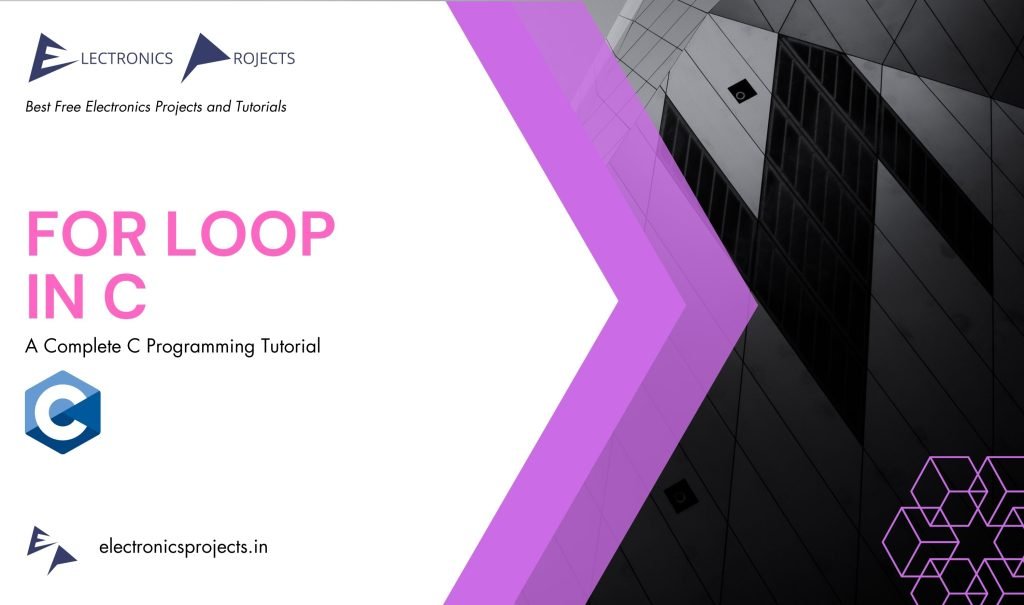
What is for loop in C?
A for loop is a control flow statement that allows you to execute a block of code repeatedly for a fixed number of times. The for loop provides a convenient way to iterate over a range of values, with a built-in loop counter that can be used to control the flow of execution.
for (initialization; condition; increment/decrement) {
// code to be executed repeatedly
}
Here’s what each part of the for loop means:
- initialization initializes the loop counter variable before the loop starts.
- condition is a Boolean expression that determines whether the loop continues to run or not.
- increment/decrement changes the value of the loop counter variable after each iteration.
During each iteration of the loop, the loop variable is tested against the loop condition. If the condition is true, the statements in the body of the loop are executed. After the statements in the body of the loop are executed, the loop variable is incremented by the increment statement. This process continues until the loop condition is false.
The for loop is commonly used in C programs to perform repetitive tasks, such as iterating over arrays or lists, generating sequences of numbers, and processing data streams. With the for loop, you can write concise and efficient code that automates common programming tasks and makes your programs more powerful and flexible.
Types of for loops in C:
There are three types of for loops in C:
- for loop with initialization, condition and increment/decrement statement.
- for loop with only condition statement.
- for loop with multiple initialization and increment/decrement statements.
1. for loop with initialization, condition and increment/decrement statement:
This type of for loop is the most commonly used type. It is used when the loop counter needs to be initialized, a condition needs to be checked before each iteration, and the counter needs to be incremented or decremented after each iteration.
Syntax:
for (initialization; condition; increment/decrement) {
// code to be executed repeatedly
}
Example:
#include <stdio.h>
int main() {
int i;
for (i = 1; i <= 5; i++) {
printf("%d\n", i);
}
return 0;
}
Output:
1
2
3
4
5
Explanation:
- The loop counter
i
is initialized to1
. - The condition
i <= 5
is checked before each iteration. As long as the condition is true, the loop will continue to run. - Inside the loop, the value of
i
is printed usingprintf()
function. - After each iteration, the value of
i
is incremented by1
.
