Description:
In this project we build a Snake Game using Arduino and 8×8 Dot Matrix Display. This is just a classic snake game which we all used to play back in the day. Here we have used an 8×8 Dot Matrix Display. You can use buttons to move the snake and score can be viewed on 16×2 LCD Display. Arduino UNO is used.
Circuit Diagram:
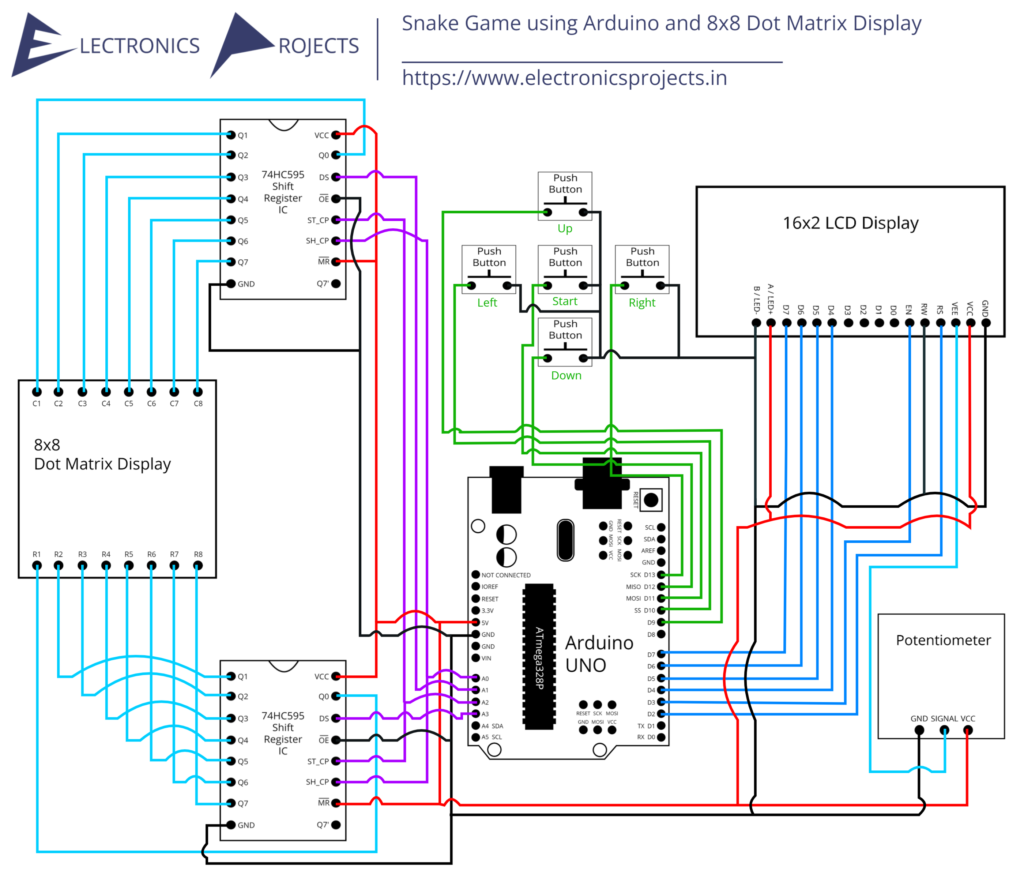
Components:
SR. NO. | COMPONENT | PINOUT DIAGRAM | BUY |
---|---|---|---|
1 | Arduino UNO (Other Arduinos can be used too) | Arduino UNO Pinout Diagram⇗ | |
