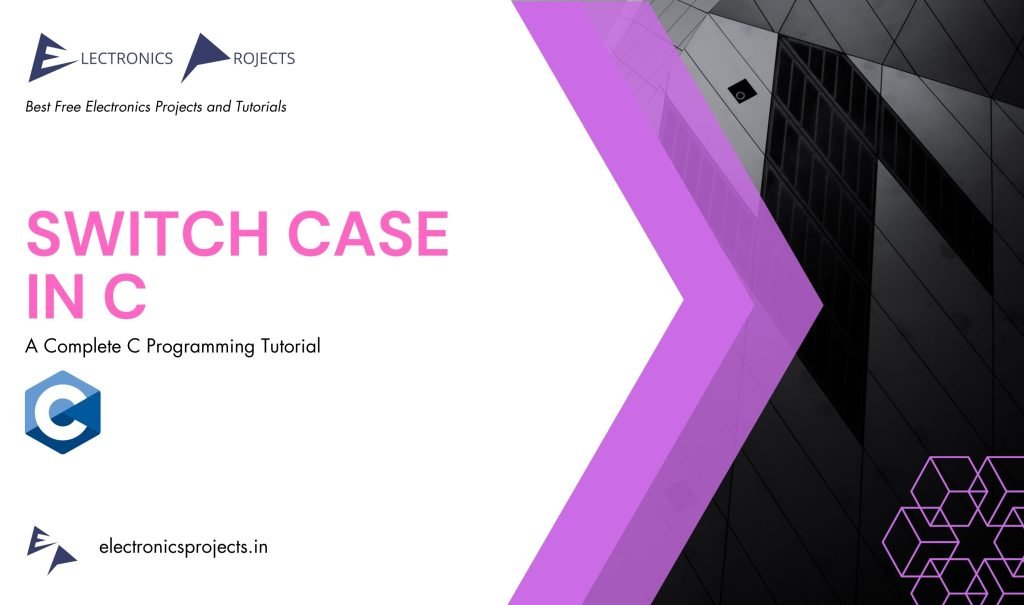
What is Switch Case in C?
Switch case is a control flow statement used in C programming language to execute different actions depending on the value of an expression. The switch statement evaluates the value of a single expression and then compares it to a set of cases to determine which action to perform.
Switch case is typically used as an alternative to a long chain of if-else statements. It provides a more concise and readable way to handle multiple cases.
Syntax:
switch (expression) {
case constant1:
// code to execute if expression is equal to constant1
break;
case constant2:
// code to execute if expression is equal to constant2
break;
// more cases can be added here
default:
// code to execute if expression doesn't match any of the cases
break;
}
Example:
#include <stdio.h>
int main() {
int num;
printf("Enter a number between 1 and 3: ");
scanf("%d", &num);
switch (num) {
case 1:
printf("You entered one.\n");
break;
case 2:
printf("You entered two.\n");
break;
case 3:
printf("You entered three.\n");
break;
default:
printf("Invalid input.\n");
break;
}
return 0;
}
Output:
Enter a number between 1 and 3: 2
You entered two.
Explanation:
In this example, the user is prompted to enter a number between 1 and 3. The scanf
function is used to read in the user’s input and store it in the variable num
. The switch case statement is then used to check the value of num
against the possible cases of 1, 2, and 3. If num
matches one of these cases, the corresponding message is printed to the screen. If num
doesn’t match any of the cases, the default message is printed.
Examples of Switch Case in C:
1. Calculator using Switch Case in C:
#include <stdio.h>
int main()
{
char operator;
double num1, num2, result;
printf("Enter an operator (+, -, *, /): ");
scanf("%c", &operator);
printf("Enter two numbers: ");
scanf("%lf %lf", &num1, &num2);
switch(operator)
{
case '+':
result = num1 + num2;
printf("%.2lf + %.2lf = %.2lf", num1, num2, result);
break;
case '-':
result = num1 - num2;
printf("%.2lf - %.2lf = %.2lf", num1, num2, result);
break;
case '*':
result = num1 * num2;
printf("%.2lf * %.2lf = %.2lf", num1, num2, result);
break;
case '/':
result = num1 / num2;
printf("%.2lf / %.2lf = %.2lf", num1, num2, result);
break;
default:
printf("Invalid operator");
break;
}
return 0;
}
Output:
Enter an operator (+, -, *, /): *
Enter two numbers: 4.2 2.3
4.20 * 2.30 = 9.66
Explanation:
This program takes an operator (+, -, *, /) and two numbers as input from the user. It performs the corresponding arithmetic operation on the numbers based on the operator entered using switch case. The result is then printed to the console. If an invalid operator is entered, the program prints “Invalid operator” to the console.
2. Grade Calculator using Switch Case in C:
#include <stdio.h>
int main()
{
int marks;
printf("Enter your marks (out of 100): ");
scanf("%d", &marks);
switch(marks / 10)
{
case 10:
case 9:
printf("Your grade is A");
break;
case 8:
printf("Your grade is B");
break;
case 7:
printf("Your grade is C");
break;
case 6:
printf("Your grade is D");
break;
case 5:
printf("Your grade is E");
break;
default:
printf("Your grade is F");
break;
}
return 0;
}
Output:
Enter your marks (out of 100): 76
Your grade is C
Explanation:
This program takes the marks obtained by a student (out of 100) as input and uses switch case to determine the corresponding grade. The switch case is based on the marks obtained, divided by 10. If the marks are between 90 and 100, the grade is A, if the marks are between 80 and 89, the grade is B, and so on. If the marks are below 50, the grade is F.
3. Days in a Month:
#include <stdio.h>
int main()
{
int month, year, days;
printf("Enter month (1-12): ");
scanf("%d", &month);
printf("Enter year: ");
scanf("%d", &year);
switch(month)
{
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
case 2:
days = (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) ? 29 : 28;
break;
default:
days = 31;
break;
}
printf("Number of days in the month is: %d", days);
return 0;
}
Output:
Enter month (1-12): 2
Enter year: 2024
Number of days in the month is: 29
This updated program takes a month and year as input from the user and uses switch case to determine the number of days in the month. For the months of April, June, September, and November, the program sets the number of days to 30, while for February, the program checks if the year is a leap year or not using a ternary operator. If the year is a leap year, the number of days is set to 29, otherwise it is set to 28. For all other months, the number of days is set to 31. The program then prints the number of days to the console.