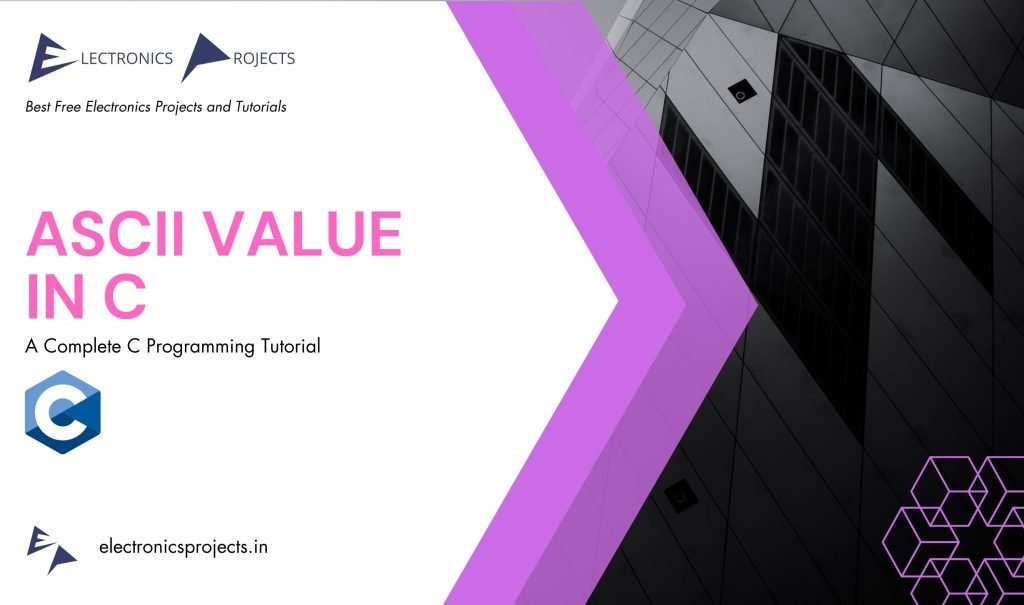
What is ASCII value in C?
ASCII stands for American Standard Code for Information Interchange, and it is a standard code used to represent characters in computers. In C, ASCII values are represented as integers, where each integer represents a specific character.
Each character in the ASCII table has a unique integer value assigned to it, ranging from 0 to 127. For example, the ASCII value for the uppercase letter ‘A’ is 65, and the ASCII value for the lowercase letter ‘a’ is 97.
Example:
#include <stdio.h>
int main() {
char c = 'A';
printf("The ASCII value of %c is %d\n", c, c);
return 0;
}
Output:
The ASCII value of A is 65
Explanation:
In this example, we declare a variable c
of type char
and initialize it with the uppercase letter ‘A’. Then, we use the printf()
function to print the ASCII value of c
using the %d
format specifier.
Example 2:
Here is a more advanced example that demonstrates the use of ASCII values to create a simple encryption program:
#include <stdio.h>
int main() {
char message[] = "Hello, World!";
int key = 10;
int i;
for(i = 0; message[i] != '\0'; ++i) {
message[i] = message[i] + key;
}
printf("Encrypted message: %s\n", message);
for(i = 0; message[i] != '\0'; ++i) {
message[i] = message[i] - key;
}
printf("Decrypted message: %s\n", message);
return 0;
}
Output:
Encrypted message: Rovvy6*ay|vn+
Decrypted message: Hello, World!
Explanation:
In this example, we declare a character array message
that contains the string “Hello, World!” and an integer key
that represents the number of positions to shift each character by. We then use a for
loop to iterate over each character in the message
array, add the key
to its ASCII value, and store the new character back in the array. This effectively encrypts the message by shifting each character by key
positions.
We then use another for
loop to iterate over the encrypted message
array, subtract the key
from each character’s ASCII value, and store the decrypted character back in the array. Finally, we print the decrypted message to confirm that the encryption and decryption process was successful.
Rules to follow while using ASCII values in C:
- ASCII values are represented as integers, not characters.
- Each character in the ASCII table has a unique integer value assigned to it.
- ASCII values range from 0 to 127.
- To use ASCII values, you can simply assign them to a variable of type
int
orchar
. - You can use ASCII values to represent special characters and symbols.
- When printing ASCII values, use the
%d
format specifier. When printing characters, use the%c
format specifier. - When performing calculations with ASCII values, be mindful of overflow and underflow.