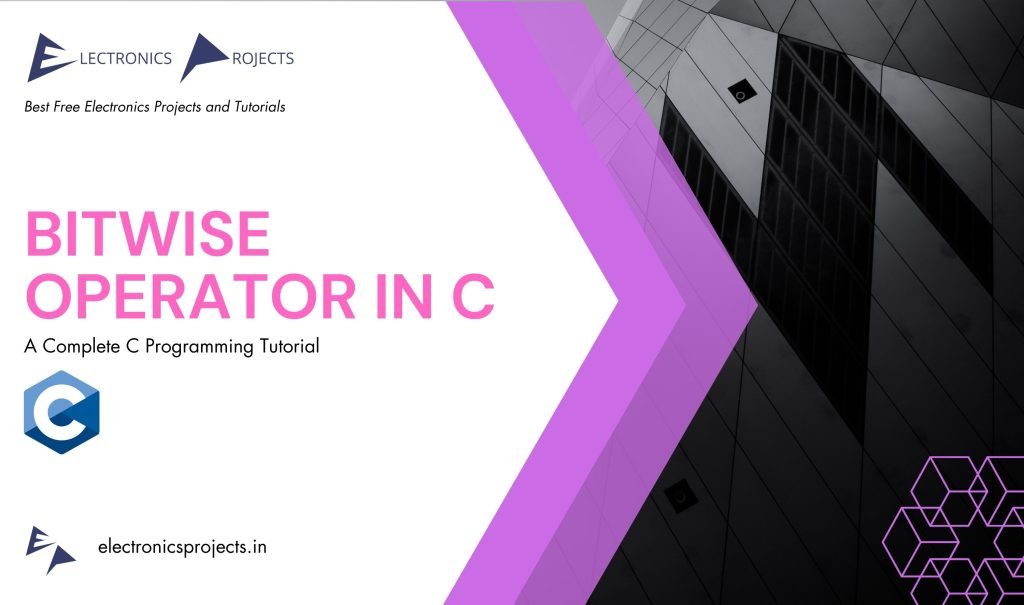
What is Bitwise Operator in C?
Bitwise operators in C are used to manipulate individual bits of data. They perform operations on the binary representations of integers. Bitwise operators can be used to set, clear or toggle bits within an integer, and to shift bits left or right.
Types of Bitwise Operator in C:
There are six bitwise operators in C:
Sr. No. | Operator | Symbol | Information |
---|---|---|---|
1 | Bitwise AND | & | Sets each bit to 1 if both bits are 1. |
2 | Bitwise OR | | | Sets each bit to 1 if one of the two bits is 1. |
3 | Bitwise XOR | ^ | Sets each bit to 1 if only one of the two bits is 1. |
4 | Bitwise NOT | ~ | Inverts all the bits. |
5 | Left Shift | << | Shifts the bits of the first operand to the left. |
6 | Right Shift | >> | Shifts the bits of the first operand to the right. |
Truth Table:
A | B | A&B | A|B | A^B |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
A | -A |
---|---|
0 | 1 |
1 | 0 |
1. Bitwise AND:
In C, the bitwise AND operator is represented by the “&” symbol. It is a binary operator that takes two integer operands and performs a bitwise AND operation on them.
The bitwise AND operation compares each bit of the two operands and produces a new integer where each bit in the result is set to 1 only if the corresponding bits of both operands are also 1. Otherwise, the result bit is set to 0.
Example:
#include <stdio.h>
int main() {
int num1 = 35; // Binary: 00100011
int num2 = 45; // Binary: 00101101
int result = num1 & num2;
printf("num1: %d\n", num1);
printf("num2: %d\n", num2);
printf("result: %d\n", result);
return 0;
}
Output:
num1: 35
num2: 45
result: 33
Explanation:
In above example, we declare two integer variables num1
and num2
with the values 35 and 45 respectively. These values are represented in binary to make it easier to see the individual bits.
We then declare a third integer variable result
and set its value to the result of performing a bitwise AND operation on num1
and num2
.
Finally, we print the values of all three variables using printf()
statements.
As we can see from the output, the result of the bitwise AND operation on num1
and num2
is 33, which is the binary representation of the value 00100001. This is because only the third and sixth bits of num1
and num2
are both 1, so those are the only bits that are set to 1 in the result. All other bits are set to 0.
2. Bitwise OR:
In C, the bitwise OR operator is represented by the “|” symbol. It is a binary operator that takes two integer operands and performs a bitwise OR operation on them.
The bitwise OR operation compares each bit of the two operands and produces a new integer where each bit in the result is set to 1 if either of the corresponding bits of both operands is 1. Otherwise, the result bit is set to 0.
Example:
#include <stdio.h>
int main() {
int num1 = 35; // Binary: 00100011
int num2 = 45; // Binary: 00101101
int result = num1 | num2;
printf("num1: %d\n", num1);
printf("num2: %d\n", num2);
printf("result: %d\n", result);
return 0;
}
Output:
num1: 35
num2: 45
result: 47
Explanation:
In above example, we declare two integer variables num1
and num2
with the values 35 and 45 respectively. These values are represented in binary to make it easier to see the individual bits.
We then declare a third integer variable result
and set its value to the result of performing a bitwise OR operation on num1
and num2
.
Finally, we print the values of all three variables using printf()
statements.