2. for loop with only condition statement:
This type of for loop is used when the loop counter has already been initialized and incremented or decremented outside the loop, and only a condition needs to be checked before each iteration.
Syntax:
for (; condition;) {
// code to be executed repeatedly
}
Example:
#include <stdio.h>
int main() {
int i = 1;
for (; i <= 5;) {
printf("%d\n", i);
i++;
}
return 0;
}
Output:
1
2
3
4
5
Explanation:
- The loop counter
i
is initialized to1
outside the loop. - Inside the loop, the value of
i
is printed usingprintf()
function. - After each iteration, the value of
i
is incremented by1
.
3. for loop with multiple initialization and increment/decrement statements:
This type of for loop is used when there are multiple loop counters that need to be initialized, and multiple increment/decrement statements that need to be executed after each iteration.
Syntax:
for (initialization; condition; increment/decrement, increment/decrement, ...) {
// code to be executed repeatedly
}
Example:
#include <stdio.h>
int main() {
int i, j;
for (i = 1, j = 5; i <= 5 && j >=1; i++, j--) {
printf("%d %d\n", i, j);
}
return 0;
}
Output:
1 5
2 4
3 3
4 2
5 1
Explanation:
- Two loop counters
i
andj
are initialized to1
and5
, respectively. - The condition
i <= 5 && j >= 1
is checked before each iteration. As long as the condition is true, the loop will continue to run. - Inside the loop, the values of
i
andj
are printed usingprintf()
function. - After each iteration, the value of
i
is incremented by1
and the value ofj
is decremented by1
.
Examples of for loop:
1. Printing Even Numbers
This for loop prints the first 10 even numbers starting from 0.
#include <stdio.h>
int main() {
int i;
for (i = 0; i <= 18; i += 2) {
printf("%d ", i);
}
return 0;
}
Output:
0 2 4 6 8 10 12 14 16 18
Explanation:
- The loop counter variable
i
is initialized to0
. - The condition
i <= 18
is checked before each iteration. As long as the condition is true, the loop will continue to run. - Inside the loop, the value of
i
is printed usingprintf()
function. - After each iteration, the value of
i
is incremented by2
, so that only even numbers are printed.
2. Calculating Factorial
This for loop calculates the factorial of a given number n
.
#include <stdio.h>
int main() {
int n, i, fact = 1;
printf("Enter a positive integer: ");
scanf("%d", &n);
for (i = 1; i <= n; i++) {
fact *= i;
}
printf("Factorial of %d is %d", n, fact);
return 0;
}
Output:
Enter a positive integer: 5
Factorial of 5 is 120
Explanation:
- The loop counter variable
i
is initialized to1
. - The condition
i <= n
is checked before each iteration. As long as the condition is true, the loop will continue to run. - Inside the loop, the value of
fact
is multiplied byi
. - After each iteration, the value of
i
is incremented by1
, so that the loop multipliesfact
by every integer from1
ton
.
3. Nested Loops for Multiplication Table
This for loop uses nested loops to print the multiplication table for numbers from 1 to 5.
#include <stdio.h>
int main() {
int i, j;
for (i = 1; i <= 5; i++) {
for (j = 1; j <= 10; j++) {
printf("%d x %d = %d\n", i, j, i*j);
}
printf("\n");
}
return 0;
}
Output:
1 x 1 = 1
1 x 2 = 2
1 x 3 = 3
1 x 4 = 4
1 x 5 = 5
1 x 6 = 6
1 x 7 = 7
1 x 8 = 8
1 x 9 = 9
1 x 10 = 10
2 x 1 = 2
2 x 2 = 4
2 x 3 = 6
2 x 4 = 8
2 x 5 = 10
2 x 6 = 12
2 x 7 = 14
2 x 8 = 16
2 x 9 = 18
2 x 10 = 20
3 x 1 = 3
3 x 2 = 6
3 x 3 = 9
3 x 4 = 12
3 x 5 = 15
3 x 6 = 18
3 x 7 = 21
3 x 8 = 24
3 x 9 = 27
3 x 10 = 30
4 x 1 = 4
4 x 2 = 8
4 x 3 = 12
4 x 4 = 16
4 x 5 = 20
4 x 6 = 24
4 x 7 = 28
4 x 8 = 32
4 x 9 = 36
4 x 10 = 40
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
Explanation:
- The outer loop counter variable
i
is initialized to1
and iterates over the numbers from1
to5
. - The inner loop counter variable
j
is initialized to1
and iterates over the numbers from1
to10
. - Inside the inner loop, the product of
i
andj
is printed usingprintf()
function. - After each iteration of the inner loop, a newline character is printed using
printf()
function. - After each iteration of the outer loop, an additional newline character is printed to separate the multiplication tables for each number.
