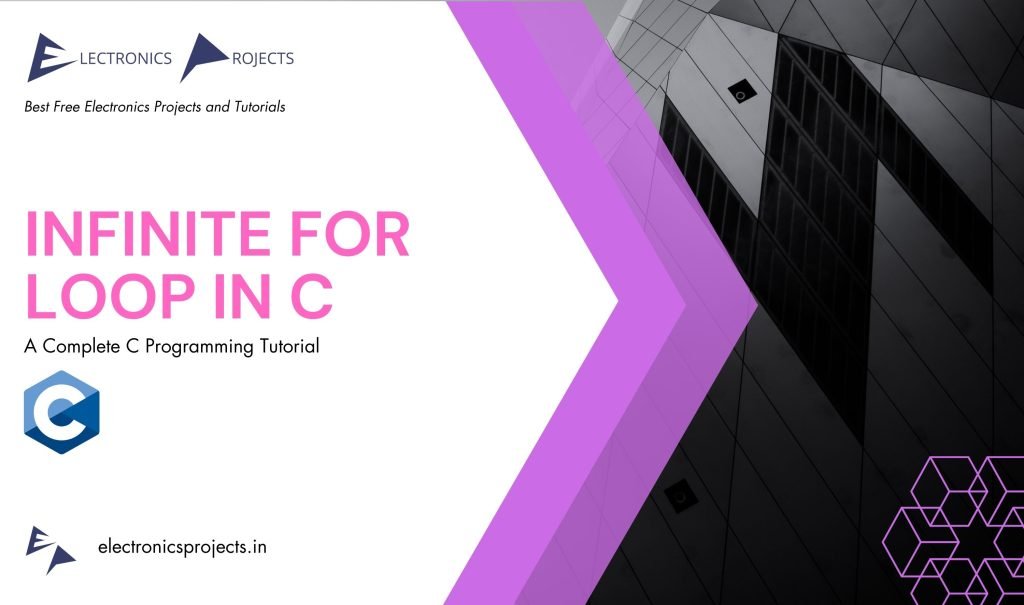
What is Infinite for loop in C?
An infinite for loop in C is a loop that runs indefinitely, without any condition to terminate the loop. It means that the loop continues to execute its body repeatedly as long as the program is running.
There are a few reasons why an infinite for loop is used in C. For instance, it can be used when the program needs to perform a task repeatedly, and the number of iterations is not known in advance. It can also be used to implement a real-time system, such as a program that needs to monitor input continuously.
Syntax:
for (initialization; ; increment/decrement) {
// Code to be executed repeatedly
}
Examples of Infinite for loop:
Example 1:
#include <stdio.h>
int main() {
for (;;) {
printf("This is an infinite loop\n");
}
return 0;
}
Output:
This is an infinite loop
This is an infinite loop
This is an infinite loop
...
Explanation:
In above example, the for loop runs continuously without any termination condition. Therefore, the statement inside the loop (printf()
) will be executed repeatedly, resulting in an infinite loop that prints “This is an infinite loop” repeatedly.
Example 2:
#include <stdio.h>
int main() {
int i = 0;
for (; i < 5;) {
printf("This is also an infinite loop\n");
}
return 0;
}
Output:
This is also an infinite loop
This is also an infinite loop
This is also an infinite loop
...
Explanation:
In above example, the loop condition is omitted from the for loop declaration, and the loop variable (i
) is incremented inside the loop statement. However, the value of i
is never changed, so the loop runs infinitely, resulting in an infinite loop that prints “This is also an infinite loop” repeatedly.
Example 3:
#include <stdio.h>
int main() {
int i = 0;
for (;;) {
if (i < 5) {
printf("%d ", i);
i++;
}
}
return 0;
}
Output:
0 1 2 3 4 0 1 2 3 4 0 1 2 3 4 ...
Explanation:
In above example, the loop runs infinitely since the loop condition is omitted from the for loop declaration. Inside the loop, an if statement is used to print the value of the loop variable (i
) if it is less than 5. The value of i
is then incremented. Since the loop condition is never met, the loop will continue to print the values of i
in a cyclic manner (0 to 4).
Example 4:
#include <stdio.h>
int main() {
int i = 0;
for (;;) {
i++;
printf("%d\n", i);
}
return 0;
}
Output:
1
2
3
...
Explanation:
In above example, the loop runs indefinitely, and the program keeps incrementing the value of i and printing it to the console.
Rules to follow while using Infinite for loop:
- Make sure to have a way to break out of the loop (e.g. using a break statement).
- Use an infinite loop only when necessary and ensure that the code inside the loop does not cause any unwanted side effects.
- Avoid using an infinite loop for time-consuming operations.
- Ensure that the loop variable is updated correctly to prevent an infinite loop.