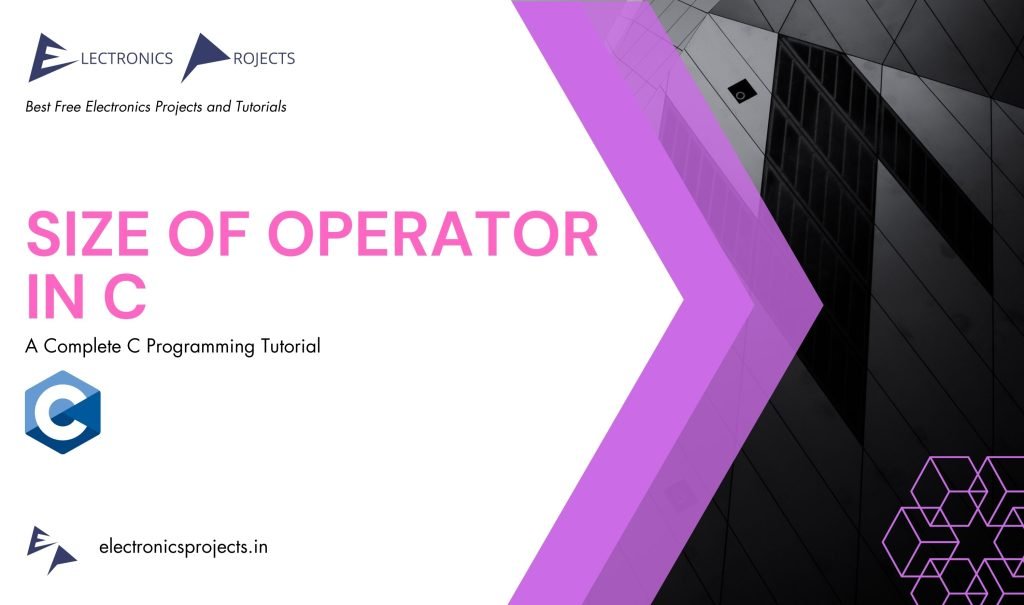
What is sizeof in C ?
The sizeof()
operator in C is used to determine the size, in bytes, of a datatype or a variable. It returns the size as an unsigned integer value, which represents the number of bytes occupied in memory by the operand.
The sizeof()
operator is useful in various scenarios, including:
- Memory allocation: It helps in allocating the appropriate amount of memory dynamically, based on the size of a datatype or structure.
- Buffer manipulation: It allows you to calculate the size of a buffer to ensure you don’t overrun its boundaries while performing operations like copying or reading data.
Examples of sizeof in C
Here are two examples that demonstrate the usage of the sizeof()
operator:
Example 1: Finding the size of a datatype
#include <stdio.h>
int main() {
int size = sizeof(int);
printf("The size of int is: %d bytes\n", size);
return 0;
}
Output:
The size of int is: 4 bytes
Explanation:
In above example, we use the sizeof()
operator to find the size of the int
datatype. The sizeof(int)
returns the size of an integer in bytes, which is typically 4 bytes on most modern systems.
Example 2: Determining the size of an array
#include <stdio.h>
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
printf("The size of the array is: %d\n", size);
return 0;
}
Output:
The size of the array is: 5
Explanation:
In above example, we have an array arr
containing 5 elements. To determine the size of the array, we divide the total size of the array (sizeof(arr)
) by the size of a single element (sizeof(arr[0])
). This gives us the number of elements in the array, which is then printed as the output.
Rules to be followed when using sizeof() operator in C
- Operand type: The operand of the
sizeof()
operator can be a datatype, variable, or expression. It should be enclosed in parentheses. For example:sizeof(int)
,sizeof(variable)
, orsizeof(expression)
. - Parentheses: Always use parentheses around the operand of
sizeof()
. This ensures that the operand is correctly interpreted as a single entity. For example:sizeof(int)
, notsizeof int
. - Compile-time evaluation: The
sizeof()
operator is evaluated at compile-time, not runtime. It determines the size of the operand based on the datatype. Therefore, it cannot be used to determine the size of dynamically allocated memory or runtime variables. - Arrays: When used with an array, the
sizeof()
operator returns the size of the entire array in bytes, not the number of elements. To get the number of elements in an array, divide the total size of the array by the size of a single element. For example:sizeof(array) / sizeof(array[0])
. - Pointers: When used with a pointer, the
sizeof()
operator returns the size of the pointer itself, not the size of the object it points to. To get the size of the object, dereference the pointer and then usesizeof()
. For example:sizeof(*pointer)
. - Structures and Unions: The
sizeof()
operator provides the size of a structure or union, including padding. It calculates the total size by summing the sizes of all members, including any padding inserted by the compiler for alignment. - Compiler-specific behavior: The size calculated by
sizeof()
may vary depending on the compiler, platform, and compiler flags used. Therefore, it’s important to be aware of any compiler-specific behavior that might affect the size calculation.