2 | 8×8 Dot Matrix Display | ||
3 | 74HC595 Shift Register IC | ||
4 | 16×2 LCD Display | ||
5 | Push Buttons x 5 | ||
6 | 10K Potentiometer |
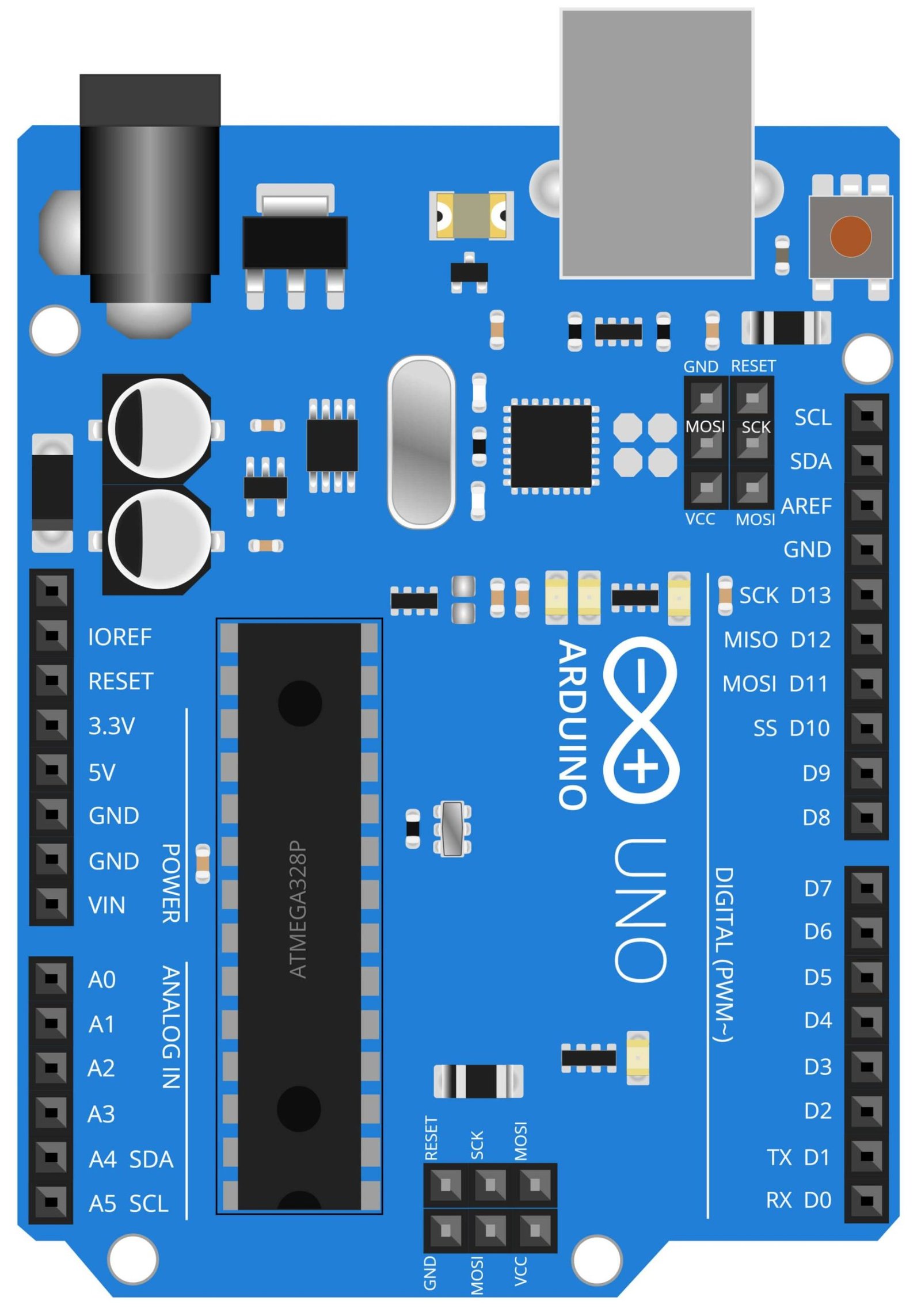
Arduino UNO
The Arduino UNO is a microcontroller board based on the ATmega328P. It has 14 digital input/output pins, 6 analog inputs, a 16 MHz quartz crystal, a USB connection, a power jack, an ICSP header, and a reset button. The board is powered by a 9V battery or by the USB cable. It is open-source hardware, which means that users are able to modify and distribute the device.
