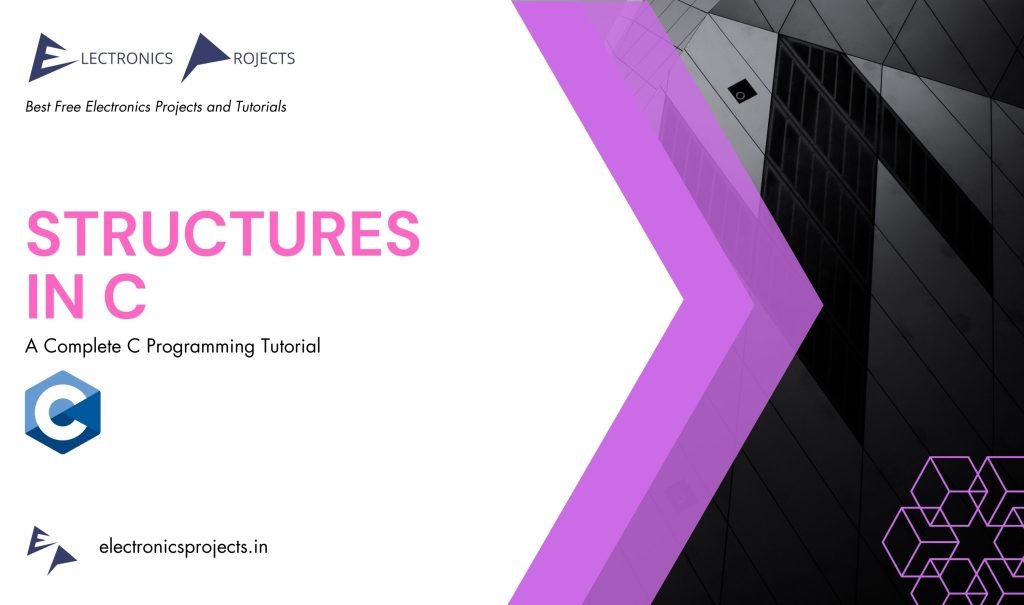
What is Structure in C ?
In C, a structure is a user-defined data type that allows you to combine different types of variables under a single name. It is used to group related data items together. Structures provide a convenient way to represent complex data structures such as records, objects, or entities.
To declare a structure in C, you use the struct
keyword followed by the structure name and a set of braces {}
that contain the members of the structure. Each member is declared with its data type and name.
Here’s an example of a simple structure declaration in C:
struct Person {
char name[50];
int age;
float height;
};
In the above example, we declared a structure named Person
with three members: name
of type character array, age
of type integer, and height
of type float.
To access the members of a structure, you use the dot .
operator. Here’s an example:
struct Person john;
strcpy(john.name, "John Doe");
john.age = 25;
john.height = 1.75;
In the above code, we declared a structure variable named john
of type Person
. We can access the members of the structure using the dot operator and assign values to them.
Types of Structures in C:
1. Structure without a tag:
struct {
int x;
int y;
} point;
2. Structure with a tag:
struct Point {
int x;
int y;
};
struct Point point;
3. Structure with typedef:
typedef struct {
int x;
int y;
} Point;
Point point;
Examples of Structure in C
1. Person Information
#include <stdio.h>
struct Person {
char name[50];
int age;
};
int main() {
struct Person john;
strcpy(john.name, "John Doe");
john.age = 25;
printf("Name: %s\n", john.name);
printf("Age: %d\n", john.age);
return 0;
}
Output:
Name: John Doe
Age: 25
Explanation:
In above example, we define a simple structure named Person
with two members: name
(a character array to store a person’s name) and age
(an integer to store the person’s age). In the main
function, we create an instance of this structure named john
. We then use strcpy
to copy the name “John Doe” into john.name
and set john.age
to 25. Finally, we print out the values of john.name
and john.age
.
2. Rectangle Area Calculation
#include <stdio.h>
struct Rectangle {
int width;
int height;
};
int area(struct Rectangle rect) {
return rect.width * rect.height;
}
int main() {
struct Rectangle rect;
rect.width = 5;
rect.height = 10;
int rectArea = area(rect);
printf("Rectangle Area: %d\n", rectArea);
return 0;
}
Output:
Rectangle Area: 50
Explanation:
In above example, we define a structure named Rectangle
with two members: width
and height
, both of type integer. We then declare a function area
that takes a Rectangle
structure as a parameter and calculates the area of the rectangle. In the main
function, we create an instance of the Rectangle
structure named rect
, set its width
and height
, and then calculate and print its area.
3. Employee Database Management
#include <stdio.h>
struct Employee {
char name[50];
int age;
float salary;
};
void displayEmployee(struct Employee emp) {
printf("Name: %s\n", emp.name);
printf("Age: %d\n", emp.age);
printf("Salary: %.2f\n", emp.salary);
}
int main() {
struct Employee employees[3];
for (int i = 0; i < 3; i++) {
printf("Enter details of Employee %d:\n", i + 1);
printf("Name: ");
scanf("%s", employees[i].name);
printf("Age: ");
scanf("%d", &employees[i].age);
printf("Salary: ");
scanf("%f", &employees[i].salary);
}
printf("\nEmployee Details:\n");
for (int i = 0; i < 3; i++) {
displayEmployee(employees[i]);
printf("--------------------\n");
}
return 0;
}
Output:
Enter details of Employee 1:
Name: John
Age: 30
Salary: 50000
Enter details of Employee 2:
Name: Alice
Age: 28
Salary: 45000
Enter details of Employee 3:
Name: Bob
Age: 35
Salary: 60000
Employee Details:
Name: John
Age: 30
Salary: 50000.00
--------------------
Name: Alice
Age: 28
Salary: 45000.00
--------------------
Name: Bob
Age: 35
Salary: 60000.00
--------------------
Explanation:
In above example, we define a structure named Employee
with three members: name
(a character array to store the employee’s name), age
(an integer to store the employee’s age), and salary
(a float to store the employee’s salary). We also define a function displayEmployee
that takes an Employee
structure as a parameter and displays its details.
In the main
function, we create an array of Employee
structures named employees
to store information about three employees. We use a loop to input the details of each employee (name, age, and salary) and then display their details using the displayEmployee
function.