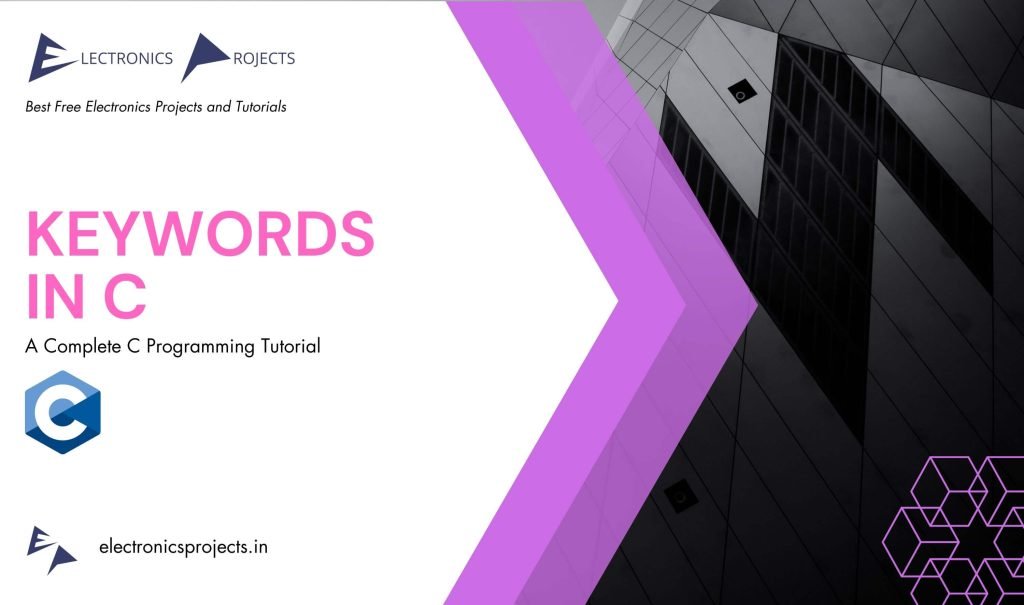
What is Keywords in C?
Keywords in C are predefined reserved words that have a specific meaning in the C programming language. These words cannot be used as variable names, function names, or any other identifiers. They are used to define the syntax and structure of the C language and play a crucial role in its compilation and interpretation.
Keywords are used to define various elements of the C language, such as data types, control structures, loops, functions, and storage classes. They provide a standard set of instructions that the compiler can interpret and execute efficiently.
There are a total of 32 keywords in C, and they can be divided into the following categories:
- Data types: These keywords are used to define the basic data types in C, such as int, char, float, double, and void.
- Control structures: These keywords are used to control the flow of the program, such as if, else, switch, case, and default.
- Loops: These keywords are used to implement iteration, such as for, while, and do.
- Functions: These keywords are used to define functions, such as return, break, and continue.
- Storage classes: These keywords are used to define the storage class of variables, such as auto, register, static, and extern.
Types of Keywords in C:
SR. NO. | Keyword | Information |
---|---|---|
1 | auto | Used to declare local variables with automatic storage duration. |
2 | break | Used to exit from a loop or switch statement. |
3 | case | Used to define a case in a switch statement. |
4 | char | Used to define a character data type. |
5 | const | Used to define a constant value. |
6 | continue | Used to skip the current iteration of a loop. |
7 | default | Used to define a default case in a switch statement. |
8 | do | Used to define a do-while loop. |
9 | double | Used to define a double-precision floating-point data type. |
10 | else | Used to define an alternative branch in an if statement. |
11 | enum | Used to define an enumerated data type. |
12 | extern | Used to declare a variable or function that is defined in another file. |
13 | float | Used to define a single-precision floating-point data type. |
14 | for | Used to define a for loop. |
15 | goto | Used to jump to a labeled statement. |
16 | if | Used to define a conditional statement. |
17 | int | Used to define an integer data type. |
18 | long | Used to define a long integer data type. |
19 | register | Used to declare local variables with register storage duration. |
20 | return | Used to return a value from a function. |
21 | short | Used to define a short integer data type. |
22 | signed | Used to define a signed data type. |
23 | sizeof | Used to determine the size of a data type or variable. |
24 | static | Used to declare a variable or function with static storage duration. |
25 | struct | Used to define a structure data type. |
26 | switch | Used to define a switch statement. |
27 | typedef | Used to define a new data type. |
28 | union | Used to define a union data type. |
29 | unsigned | Used to define an unsigned data type. |
30 | void | Used to define a void data type. |
31 | volatile | Used to declare a variable that can be modified by hardware or other software. |
32 | while | Used to define a while loop. |
Rules while declaring Keywords in C:
- Keywords cannot be used as variable names, function names, or any other identifiers.
- Keywords are case sensitive and must be spelled correctly.
- Keywords cannot be abbreviated or modified in any way.