In this project, the Arduino UNO serves as the brain of the device, it receives input from the push buttons and process the data to control the 8×8 Dot Matrix Display, which is used to display the snake game, and the 16×2 LCD display, which is used to display the score and other information related to the game. The Arduino UNO is programmed using the Arduino IDE, which makes it easy to write, upload and debug the code.
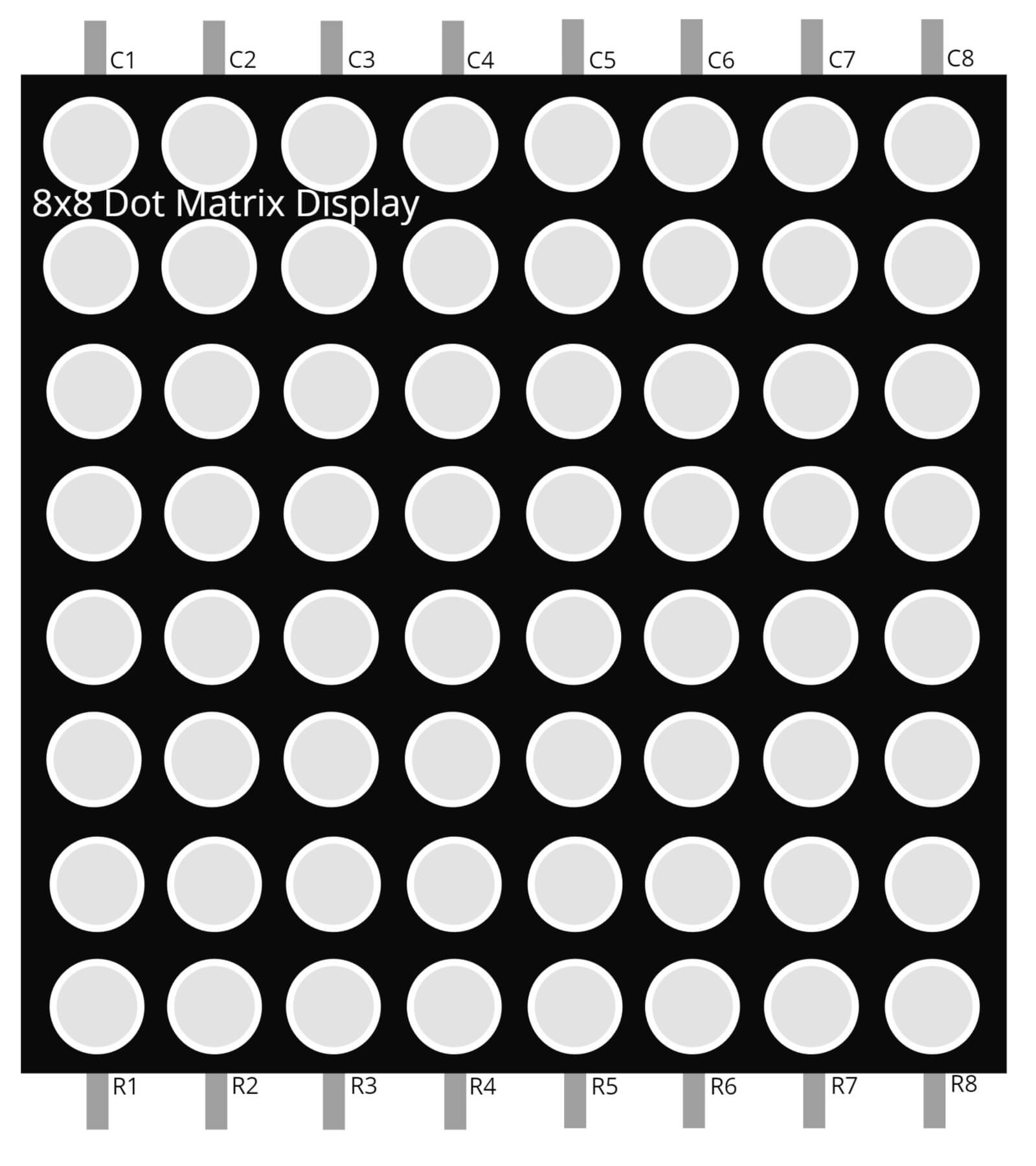
8×8 Dot Matrix Display
The 8×8 Dot Matrix Display is a type of LED matrix that consists of 64 individual LEDs arranged in an 8×8 grid. These LEDs can be controlled individually, allowing for the creation of custom patterns and characters. The 8×8 Dot Matrix Display is commonly used in projects such as LED displays, games and other applications where a compact and efficient display is required.
