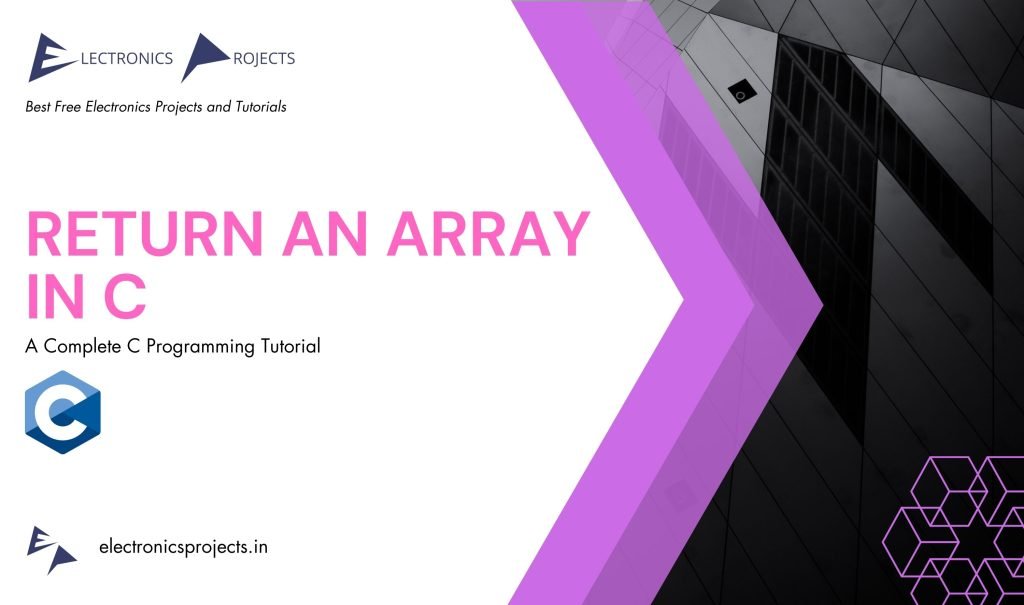
What is returning an Array in C?
Returning an array in C involves creating a function that returns an array as its result. This can be useful when you need to pass back multiple values from a function or manipulate an array inside a function and return the modified array.
Methods to return an Array in C:
1. Returning a pointer to the array
In C, arrays decay into pointers when passed to functions. So, you can return a pointer to an array from a function. However, you need to be cautious when using this method, as the memory for the array must be dynamically allocated or declared as static to ensure its availability outside the function.
#include <stdio.h>
#include <stdlib.h>
int* createArray(int size) {
int* arr = malloc(size * sizeof(int));
for (int i = 0; i < size; i++) {
arr[i] = i + 1;
}
return arr;
}
int main() {
int* array = createArray(5);
for (int i = 0; i < 5; i++) {
printf("%d ", array[i]);
}
free(array); // Don't forget to free the dynamically allocated memory
return 0;
}
Output:
1 2 3 4 5
2. Using a struct to encapsulate the array
Another approach is to define a struct that contains the array as a member and return an instance of that struct from the function. This allows you to return the entire array as part of a larger data structure.
#include <stdio.h>
typedef struct {
int array[5];
} ArrayContainer;
ArrayContainer createArray() {
ArrayContainer container;
for (int i = 0; i < 5; i++) {
container.array[i] = i + 1;
}
return container;
}
int main() {
ArrayContainer container = createArray();
for (int i = 0; i < 5; i++) {
printf("%d ", container.array[i]);
}
return 0;
}
Output:
1 2 3 4 5
3. Using a global array
Instead of returning an array from a function, you can declare the array as a global variable and manipulate it inside the function. This allows other parts of the program to access the modified array.
#include <stdio.h>
int arr[5];
void modifyArray() {
for (int i = 0; i < 5; i++) {
arr[i] = i + 1;
}
}
int main() {
modifyArray();
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Output:
1 2 3 4 5
4. Passing the Array as a function argument
Instead of returning the array from a function, you can pass it as an argument and modify it directly inside the function.
#include <stdio.h>
void modifyArray(int* arr, int size) {
for (int i = 0; i < size; i++) {
arr[i] = i + 1;
}
}
int main() {
int array[5];
modifyArray(array, 5);
for (int i = 0; i < 5; i++) {
printf("%d ", array[i]);
}
return 0;
}
Output:
1 2 3 4 5
5. Using a dynamic data structure
Instead of returning a raw array, you can utilize dynamic data structures like linked lists or dynamic arrays (e.g., ArrayList). You can create the array-like structure dynamically, populate it with elements, and return a pointer to the structure.
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int* array;
int size;
} DynamicArray;
DynamicArray* createDynamicArray(int size) {
DynamicArray* dynamicArray = malloc(sizeof(DynamicArray));
dynamicArray->array = malloc(size * sizeof(int));
dynamicArray->size = size;
for (int i = 0; i < size; i++) {
dynamicArray->array[i] = i + 1;
}
return dynamicArray;
}
void destroyDynamicArray(DynamicArray* dynamicArray) {
free(dynamicArray->array);
free(dynamicArray);
}
int main() {
DynamicArray* dynamicArray = createDynamicArray(5);
for (int i = 0; i < dynamicArray->size; i++) {
printf("%d ", dynamicArray->array[i]);
}
destroyDynamicArray(dynamicArray);
return 0;
}
Output:
1 2 3 4 5
Rules to follow when returning an array in C:
- Avoid returning a pointer to a local array: If you declare an array inside a function and attempt to return a pointer to that array, it will lead to undefined behavior. Local variables have automatic storage duration, meaning their memory is deallocated once the function exits. Therefore, returning a pointer to a local array can result in accessing invalid memory.
- Allocate memory dynamically or use static arrays: To safely return an array, ensure that the array memory is allocated dynamically using functions like
malloc()
orcalloc()
. Alternatively, you can use static arrays declared outside any function, which have static storage duration and retain their memory throughout the program’s execution. - Document memory ownership and deallocation responsibility: Clearly document who is responsible for deallocating the returned array. If the memory is dynamically allocated, it is typically the caller’s responsibility to free the memory using
free()
once it is no longer needed. Make sure to communicate this in the function’s documentation or comments. - Specify array size or provide a termination condition: If the size of the returned array is fixed, communicate it to the caller either through function documentation or by returning the size along with the array. If the array size is variable or unknown, consider using a termination condition to indicate the end of the array (e.g., using a sentinel value or a separate length parameter).
- Handle potential allocation failures: When dynamically allocating memory for the array, check if the allocation was successful. If the allocation fails (returns NULL), handle the error gracefully, either by terminating the program or by returning an error code or NULL pointer to indicate the failure.
- Avoid returning a dangling pointer: Ensure that the returned array pointer remains valid even after the function exits. Avoid returning pointers to local variables or memory that has been deallocated. If the array is declared inside the function, consider using one of the alternative methods mentioned earlier, such as passing the array as an argument or using a global array.