As we can see from the output, the result of the bitwise OR operation on num1
and num2
is 47, which is the binary representation of the value 00101111. This is because the third and sixth bits of num1
and num2
are both 1, so those bits are set to 1 in the result. All other bits are set to 0 because only one of the corresponding bits in num1
and num2
is 1.
3. Bitwise XOR:
In C, the bitwise XOR operator is represented by the “^” symbol. It is a binary operator that takes two integer operands and performs a bitwise XOR operation on them.
The bitwise XOR operation compares each bit of the two operands and produces a new integer where each bit in the result is set to 1 only if the corresponding bits of the two operands are different. Otherwise, the result bit is set to 0.
Example:
#include <stdio.h>
int main() {
int num1 = 35; // Binary: 00100011
int num2 = 45; // Binary: 00101101
int result = num1 ^ num2;
printf("num1: %d\n", num1);
printf("num2: %d\n", num2);
printf("result: %d\n", result);
return 0;
}
Output:
num1: 35
num2: 45
result: 14
Explanation:
In above example, we declare two integer variables num1
and num2
with the values 35 and 45 respectively. These values are represented in binary to make it easier to see the individual bits.
We then declare a third integer variable result
and set its value to the result of performing a bitwise XOR operation on num1
and num2
.
Finally, we print the values of all three variables using printf()
statements.
As we can see from the output, the result of the bitwise XOR operation on num1
and num2
is 14, which is the binary representation of the value 00001110. This is because only the third and sixth bits of num1
and num2
are different, so those bits are set to 1 in the result. All other bits are set to 0 because the corresponding bits in num1
and num2
are the same.
4. Bitwise NOT:
In C, the bitwise NOT operator is represented by the “~” symbol. It is a unary operator that takes a single integer operand and performs a bitwise NOT operation on it.
The bitwise NOT operation flips each bit of the operand, so that each 1 becomes 0 and each 0 becomes 1. The result is an integer with all the bits flipped.
Example:
#include <stdio.h>
int main() {
int num = 35; // Binary: 00100011
int result = ~num;
printf("num: %d\n", num);
printf("result: %d\n", result);
return 0;
}
Output:
num: 35
result: -36
Explanation:
In above example, we declare an integer variable num
with the value 35, represented in binary to make it easier to see the individual bits.
We then declare a second integer variable result
and set its value to the result of performing a bitwise NOT operation on num
.
Finally, we print the values of both variables using printf()
statements.
As we can see from the output, the result of the bitwise NOT operation on num
is -36, which is the two’s complement representation of the binary value 11011100. This is because all the bits in num
have been flipped, so all the 1s become 0s and all the 0s become 1s.
It is important to note that the result of the bitwise NOT operation is signed, and the sign bit (the leftmost bit) is also flipped. In this case, the sign bit of the original number num
is 0, indicating a positive value. The sign bit of the result is 1, indicating a negative value. This is because the two’s complement representation is used for negative numbers in C.
5. Bitwise Left Shift:
In C, the bitwise left shift operator is represented by the “<<” symbol. It is a binary operator that takes two integer operands: the first operand is the value to be shifted, and the second operand is the number of bits to shift the value by.
The bitwise left shift operation shifts the bits of the first operand to the left by the number of bits specified in the second operand. The bits that are shifted out of the leftmost position are discarded, and new zeros are shifted in on the right side.
Example:
#include <stdio.h>
int main() {
int num = 35; // Binary: 00100011
int shift = 2;
int result = num << shift;
printf("num: %d\n", num);
printf("shift: %d\n", shift);
printf("result: %d\n", result);
return 0;
}
Output:
num: 35
shift: 2
result: 140
Explanation:
In above example, we declare an integer variable num
with the value 35, represented in binary to make it easier to see the individual bits.
We then declare an integer variable shift
with the value 2, which will determine how many bits we want to shift num
to the left.
We declare a third integer variable result
and set its value to the result of performing a bitwise left shift operation on num
by shift
number of bits.
Finally, we print the values of all three variables using printf()
statements.
As we can see from the output, the result of the bitwise left shift operation on num
by 2 bits is 140, which is the binary representation of the value 10001100. This is because we shifted the bits of num
to the left by 2 positions, discarding the leftmost bits and shifting in two new zeros on the right side.
6. Bitwise Right Shift:
In C, the bitwise right shift operator is represented by the “>>” symbol. It is a binary operator that takes two integer operands: the first operand is the value to be shifted, and the second operand is the number of bits to shift the value by.
The bitwise right shift operation shifts the bits of the first operand to the right by the number of bits specified in the second operand. The bits that are shifted out of the rightmost position are discarded, and new zeros are shifted in on the left side.
Example:
#include <stdio.h>
int main() {
int num = 35; // Binary: 00100011
int shift = 2;
int result = num >> shift;
printf("num: %d\n", num);
printf("shift: %d\n", shift);
printf("result: %d\n", result);
return 0;
}
Output:
num: 35
shift: 2
result: 8
Explanation:
In this code, we declare an integer variable num
with the value 35, represented in binary to make it easier to see the individual bits.
We then declare an integer variable shift
with the value 2, which will determine how many bits we want to shift num
to the right.
We declare a third integer variable result
and set its value to the result of performing a bitwise right shift operation on num
by shift
number of bits.
Finally, we print the values of all three variables using printf()
statements.
As we can see from the output, the result of the bitwise right shift operation on num
by 2 bits is 8, which is the binary representation of the value 00001000. This is because we shifted the bits of num
to the right by 2 positions, discarding the rightmost bits and shifting in two new zeros on the left side.