In this project, the 8×8 Dot Matrix Display serves as the primary display for the snake game. The game is displayed on the matrix using a pattern of lit and unlit LEDs. The Arduino UNO receives input from the push buttons and process the data to control the 8×8 Dot Matrix Display. The 8×8 Dot Matrix Display is controlled by a 74HC595 Shift Register IC and the input from push buttons is used to control the snake movements.
The 8×8 Dot Matrix Display is a low-cost, easy to use and reliable display that can be used in a wide range of applications. It is small, compact and easy to connect to a microcontroller. It can be controlled by a microcontroller or shift register IC and can be used to create various patterns and characters, making it a versatile display option for various projects.
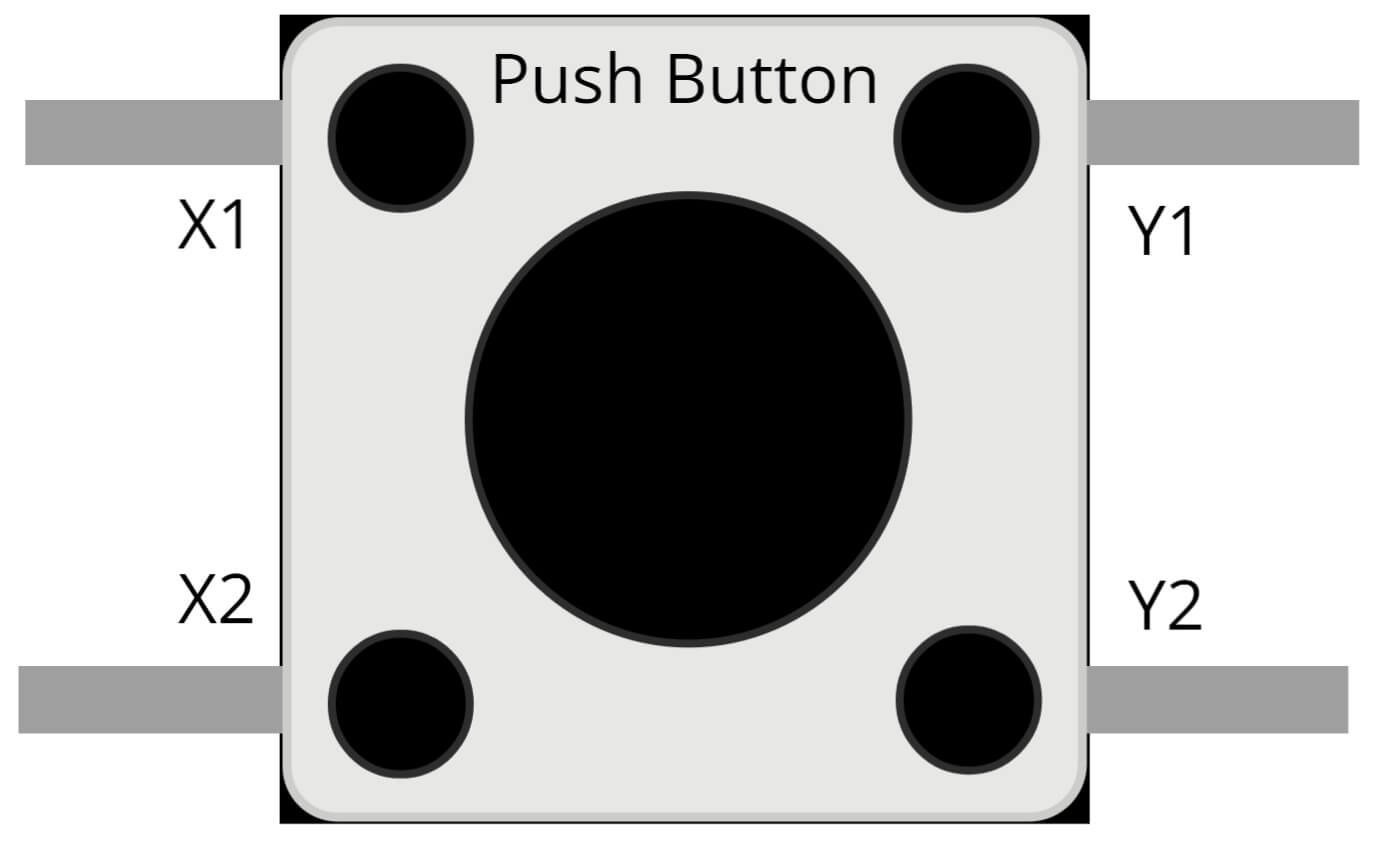
Push Button
Push buttons are electronic switches that are commonly used in various electronic projects, including games, control systems, and interactive displays. They are available in a variety of forms, including mechanical, membrane and capacitive types. Push buttons can be connected to a microcontroller or other electronic device to detect when they have been pressed or released.
In this project, the push buttons are used to control the snake movements in the game. The push buttons are connected to the Arduino UNO, which receives input from the push buttons and process the data to control the snake movements on the 8×8 Dot Matrix Display. The push buttons are used to change the direction of the snake. The Arduino UNO is programmed to recognize the input from the push buttons and make the corresponding changes to the snake movements on the 8×8 Dot Matrix Display
Steps and Info:
1. Get correct components as given. You can buy online or offline. I buy electronics components mostly from Amazon.
