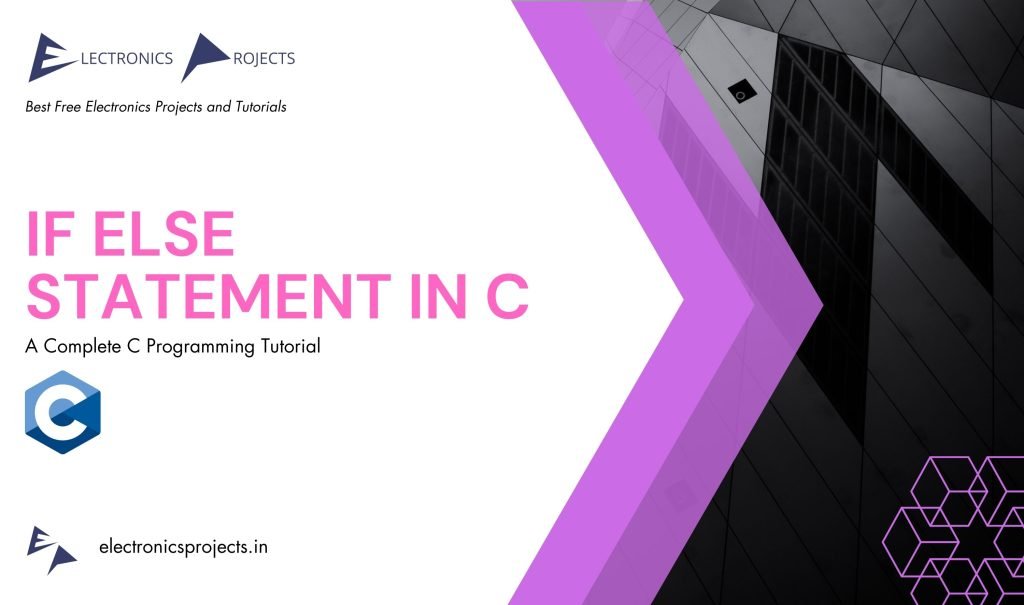
What is If Else Statement in C?
In C programming language, the If statement is used for conditional execution of code. It evaluates the expression inside the parentheses, and if the expression is true, it executes the code inside the block of curly braces that follows.
1. If Statement in C:
Syntax:
if (expression)
{
// code to execute if the expression is true
}
Example:
#include <stdio.h>
int main() {
int num = 10;
if (num > 0) {
printf("The number is positive.\n");
}
return 0;
}
Output:
The number is positive.
Explanation:
In above example, we have defined a variable “num” with the value of 10. We have then used an If statement to check whether the value of “num” is greater than 0 or not. Since 10 is indeed greater than 0, the condition is true and the statement inside the If block is executed, which in this case is to print “The number is positive.” to the console. The program then terminates with a return value of 0. If the value of “num” was negative, then the condition would have been false and the statement inside the If block would not have been executed.
2. If-Else Statement in C:
In the If-Else statement, the program executes one block of code if the expression is true, and the other block of code if the expression is false.
Syntax:
if (expression)
{
// code to execute if the expression is true
}
Example:
#include <stdio.h>
int main() {
int num = 10;
if (num > 0) {
printf("The number is positive.\n");
}
else {
printf("The number is non-positive.\n");
}
return 0;
}
Output:
The number is positive.
Explanation:
In the above example, we are using the If-Else statement to check if the variable “num” is greater than zero or not. If the variable “num” is greater than zero, then the message “The number is positive.” is printed on the screen. Otherwise, the message “The number is non-positive.” is printed on the screen.
3. Nested If-Else Statement in C:
In the Nested If-Else statement, we use one If-Else statement inside another If-Else statement.
Syntax:
if (expression1)
{
// code to execute if expression1 is true
if (expression2)
{
// code to execute if both expression1 and expression2 are true
}
else
{
// code to execute if expression1 is true and expression2 is false
}
}
else
{
// code to execute if expression1 is false
}
Example:
#include <stdio.h>
int main() {
int num = 10;
if (num > 0) {
if (num < 100) {
printf("The number is between 0 and 100.\n");
}
else {
printf("The number is greater than or equal to 100.\n");
}
}
else {
printf("The number is non-positive.\n");
}
return 0;
}
Output:
The number is between 0 and 100.
Explanation:
In the above example, we are using a Nested If-Else statement to check if the variable “num” is greater than zero or not. If the variable “num” is greater than zero, then the second If statement checks if the variable “num” is less than 100 or not. If the variable “num” is less than 100, then the message “The number is between 0 and 100.” is printed on the screen. Otherwise, the message “The number is greater than or equal to 100.” is printed on the screen. If the variable “num” is not greater than zero, then the message “The number is non-positive.” is printed on the screen.
