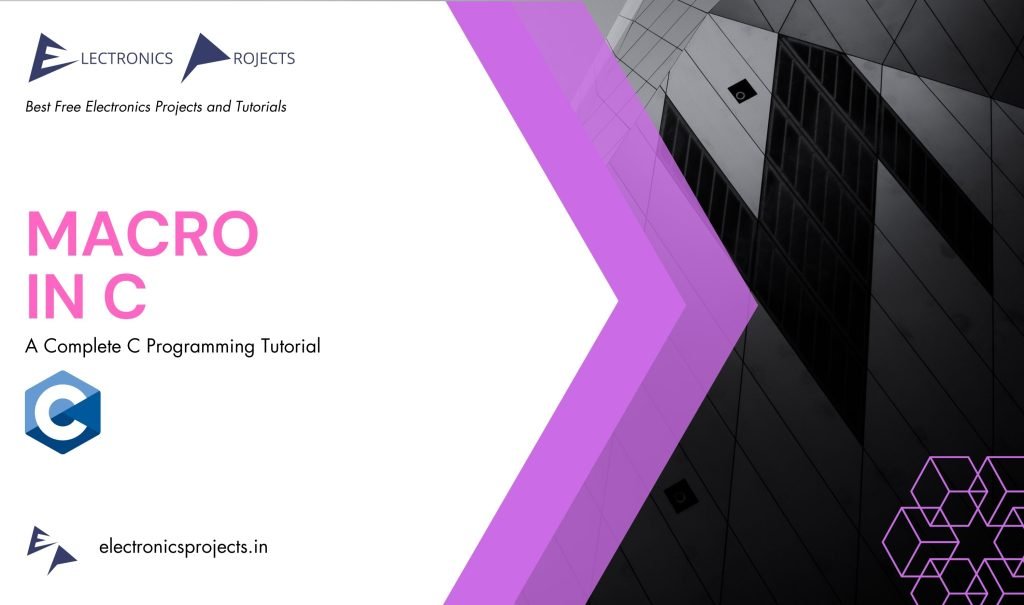
What is Macro in C ?
In C, a macro is a preprocessor directive that allows you to define a symbolic name or identifier for a specific sequence of code or values. Macros are used for code generation and code replacement before the actual compilation process begins. They are processed by the C preprocessor (a part of the compiler) before the source code is compiled into machine code. Macros are often used to make code more readable, maintainable, and to avoid repetitive tasks.
Syntax for defining macro in C:
#define MACRO_NAME replacement_value
Where:
MACRO_NAME
is the symbolic name you want to define for the replacement.replacement_value
is the code or value you want to associate with the macro.
Examples of Macro in C
Example 1:
#include <stdio.h>
#define SQUARE(x) (x * x)
int main() {
int num = 5;
int result = SQUARE(num);
printf("The square of %d is %d\n", num, result);
return 0;
}
Output:
The square of 5 is 25
Explanation:
In above example, we define a macro SQUARE(x)
that squares the input x
. When we call SQUARE(num)
with num
being 5, it gets replaced with (5 * 5)
by the preprocessor, resulting in result
being assigned the value 25.
Example 2:
#include <stdio.h>
#define MAX(a, b) ((a > b) ? a : b)
int main() {
int num1 = 10, num2 = 7;
int max = MAX(num1, num2);
printf("The maximum between %d and %d is %d\n", num1, num2, max);
return 0;
}
Output:
The maximum between 10 and 7 is 10
Explanation:
In above example, we define a macro MAX(a, b)
that returns the maximum of two values a
and b
. When we call MAX(num1, num2)
with num1
being 10 and num2
being 7, it gets replaced with ((10 > 7) ? 10 : 7)
by the preprocessor, resulting in max
being assigned the value 10.
Rules to be followed when using Macro in C
- Define Macros UPPERCASE: Conventionally, macros are defined in uppercase to distinguish them from regular C code.
- Parentheses: Ensure that you use parentheses appropriately within macros to avoid unexpected operator precedence issues. This is especially important when macros involve mathematical operations.
- Avoid Side Effects: Be cautious when using macros that have side effects, such as modifying variables or performing I/O operations, as they can lead to unexpected behavior.
- Use Macros for Constants: Macros are often used for defining constants like
#define PI 3.14159265
to improve code readability. - Keep Macros Simple: Keep macros simple and easy to understand. Complex macros can make code difficult to read and debug.
- Document Macros: Include comments that explain the purpose and usage of macros to make your code more maintainable.
- Avoid Recursion: Avoid defining macros in terms of themselves (recursive macros) as it can lead to infinite expansion during preprocessing.
- Use Macros Judiciously: While macros can be powerful, they should be used judiciously. In some cases, using inline functions or const variables may be a better choice for code clarity and type safety.