2. Start connecting the components. If you are new to connecting components in an electronic circuit, then use a Breadboard or you can straight up make connections using Jumper wires but it will create problem when there will be two or more connections needed at one pin (for example two or more different sensors 5V connection to Arduino’s 5V pin). But before soldering components on a PCB or printing a PCB for your circuit, better try using breadboard so that any errors and mistakes can be observed on breadboard and not after soldering. Or do connections in your way.
3. Now after building the circuit, download the Arduino IDE from https://www.arduino.cc/ website.
4. If you are new to Arduino IDE software, then watch this video we made specially for beginners about Arduino IDE.
5. If you know Arduino IDE then straight up copy the code we given and paste it into the Arduino IDE sketch.
6. Connect the Arduino to your Computer/device. Select a proper port, proper Arduino type and whatever other settings are. Here it is Arduino UNO. (If you don’t know what this all is then watch our video: ).
7. Compile the code and Upload it.
8. If any error occurs then try to troubleshoot it by finding/copy-pasting it into our Solve Errors page https://electronicsprojects.in/solve-errors/, or you can straight up paste the error on Internet and you know the rest. Also check if there are no spelling/syntax errors in the code. Compile the code again once to check if errors are fixed. I have given proper connections and code but still nothing is perfect.
9. Once code compiles and uploads smoothly, you can start testing the working of your circuit/project.
10. Arrange the Buttons in correct positions as per the moment of the snake. Start playing it.
11. If it doesn’t work, then check connections and code. Also you can talk with me and the community through discord. All the links to social is given at the right side of this page.