4. If-Else If Ladder Statement in C:
In the If-Else If ladder statement, we use multiple If-Else statements to check multiple conditions one by one.
Syntax:
if (expression1)
{
// code to execute if expression1 is true
}
else if (expression2)
{
// code to execute if expression1 is false and expression2 is true
}
else if (expression3)
{
// code to execute if expression1 and expression2 are false and expression3 is true
}
.
.
.
else
{
// code to execute if all the expressions are false
}
Example:
#include <stdio.h>
int main() {
int num = 20;
if (num < 0) {
printf("The number is negative.\n");
}
else if (num == 0) {
printf("The number is zero.\n");
}
else if (num > 0 && num < 10) {
printf("The number is between 0 and 10.\n");
}
else if (num >= 10 && num < 20) {
printf("The number is between 10 and 20.\n");
}
else {
printf("The number is greater than or equal to 20.\n");
}
return 0;
}
Output:
The number is between 10 and 20.
Explanation:
In the above example, we are using an If-Else If ladder statement to check the value of the variable “num” and print a message accordingly. The first If statement checks if the variable “num” is negative or not. If it is negative, then the message “The number is negative.” is printed. If it is not negative, then the second If statement checks if the variable “num” is equal to zero or not. If it is equal to zero, then the message “The number is zero.” is printed. If it is not equal to zero, then the third If statement checks if the variable “num” is between 0 and 10 or not. If it is between 0 and 10, then the message “The number is between 0 and 10.” is printed. If it is not between 0 and 10, then the fourth If statement checks if the variable “num” is between 10 and 20 or not. If it is between 10 and 20, then the message “The number is between 10 and 20.” is printed. If it is not between 10 and 20, then the Else block is executed and the message “The number is greater than or equal to 20.” is printed.
Rules while using If Else Statements in C:
- The expression inside the parentheses of the If statement must be a Boolean expression (either true or false).
- The code inside the block of curly braces following the If or Else keyword should be indented for readability.
- The code inside the block of curly braces following the If or Else keyword should be enclosed in curly braces, even if there is only one line of code, to avoid errors caused by ambiguity.
- The If statement can be used with or without an Else statement.
- The Else statement must be immediately preceded by an If statement.
- The If-Else statements can be nested.
- The order of the conditions in the If-Else If ladder statements is important. The first condition that evaluates to true will cause the corresponding block of code to execute. Therefore, it is important to order the conditions appropriately.
- The If-Else statements can be used with other control flow statements, such as loops.
Modifications that can be made while using If Else statement in C:
1. Using the Comma Operator:
The comma operator allows the execution of multiple expressions in a single statement. In an If-Else statement, the comma operator can be used to execute multiple statements within the If or Else block.
Example:
if (num >= 0)
printf("The number is positive."), printf("This message is also printed.\n");
else
printf("The number is negative.\n");
Output:
The number is positive.
This message is also printed.
2. Using the Conditional Operator:
The conditional operator (?:) is a shorthand way of writing an If-Else statement with a single condition. It is particularly useful when a simple assignment needs to be made based on a condition.
Example:
int num = -10;
int abs_num = (num >= 0) ? num : -num;
printf("The absolute value of %d is %d.\n", num, abs_num);
Output:
The absolute value of -10 is 10.
3. Using multiple If-Else statements instead of nested If-Else statements:
Instead of using nested If-Else statements, multiple If-Else statements can be used to check for multiple conditions. This can make the code easier to read and understand.
Example:
if (num < 0)
printf("The number is negative.\n");
if (num == 0)
printf("The number is zero.\n");
if (num > 0 && num < 10)
printf("The number is between 0 and 10.\n");
if (num >= 10 && num < 20)
printf("The number is between 10 and 20.\n");
if (num >= 20)
printf("The number is greater than or equal to 20.\n");
Output:
The number is between 10 and 20.
4. Using the Logical Operators:
Logical operators (&&, ||, !) can be used to combine multiple conditions into a single If or Else statement. This can make the code more concise and easier to read.
Example:
if (num >= 0 && num <= 100)
printf("The number is between 0 and 100.\n");
else if (num > 100 && num <= 1000)
printf("The number is between 100 and 1000.\n");
else if (num > 1000)
printf("The number is greater than 1000.\n");
else
printf("The number is negative.\n");
Output:
The number is between 0 and 100.