4. Reversing an Array
This example shows how to use a for loop to reverse an array of integers.
#include <stdio.h>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
int i, j, temp;
printf("Original array: ");
for(i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
for(i = 0, j = 4; i < j; i++, j--) {
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
printf("\nReversed array: ");
for(i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Output:
Original array: 1 2 3 4 5
Reversed array: 5 4 3 2 1
Explanation:
- The array
arr
is initialized with 5 integers. - The original array is printed using a for loop.
- A second for loop is used to swap the elements of the array. The loop starts from the first element and the last element and moves towards the middle of the array, swapping the elements at each step.
- The reversed array is printed using another for loop.
5. Prime Numbers
This example shows how to use a for loop to print all prime numbers between 1 and 100.
#include <stdio.h>
int main() {
int i, j, isPrime;
printf("Prime numbers between 1 and 100:\n");
for(i = 2; i <= 100; i++) {
isPrime = 1;
for(j = 2; j <= i/2; j++) {
if(i % j == 0) {
isPrime = 0;
break;
}
}
if(isPrime == 1) {
printf("%d ", i);
}
}
return 0;
}
Output:
Prime numbers between 1 and 100:
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
Explanation:
- The outer for loop iterates over the numbers from 2 to 100.
- For each number, an inner for loop checks if it is a prime number by dividing it by all numbers from 2 to half of the number. If the number is divisible by any of these numbers, it is not a prime number.
- If the number is prime, it is printed using printf() function.
6. Fibonacci Sequence
This example shows how to use a for loop to generate the first 20 numbers in the Fibonacci sequence.
#include <stdio.h>
int main() {
int i, num1 = 0, num2 = 1, fib;
printf("First 20 numbers in Fibonacci sequence:\n");
for(i = 1; i <= 20; i++) {
printf("%d ", num1);
fib = num1 + num2;
num1 = num2;
num2 = fib;
}
return 0;
}
Output:
First 20 numbers in Fibonacci sequence:
0 1 1 2 3 5
Explanation:
- The first two numbers in the Fibonacci sequence are 0 and 1. These are stored in the variables
num1
andnum2
. - The for loop runs 20 times to generate the first 20 numbers in the sequence.
- Inside the loop, the current number in the sequence is printed using printf().
- The next number in the sequence is calculated by adding
num1
andnum2
, and stored in the variablefib
. - The values of
num1
andnum2
are updated to generate the next number in the sequence.
Rules to follow while using Loops in C:
- The loop variable must be initialized before the loop starts.
- The loop condition must be a boolean expression that evaluates to true or false.
- The loop variable must be incremented or decremented in each iteration of the loop.
- The body of the loop must be enclosed in braces {}.
- The loop variable should not be modified inside the body of the loop, unless you know what you’re doing.
- The loop should not run forever, unless you have a good reason for doing so.
Modifications that can be made when using for loop:
- You can use different loop variables, with different data types and starting/ending values.
- You can use different loop conditions, including boolean expressions that involve multiple variables or functions.
- You can modify the loop variable by incrementing or decrementing it by a value other than 1.
- You can use multiple statements in the body of the loop, including if statements, switch statements, and function calls.
- You can use the break statement to exit the loop prematurely if a certain condition is met.
- You can use the continue statement to skip the rest of the current iteration and move on to the next one.