Program Code:
/* https://www.electronicsprojects.in Snake Game using Arduino and 8x8 Dot Matrix Display */
#include<LiquidCrystal.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
#define ds_col 15
#define sh_col 16
#define st_col 14
#define ds_row 17
#define start 11
#define up 9
#define down 12
#define left 10
#define right 13
char Col[21],Row[21],move_c,move_r;
int colum_data(int temp)
{
switch(temp)
{
case 1: return 1;break;
case 2: return 2; break;
case 3: return 4; break;
case 4: return 8; break;
case 5: return 16; break;
case 6: return 32; break;
case 7: return 64; break;
case 8: return 128; break;
default: return 0; break;
}
}
int row_data(int temp)
{
switch(temp)
{
case 1: return 1;break;
case 2: return 2; break;
case 3: return 4; break;
case 4: return 8; break;
case 5: return 16; break;
case 6: return 32; break;
case 7: return 64; break;
case 8: return 128; break;
default: return 0; break;
}
}
void read_button()
{
if(!digitalRead(left))
{
move_r=0;
move_c!=-1 ? move_c=-1 : move_c=1;
while(!digitalRead(left));
}
if(!digitalRead(right))
{
move_r=0;
move_c!=1 ? move_c=1 : move_c=-1;
while(!digitalRead(right));
}
if(!digitalRead(up))
{
move_c=0;
move_r!=-1 ? move_r=-1 : move_r=1;
while(!digitalRead(up));
}
if(!digitalRead(down))
{
move_c=0;
move_r!=1 ? move_r=1 : move_r=-1;
while(!digitalRead(down));
}
}
void show_snake(int temp)
{
for(int n=0;n<temp;n++)
{
int r,c;
for(int k=0;k<21;k++)
{
int temp1=Col[k];
c=colum_data(temp1);
int temp2=Row[k];
r=0xff-row_data(temp2);
for(int i=0;i<8;i++)
{
int ds=(c & 0x01);
digitalWrite(ds_col, ds);
ds=(r & 0x01);
digitalWrite(ds_row, ds);
digitalWrite(sh_col, HIGH);
c>>=1;
r>>=1;
digitalWrite(sh_col, LOW);
}
digitalWrite(st_col, HIGH);
digitalWrite(st_col, LOW);
read_button();
delayMicroseconds(500);
}
}
}
void setup()
{
lcd.begin(16,2);
pinMode(ds_col, OUTPUT);
pinMode(sh_col, OUTPUT);
pinMode(st_col, OUTPUT);
pinMode(ds_row, OUTPUT);
pinMode(start, INPUT);
pinMode(up, INPUT);
pinMode(down, INPUT);
pinMode(left, INPUT);
pinMode(right, INPUT);
digitalWrite(up, HIGH);
digitalWrite(down, HIGH);
digitalWrite(left, HIGH);
digitalWrite(right, HIGH);
digitalWrite(start, HIGH);
lcd.setCursor(0,0);
lcd.print(" Snake game ");
lcd.setCursor(0,1);
lcd.print("Circuit Digest ");
delay(2000);
lcd.setCursor(0,0);
lcd.print(" Press Start ");
lcd.setCursor(0,1);
lcd.print(" To Play ");
delay(2000);
}
void loop()
{
int j,k,Speed=40,score=0;
j=k=move_c=0;
move_r=1;
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Score: ");
lcd.print(score);
while(1)
{
for(int i=3;i<21;i++)
{
Row[i]=100;
Col[i]=100;
}
Row[0]=rand()%8+1;
Col[0]=rand()%8+1;
Row[1]=1;
Col[1]=1;
Row[2]=2;
Col[2]=1;
j=2,k=1;
while(k==1)
{
move_c=0;
move_r=1;
show_snake(1);
lcd.setCursor(7,0);
lcd.print(score);
if(!digitalRead(start))
{
k=2;
Speed=40;
score=0;
}
}
while(k==2)
{
show_snake(Speed);
if(Row[1]>8 || Col[1]>8 || Row[1]<0 || Col[1]<0)
{
Row[1]=1;
Col[1]=1;
k=1;
lcd.setCursor(0,1);
lcd.print("Game Over");
delay(5000);
score=0;
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Score: ");
lcd.print(score);
}
if(Row[0]==Row[1]+move_r && Col[0]==Col[1]+move_c)
{
j++;
Speed-=2;
score=score+5;
lcd.setCursor(7,0);
lcd.print(score);
Row[0]=rand()%8+1;
Col[0]=rand()%8+1;
}
for(int i=j;i>1;i--)
{
Col[i]=Col[i-1];
Row[i]=Row[i-1];
}
Col[1]=Col[2]+move_c;
Row[1]=Row[2]+move_r;
}
}
}